Overcoming Duplicate Values in EnumSet Collections
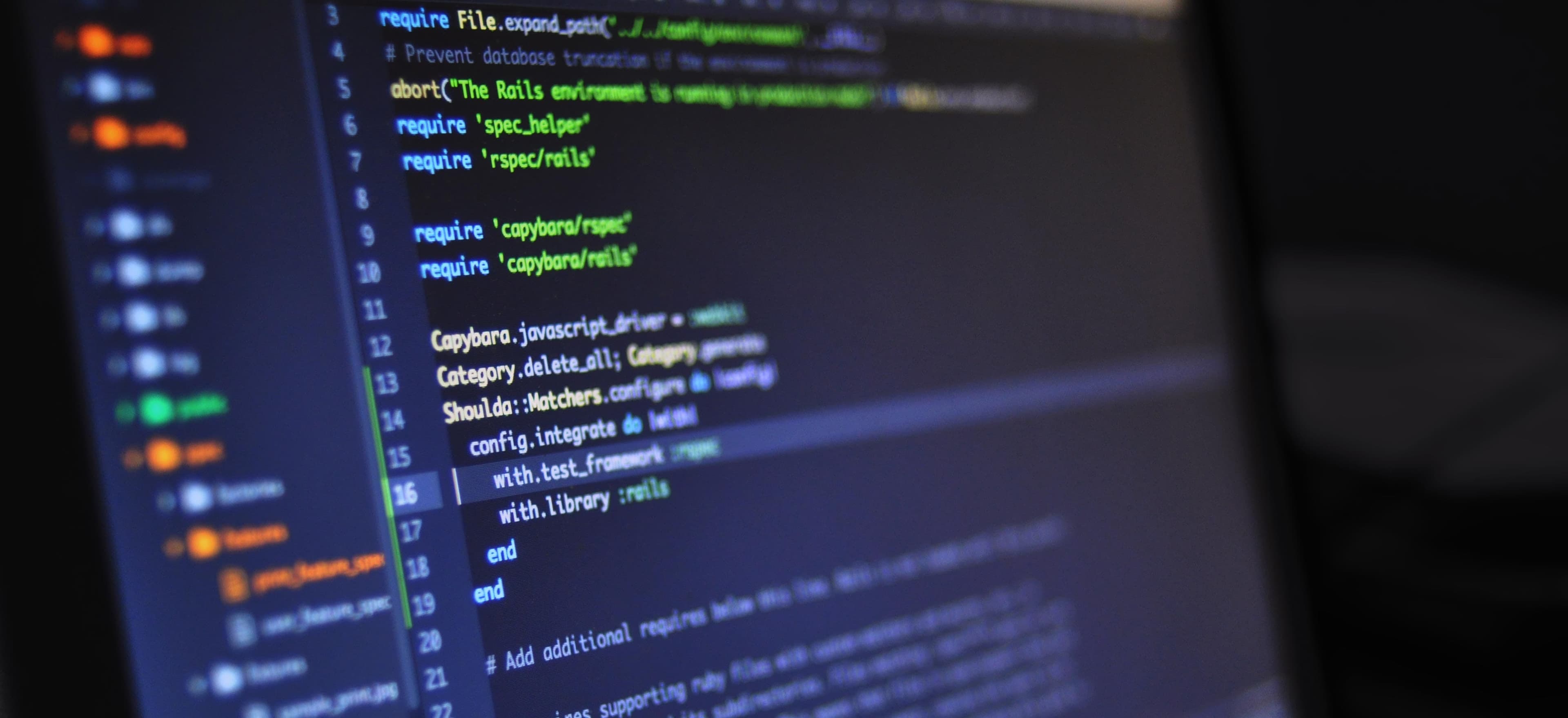
- Published on
Overcoming Duplicate Values in EnumSet Collections
When working with collections in Java, it's important to understand the different types of collections available and how to effectively use them. One specific type of collection, EnumSet, provides an efficient way to represent a set of values from a single enum type. However, EnumSet has a unique feature that can catch developers off guard - it does not allow duplicate values. This can lead to unexpected behavior if not handled correctly.
In this article, we'll delve into the use of EnumSet collections, explore the issue of duplicate values, and provide solutions to overcome this challenge.
Understanding EnumSet
EnumSet is a specialized Set implementation designed for use with enum types. It is implemented as a compact bit vector, making it highly efficient for representing sets of enum constants. EnumSet is part of the java.util
package and was introduced in Java 5.
The main benefit of using EnumSet is its performance. It is optimized for enum types, providing a very efficient representation of sets with a small memory footprint and fast execution time for common operations such as adding, removing, and testing for the presence of elements.
Let's consider an example of an enum type representing different colors:
public enum Color {
RED, GREEN, BLUE, YELLOW
}
We can create an EnumSet to hold these colors:
EnumSet<Color> primaryColors = EnumSet.of(Color.RED, Color.GREEN, Color.BLUE);
The Issue with Duplicate Values
One of the key characteristics of EnumSet is its restriction on allowing duplicate values. If a duplicate value is added, it will not be included in the set, and no exception is thrown. This behavior is rooted in the design of EnumSet, as enum types by definition do not allow duplicate constants.
Consider the following code:
EnumSet<Color> colors = EnumSet.of(Color.RED, Color.GREEN, Color.RED);
System.out.println(colors.contains(Color.RED)); // Output: true
System.out.println(colors.size()); // Output: 2
In this case, although we attempted to include Color.RED
twice in the set, it only appears once, as EnumSet's underlying implementation ensures uniqueness.
Handling Duplicate Values
When encountering a situation where you need to work with a set of enum values that could potentially have duplicates, there are several approaches to tackle this issue.
1. Using a Different Collection Type
If your use case necessitates the presence of duplicate values in the collection, it might be more appropriate to use a different type of collection, such as a List
or a HashSet
, with proper equals/hashCode implementation. These collections allow duplicate values and provide different semantics for handling elements.
List<Color> colorList = new ArrayList<>();
colorList.add(Color.RED);
colorList.add(Color.GREEN);
colorList.add(Color.RED);
System.out.println(colorList.size()); // Output: 3
2. Using a Wrapper Class
Another approach is to use a wrapper class to store the enum values alongside their frequency. This wrapper can maintain the counts of each enum value and provide methods to increment, decrement, or retrieve the frequency of a specific value.
public class EnumFrequencyWrapper<E extends Enum<E>> {
private final Map<E, Integer> frequencyMap = new HashMap<>();
public void increment(E value) {
frequencyMap.put(value, frequencyMap.getOrDefault(value, 0) + 1);
}
public void decrement(E value) {
int count = frequencyMap.getOrDefault(value, 0);
if (count == 1) {
frequencyMap.remove(value);
} else {
frequencyMap.put(value, count - 1);
}
}
public int getFrequency(E value) {
return frequencyMap.getOrDefault(value, 0);
}
}
By using this wrapper class, you can keep track of the frequency of each enum value while also ensuring that all values are accounted for, including duplicates.
3. Custom Handling and Validation
In some cases, it may be beneficial to customarily handle duplicate values by designing explicit validation or deduplication processes in your code. For instance, you can create a method that checks for duplicates before adding a value to the EnumSet, or implement a mechanism to merge the sets and handle duplicate values according to your application's requirements.
EnumSet<Color> combinedColors = EnumSet.copyOf(primaryColors);
combinedColors.addAll(EnumSet.of(Color.RED, Color.GREEN, Color.RED));
System.out.println(combinedColors); // Output: [RED, GREEN, BLUE]
Key Takeaways
In this article, we delved into the efficiency and uniqueness characteristics of EnumSet collections in Java. While EnumSet is a powerful tool for representing sets of enum constants, its restriction on duplicate values can pose challenges in certain scenarios. We explored various strategies to handle duplicate values, including leveraging different collection types, using wrapper classes, and implementing custom validation and handling.
Understanding the behavior and limitations of EnumSet is crucial for making informed decisions when selecting the right collection type for your specific use cases. By considering the approaches outlined in this article, you can effectively overcome the limitations of duplicate values in EnumSet collections and design robust solutions that align with your application's requirements.
For further exploration on EnumSet and related topics, refer to the official Java documentation and additional resources such as the Java Collections Framework and Effective Java.