Maximize Efficiency: Tackle Memory Consumption Issues Today
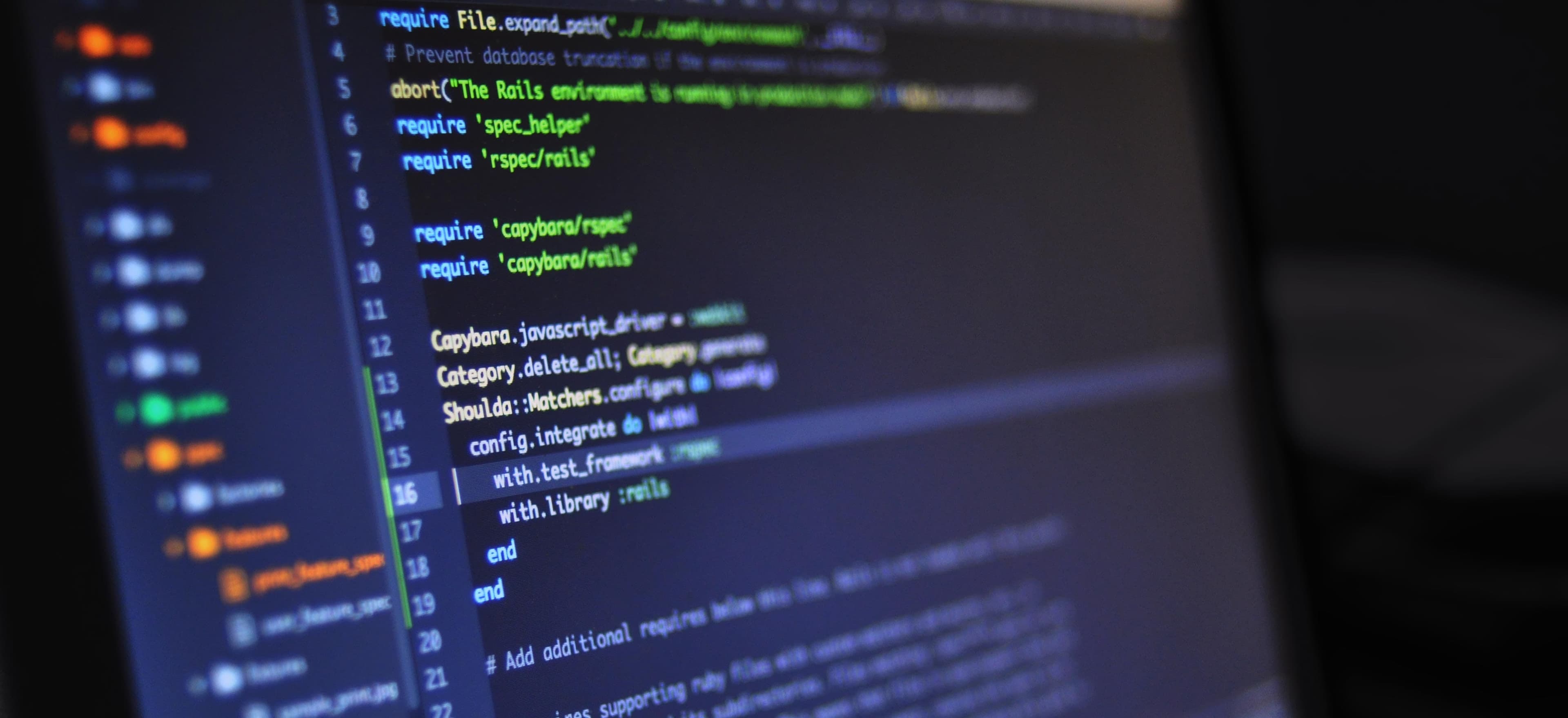
- Published on
Maximize Efficiency: Tackle Memory Consumption Issues Today
In today's technology-driven world, applications are getting increasingly complex. Memory management has thus become a critical concern for developers. In this blog post, we will explore how to analyze and optimize memory consumption issues in Java applications. We will discuss common pitfalls, tools, and techniques, and provide actionable code snippets to help you tackle these challenges effectively.
Understanding Memory Consumption in Java
Java is known for its automatic memory management through Garbage Collection (GC). However, this doesn’t mean that memory issues are non-existent. It is crucial to understand how memory is allocated and managed in Java to identify problems early.
The Java Memory Model
The Java memory model consists of several components:
- Heap Memory: Where Java objects reside.
- Stack Memory: Stores local variables and method call information.
- Metaspace: For storing class metadata.
Understanding these components will help you navigate through memory management effectively.
Common Memory Consumption Issues
Before diving into solutions, let's identify some common memory consumption issues:
1. Memory Leaks
A memory leak occurs when an object is no longer needed but is still being referenced, preventing garbage collection.
2. Excessive Object Creation
Creating too many objects can lead to high memory consumption. This is particularly important for applications with high demand, such as web servers.
3. Inefficient Data Structures
Using the wrong data structures can consume more memory than necessary.
Tools to Analyze Memory Consumption
To tackle these issues, it is important to analyze memory consumption using the right tools:
-
Java VisualVM: A tool for monitoring Java application performance, memory usage, and garbage collection.
-
Eclipse Memory Analyzer (MAT): Analyzes memory dumps to help identify memory leaks and reduce memory consumption.
-
JProfiler: A commercial tool that offers a comprehensive suite of features for performance analysis.
Code Snippet: Analyzing Memory Usage
Here’s how to analyze memory usage in a Java application using Java VisualVM
:
-
Run your application with the following JVM argument:
-Dcom.sun.management.jmxremote
-
Open Java VisualVM and attach it to your running application.
-
Use the “Memory” tab to monitor heap memory usage over time.
Code Example
The following example demonstrates how memory usage can be monitored programmatically.
import java.lang.management.ManagementFactory;
import java.lang.management.MemoryMXBean;
import java.lang.management.MemoryUsage;
public class MemoryMonitoring {
public static void main(String[] args) {
MemoryMXBean memoryMXBean = ManagementFactory.getMemoryMXBean();
MemoryUsage heapUsage = memoryMXBean.getHeapMemoryUsage();
System.out.printf("Heap Memory Usage: %s%n", heapUsage);
// Additional logging to monitor memory usage can be added here.
}
}
This program outputs the current heap memory usage, allowing you to track your memory consumption.
Techniques for Reducing Memory Consumption
Once you've identified memory consumption issues, it’s time to implement solutions.
1. Avoid Memory Leaks
To avoid memory leaks, ensure that you nullify references when they are no longer needed.
List<Object> list = new ArrayList<>();
// add elements to list
list = null; // Help GC by nullifying the reference when done.
2. Optimize Object Creation
Use object pools where applicable. Reusing objects instead of creating new ones can drastically lower memory consumption.
public class ObjectPool {
private List<HeavyObject> pool = new ArrayList<>();
public HeavyObject acquire() {
if (pool.isEmpty()) {
return new HeavyObject(); // Create new if pool is empty.
}
return pool.remove(pool.size() - 1); // Reuse an object.
}
public void release(HeavyObject obj) {
pool.add(obj); // Returns object back to the pool.
}
}
Using an object pool minimizes the creation and destruction of objects frequently, preventing memory fragmentation.
3. Choose the Right Data Structures
Always select data structures according to your application's needs. For instance, in situations where lookups are more frequent than addition/removal, consider using a HashMap
.
// Consider using a HashMap for quicker lookups
Map<String, Integer> map = new HashMap<>();
map.put("John", 25); // Fast lookups
4. Lazy Initialization
Use lazy initialization for objects that are not always required.
public class LazyLoader {
private HeavyObject heavyObject;
public HeavyObject getHeavyObject() {
if (heavyObject == null) {
heavyObject = new HeavyObject(); // Instantiate only when needed
}
return heavyObject;
}
}
This approach defers expensive initialization until it is absolutely necessary.
Monitoring Garbage Collection
To maximize efficiency, it's also important to monitor garbage collection (GC). Here’s how you can set up your Java application to log GC details:
-
Add the following JVM options when running your application:
-Xloggc:gc.log -XX:+PrintGCDetails -XX:+PrintGCTimeStamps
-
Analyze
gc.log
using tools likeGCViewer
orGCeasy
to understand your application's GC behavior.
Final Thoughts
Addressing memory consumption in Java is not just about identifying problems but also about implementing effective strategies to tackle them. By understanding memory usage, utilizing the right tools for analysis, and adopting best practices, you can greatly enhance your application's performance.
Key Takeaways:
- Use tools like Java VisualVM and Eclipse MAT for monitoring and analyzing memory.
- Avoid memory leaks and excessive object creation by employing object pools and lazy initialization.
- Always choose the correct data structures suited to your application's needs.
By maximizing your application's efficiency now, you pave the way for a more responsive and robust software experience in the future. For further reading, consider visiting Oracle’s Java Memory Management for more in-depth guidance.
Feel free to ask any questions or share your comments below! Your input might help someone else tackle their memory consumption issues more effectively.
Checkout our other articles