Spring Bean Aliasing: Solving Conflicts in Large Projects
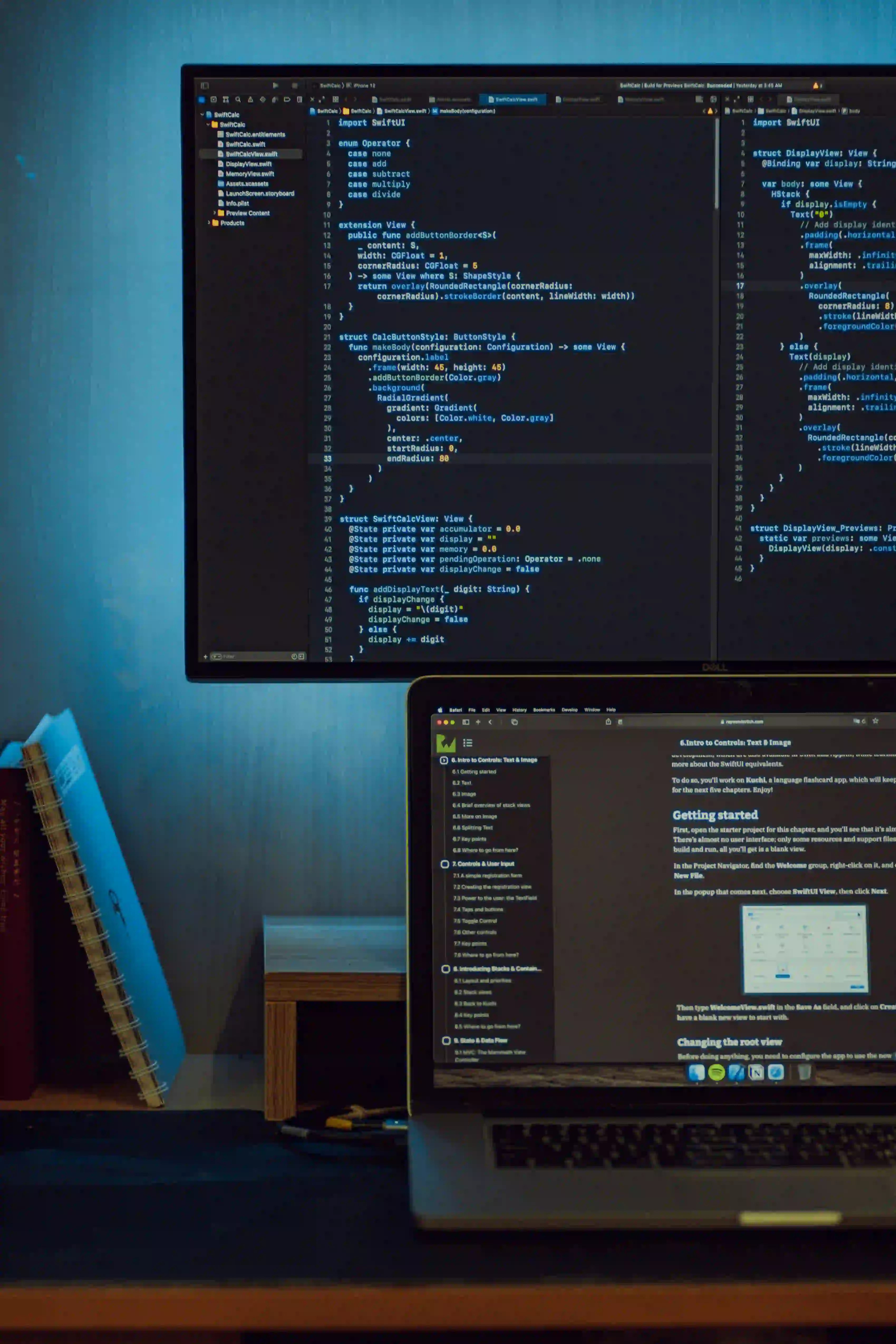
Solving Bean Naming Conflicts in Spring: The Power of Bean Aliasing
When developing large-scale Java projects with the Spring framework, you might encounter situations where multiple beans implement the same interface or extend the same class. This can lead to naming conflicts and ambiguity, making it challenging to wire the beans correctly. In such scenarios, Spring provides a powerful mechanism called "bean aliasing" to disambiguate bean names and resolve conflicts.
In this blog post, we will explore the concept of bean aliasing in Spring, understand why it's essential in large projects, and learn how to effectively use it to untangle bean wiring complexities.
What is Bean Aliasing?
Bean aliasing in Spring is a technique that allows you to define multiple names for the same bean definition. With bean aliasing, you can register a bean with one or more unique names, and then refer to the bean using any of its aliases.
Consider a situation where you have two classes, CustomerServiceImpl
and DefaultCustomerService
, both implementing the CustomerService
interface. In such cases, when defining beans in the Spring context, conflicts may arise due to the identical interface implementation.
This is where bean aliasing comes to the rescue. By creating aliases for these beans, you can differentiate them within the Spring context and avoid naming clashes.
Why Use Bean Aliasing?
1. Resolving Name Conflicts:
In large projects with a multitude of components, naming clashes are a common occurrence. Bean aliasing provides a clean and straightforward approach to address such conflicts, ensuring that each bean can be uniquely identified within the application context.
2. Clarity and Maintenance:
By using well-defined aliases, the codebase becomes more readable and maintainable. It becomes easier for developers to understand the purpose of each bean and its associations, leading to improved code comprehension and reduced chances of errors.
3. Flexibility in Integration:
When integrating third-party libraries or framework components that might have conflicting bean names, aliasing allows you to seamlessly incorporate these components into your application context without altering their original definitions.
How to Define Bean Aliases in Spring?
Defining bean aliases in Spring is a straightforward process. Let's consider the following example, where we have multiple implementations of the CustomerService
interface that need to be wired.
Example Bean Definitions with Aliases
@Configuration
public class AppConfig {
@Bean(name = "customerServicePrimary")
public CustomerService customerServicePrimary() {
return new CustomerServiceImpl();
}
@Bean
public CustomerService customerServiceSecondary() {
return new DefaultCustomerService();
}
@Bean
public CustomerService customerServiceAlias() {
return new DefaultCustomerService();
}
}
In this example, we have defined three beans implementing the CustomerService
interface. The first bean, customerServicePrimary
, is directly named. The second bean, customerServiceSecondary
, uses its method name as the default bean name. Additionally, an alias customerServiceAlias
has been created for the third bean to provide an alternate reference to the DefaultCustomerService
implementation.
By using different bean names and their aliases, the potential conflicts between the beans are effectively mitigated, and the ambiguous references are resolved.
Referencing Bean Aliases in Dependency Injection
Now that we have defined bean aliases, let's explore how we can refer to these beans in other components, such as when performing dependency injection.
Dependency Injection Using Bean Aliases
@Service
public class CustomerServiceUser {
@Autowired
@Qualifier("customerServicePrimary")
private CustomerService primaryCustomerService;
@Autowired
@Qualifier("customerServiceAlias")
private CustomerService aliasedCustomerService;
// ...
}
In the CustomerServiceUser
class, we use the @Autowired
annotation along with @Qualifier
to specify which bean instance should be injected. Here, we explicitly refer to the bean aliases customerServicePrimary
and customerServiceAlias
to resolve the ambiguity and wire the respective beans to the CustomerServiceUser
class.
By employing aliases in the annotation-based dependency injection, we ensure that the correct beans are injected based on their distinct names, thus avoiding any conflicts or uncertainties.
Bringing It All Together
In large-scale Spring projects, efficiently managing bean definitions and their associations is crucial for maintaining a robust and comprehensible codebase. Bean aliasing in Spring provides a pragmatic solution to mitigate naming conflicts, enhance code clarity, and facilitate seamless integration of components.
By leveraging bean aliases, developers can streamline the wiring of beans, improve the readability of the application context, and prevent potential clashes in naming conventions. This, in turn, leads to a more maintainable and adaptable codebase, ensuring a smooth development experience for all team members involved.
In conclusion, mastering the art of bean aliasing in Spring empowers developers to conquer the challenges of bean naming conflicts, fostering a harmonious and well-structured application architecture.
Now that you have a solid understanding of bean aliasing in Spring, take the opportunity to apply this knowledge in your own projects and witness the remarkable impact it can have on simplifying bean wiring complexities. Happy coding!
If you're keen on further enriching your understanding of Spring and Java, check out these resources:
Feel free to share your experiences and insights on leveraging bean aliasing in Spring projects in the comments section below!