Master Non-Recursive Binary Search in Java Today!
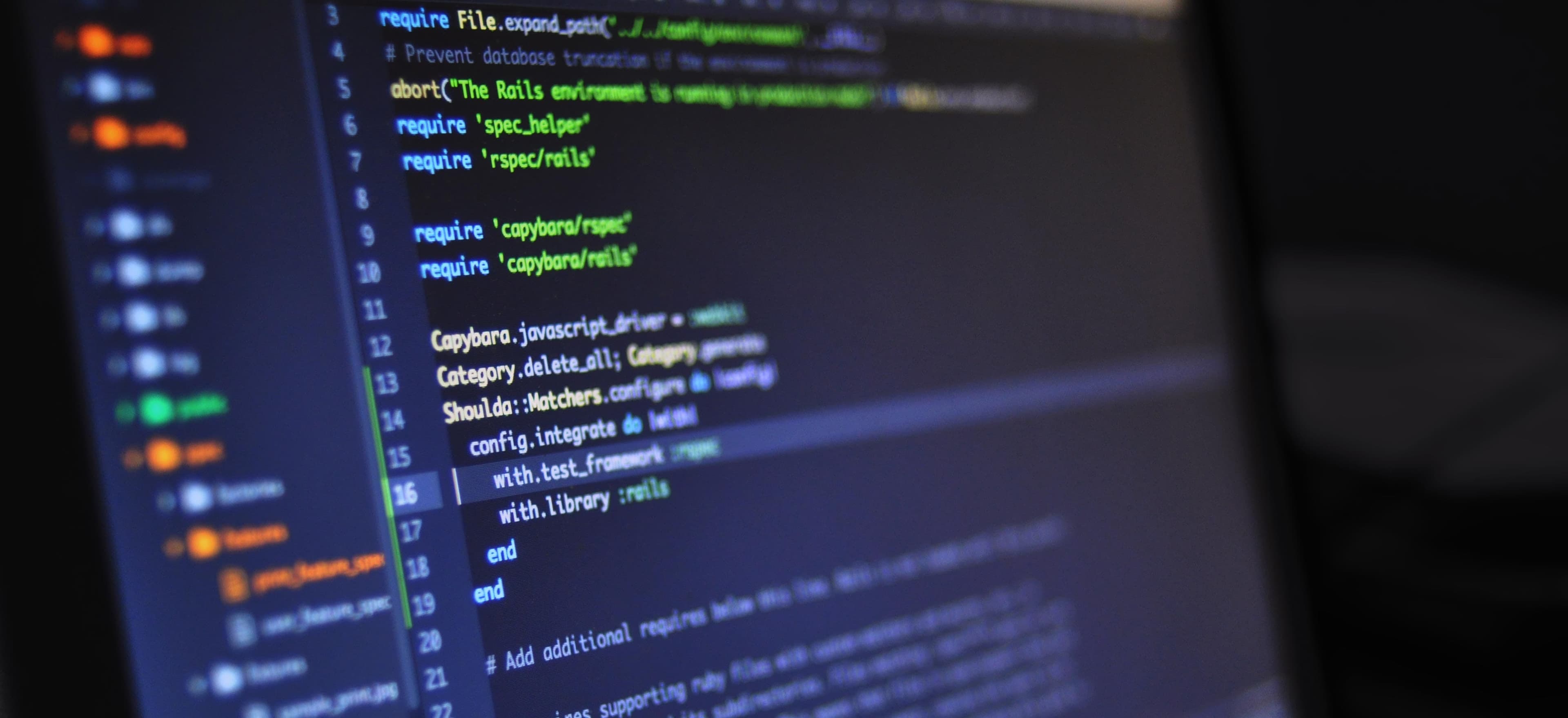
- Published on
Master Non-Recursive Binary Search in Java
Binary search is a fundamental algorithm in computer science used to efficiently locate a specific item in a sorted collection of items. In this blog post, we will delve into non-recursive binary search in Java, exploring its implementation, advantages, and use cases. By the end of this post, you will have a comprehensive understanding of how to leverage non-recursive binary search to optimize your Java programs.
Understanding Binary Search
Binary search operates by repeatedly dividing in half the portion of the array that could contain the item, until you've narrowed down the possible locations to just one. It's a logarithmic algorithm, making it significantly faster than linear search for large datasets.
How Non-Recursive Binary Search Works
Non-recursive binary search operates using a while loop to iteratively narrow down the search space until the desired item is found or determined to be absent. By utilizing this approach, we can achieve the same result as the recursive implementation, without incurring the overhead of function calls and maintaining a stack.
Let's delve into the non-recursive binary search implementation in Java.
public static int binarySearch(int[] arr, int key) {
int low = 0;
int high = arr.length - 1;
while (low <= high) {
int mid = (low + high) / 2;
if (arr[mid] == key) {
return mid; // Found the key
} else if (arr[mid] < key) {
low = mid + 1; // Continue searching in the right half
} else {
high = mid - 1; // Continue searching in the left half
}
}
return -1; // Key not found
}
In this implementation, we initialize low
and high
to the start and end indices of the array, respectively. We then enter a while loop that continues as long as the low
index is less than or equal to the high
index. Within the loop, we compute the mid
index and compare the element at that index with the key
. Based on the comparison, we adjust the low
and high
indices accordingly until we find the key
or determine it doesn't exist in the array.
Advantages of Non-Recursive Binary Search
-
Iterative Approach: The non-recursive binary search uses a loop instead of function calls, leading to improved performance and reduced memory overhead.
-
Enhanced Control: Developers have precise control over the loop and variables, making it easier to debug and understand the algorithm's flow.
-
Efficiency: Non-recursive binary search is often more efficient than its recursive counterpart due to the absence of function call overhead.
Use Cases for Non-Recursive Binary Search
Non-recursive binary search is suitable for scenarios where efficiency and controlled resource utilization are crucial. Some common use cases include:
- Searching in large, sorted datasets
- Real-time systems with strict memory constraints
- Implementing searching in environments where recursion is not supported or discouraged
Putting Non-Recursive Binary Search to Work
Let's put our non-recursive binary search to the test with a simple example. Suppose we have a sorted array of integers:
int[] arr = { 3, 6, 9, 12, 15, 18, 21, 24, 27, 30 };
We want to find the index of the element 18. We can utilize our binarySearch
method to achieve this:
int index = binarySearch(arr, 18);
if (index != -1) {
System.out.println("Element found at index: " + index);
} else {
System.out.println("Element not found in the array.");
}
Upon running this code, we will successfully locate the element 18 at index 5, showcasing the effectiveness of non-recursive binary search.
The Last Word
In conclusion, non-recursive binary search in Java is an efficient and effective method for searching in sorted arrays. Its iterative approach, enhanced control, and efficiency make it a valuable addition to any Java developer's toolkit. By mastering non-recursive binary search, you can optimize the search functionality in your Java applications, leading to improved performance and resource utilization.
I hope this guide has provided you with a solid foundation for understanding and implementing non-recursive binary search in Java. Happy coding!
Start optimizing your Java search algorithms today!
Explore Java programming and unleash the power of non-recursive binary search!