Solving Java's NoClassDefFoundError: A Step-by-Step Guide
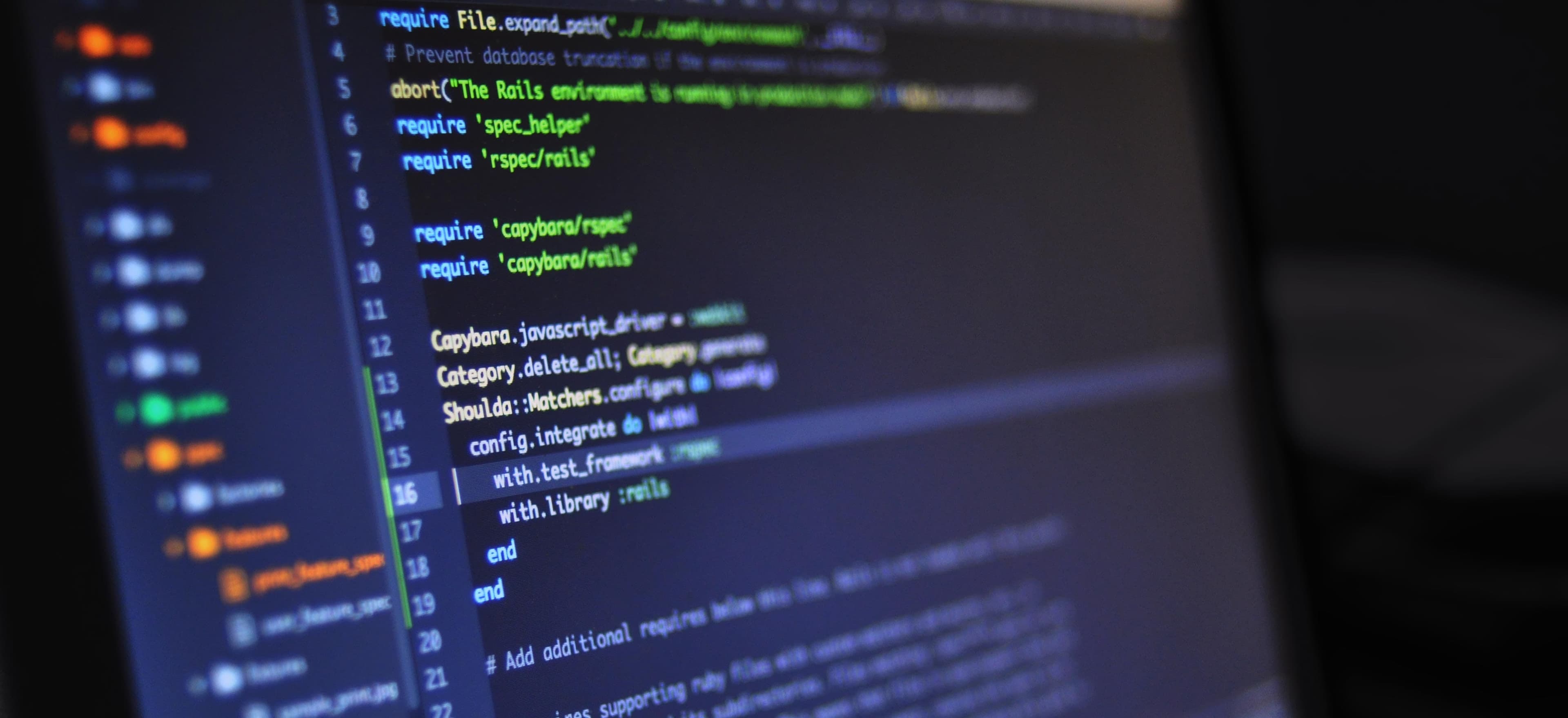
- Published on
Solving Java's NoClassDefFoundError: A Step-by-Step Guide
If you have been working with Java for some time, it's likely that you have encountered the infamous NoClassDefFoundError
at least once. This error is a common source of frustration for many Java developers, but fear not - in this guide, we'll explore what causes this error and provide a step-by-step approach to solving it.
Understanding NoClassDefFoundError
The NoClassDefFoundError
in Java is thrown when a particular class is present during compile time but is not found during runtime. This can happen for various reasons, such as missing dependencies, incorrect classpath configurations, or issues with the Java Virtual Machine (JVM).
Let's take a closer look at a few common scenarios that can lead to a NoClassDefFoundError
and how to address them.
Scenarios and Solutions
Missing Dependency Jars
One of the most frequent causes of NoClassDefFoundError
is missing dependency jars. When a class relies on another class that is not present in the classpath, this error can occur.
To resolve this issue, ensure that all required dependency jars are included in the classpath during compilation and runtime. You can use build tools like Maven or Gradle to manage dependencies efficiently.
Incorrect Classpath Configuration
Incorrect classpath configuration can also lead to NoClassDefFoundError
. This might occur if the classpath is not set properly, causing the JVM to be unable to locate the required classes.
Check the classpath configuration in your build scripts, IDE settings, or command line arguments to ensure that it includes all necessary paths for the classes to be found during runtime.
Version Mismatch or Conflicts
In some cases, a NoClassDefFoundError
can stem from conflicts or version mismatches between different libraries or modules. This can occur when multiple versions of the same library are being used, leading to classloading issues.
Utilize dependency management tools and techniques to resolve version conflicts and ensure that the correct versions of the required classes are available during runtime.
Issues with Classloaders
Classloaders play a vital role in Java's runtime environment. Problems with classloaders, such as custom classloader implementations or dynamic class loading, can trigger NoClassDefFoundError
under certain circumstances.
Review your classloader configurations and implementations to ensure that classes are being loaded correctly and that there are no conflicts with classloading mechanisms.
JVM Configuration Problems
In rare cases, NoClassDefFoundError
can be caused by issues with the JVM configuration. This could include insufficient memory allocation, incorrect JVM arguments, or other runtime environment issues.
Check and validate your JVM configuration settings to ensure that they are appropriate for your application's requirements.
Resolving NoClassDefFoundError - A Step-by-Step Approach
Now that we have explored the common causes of NoClassDefFoundError
, let's outline a step-by-step approach to resolving this error in your Java applications.
Step 1: Identify the Missing Class
When encountering a NoClassDefFoundError
, the first step is to identify the specific class that is missing at runtime. The error message accompanying the NoClassDefFoundError
typically includes the name of the missing class.
Step 2: Verify Classpath and Dependencies
Verify that the classpath is correctly configured and includes all required dependencies, including the jar files or directories containing the necessary classes. Use tools like javac
for compilation and java
for execution to ensure that the classpath is set appropriately.
Step 3: Check for Version Conflicts
If the issue persists, investigate for any potential version conflicts or mismatches between libraries or modules. Dependency management tools like Maven's dependency:tree
or Gradle's dependencies
task can help identify conflicting versions and resolve them.
Step 4: Review Classloader Configurations
Review any custom classloader implementations, dynamic class loading, or classloading configurations that might be causing the NoClassDefFoundError
. Ensure that the classloader hierarchy is not causing issues with class resolution.
Step 5: Validate JVM Configuration
Validate the JVM configuration, including memory settings, runtime arguments, and any other relevant configurations. Ensure that the JVM is adequately configured to support the required classes and resources.
Step 6: Test and Iterate
After implementing the above steps, test your application. If the NoClassDefFoundError
persists, iterate through the steps, revisiting each potential cause and ensuring that all configurations and dependencies are accurately set.
Example Code Demonstration
Let's consider an example where a NoClassDefFoundError
occurs due to a missing dependency. Suppose we have a simple Java program that uses a class from an external library. Without including the required library in the classpath, running the program would result in a NoClassDefFoundError
.
public class Main {
public static void main(String[] args) {
ExternalClass external = new ExternalClass();
external.doSomething();
}
}
In this example, ExternalClass
is part of an external library that needs to be included in the classpath. Failing to do so would lead to a NoClassDefFoundError
at runtime.
To resolve this, ensure that the external library containing ExternalClass
is included in the classpath during both compilation and execution.
The Last Word
The NoClassDefFoundError
in Java can be a perplexing issue, but armed with an understanding of its potential causes and a systematic approach to resolution, you can effectively tackle this error in your Java applications.
By identifying missing classes, verifying classpath and dependencies, addressing version conflicts, reviewing classloader configurations, and validating JVM settings, you can methodically troubleshoot and resolve NoClassDefFoundError
occurrences.
Remember to approach the resolution process systematically, testing your application after each step and iterating as needed to ensure a comprehensive solution.
In the ever-evolving landscape of Java development, encountering and overcoming errors like NoClassDefFoundError
are valuable learning experiences that contribute to your growth as a Java developer.
With a solid understanding of the intricacies involved in resolving such errors, you can navigate the challenges with confidence, ensuring the smooth operation of your Java applications.
Happy coding!
To further explore this topic, you can refer to the Java documentation for detailed insights into class loading and runtime behavior in Java. Additionally, you may find useful information in the Maven documentation for managing dependencies efficiently.