Securing Your Work: A Paranoid's Method to Backup Folders
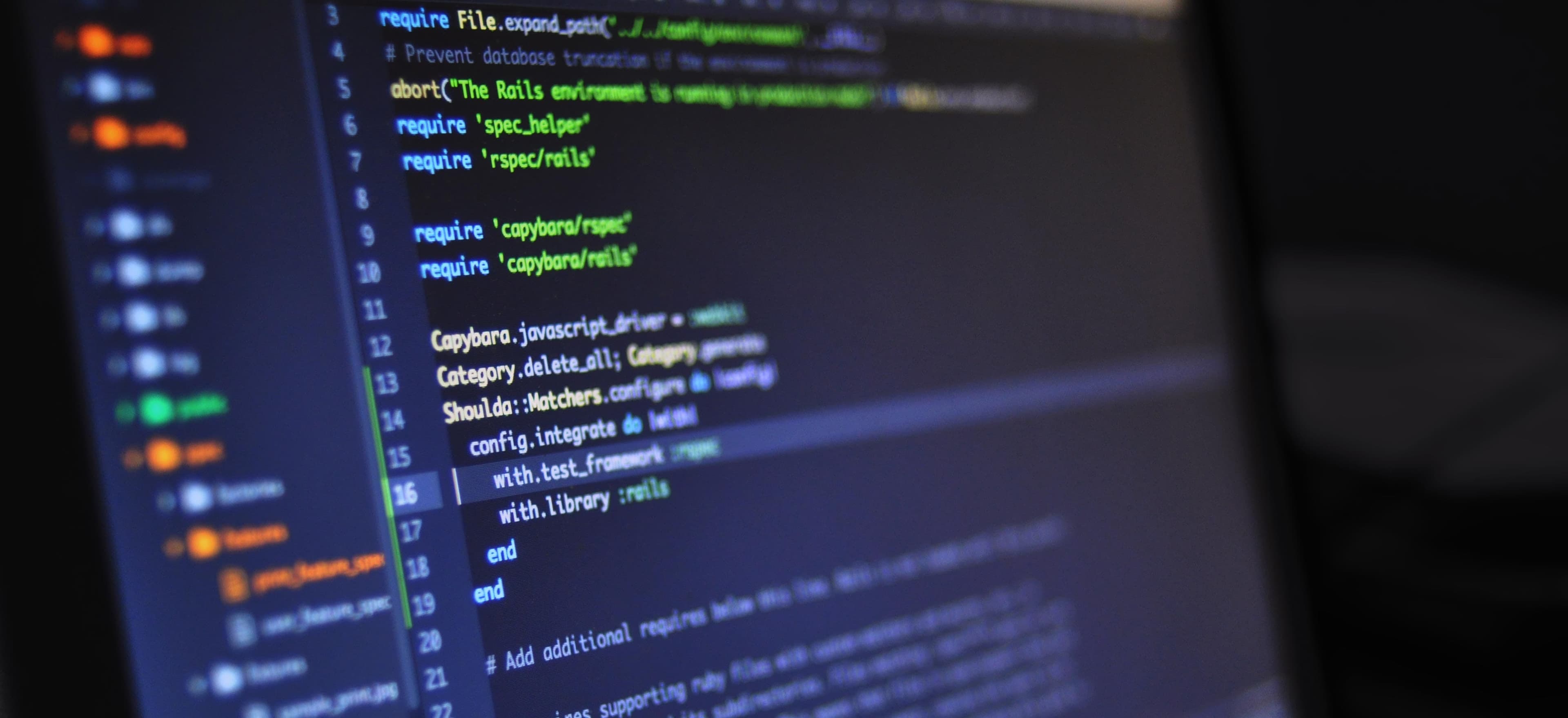
- Published on
Securing Your Work: A Paranoid's Method to Backup Folders
As a Java developer, ensuring the safety and security of your code is of utmost importance. Whether you are working on personal projects or collaborating with a team, the risk of losing valuable work due to unforeseen circumstances is always present. This is where backing up your folders becomes essential. In this post, we will explore a paranoid's method to backup folders using Java.
Why Backup Folders Using Java?
Backing up folders using Java provides a platform-independent solution that can be easily integrated into existing projects. Java's robust file and directory handling capabilities make it an ideal choice for implementing backup functionality. By creating a custom backup solution in Java, you have full control over the backup process and can tailor it to suit your specific requirements.
Understanding the Requirements
Before diving into the implementation, let's outline the requirements for our folder backup system:
- Automatic Backup: The system should be capable of automatically backing up specified folders at regular intervals.
- Versioning: Each backup should be versioned to allow for easy retrieval of previous versions of the backed-up folders.
- Error Handling: The system should handle errors gracefully and provide meaningful feedback in case of failures.
- Configurability: Users should be able to configure the backup settings, such as backup frequency, target location, and retention policy.
With these requirements in mind, let's proceed to implement a folder backup system in Java.
Implementing the Backup System
We will build the folder backup system using Java and demonstrate how each requirement is fulfilled.
Automatic Backup Using ScheduledExecutorService
Java provides the ScheduledExecutorService
that can be used to execute tasks at regular intervals. We can leverage this to implement the automatic backup functionality. Here's an example of how to schedule a backup task:
ScheduledExecutorService scheduler = Executors.newScheduledThreadPool(1);
Runnable backupTask = () -> {
// Perform backup
};
scheduler.scheduleAtFixedRate(backupTask, 0, 24, TimeUnit.HOURS); // Backup every 24 hours
In this code snippet, we create a ScheduledExecutorService
with a single thread and schedule a backup task to run every 24 hours. This ensures that our folders are automatically backed up at regular intervals.
Versioning the Backups
To implement versioning, we can simply append a timestamp to the backup folder name. This allows us to retain multiple versions of the backed-up folders without overwriting previous backups. Here's how we can achieve this:
String timestamp = new SimpleDateFormat("yyyyMMddHHmmss").format(new Date());
String backupFolderName = "backup_" + timestamp;
By incorporating the timestamp into the backup folder name, we ensure that each backup is uniquely identified and versioned.
Error Handling with Try-Catch Blocks
Error handling is a critical aspect of any robust system. In Java, we can use try-catch blocks to handle exceptions and provide meaningful feedback in case of failures. Here's an example of how to handle file copy errors during backup:
Path source = Paths.get("source-folder");
Path target = Paths.get("backup-location");
try {
Files.copy(source, target, StandardCopyOption.REPLACE_EXISTING);
System.out.println("Backup successful");
} catch (IOException e) {
System.err.println("Backup failed: " + e.getMessage());
}
In this example, we attempt to copy the source folder to the backup location. If an IOException occurs, we catch the exception and print an error message, providing information about the cause of the failure. This ensures that users are informed about any backup errors.
Configurability Using Properties Files
To allow users to configure the backup settings, we can use properties files to store the configurable parameters. Here's an example of a backup configuration properties file:
# Backup settings
backup.frequency=24
backup.target=/backup-location
backup.retention=7
In this properties file, we define the backup frequency in hours, the target location for the backups, and the retention period in days. By reading these settings from a properties file, users can easily customize the backup configuration.
A Final Look
In this post, we explored a paranoid's method to backup folders using Java. By implementing a custom backup system in Java, we addressed the requirements of automatic backup, versioning, error handling, and configurability. Leveraging Java's file and directory handling capabilities, we created a robust and flexible solution for securing our work.
By following the principles outlined in this post, you can ensure the safety and security of your code by implementing a reliable folder backup system using Java.
Implementing a backup system may seem like a paranoid approach, but in the realm of software development, it's better to be safe than sorry. With Java, the process can be streamlined and customized to fit your exact needs, providing you with peace of mind and a safety net for your valuable work. So go ahead, dive into the world of folder backups in Java, and keep your work secure!