Solving Microservices Data Consistency: A Chronicle
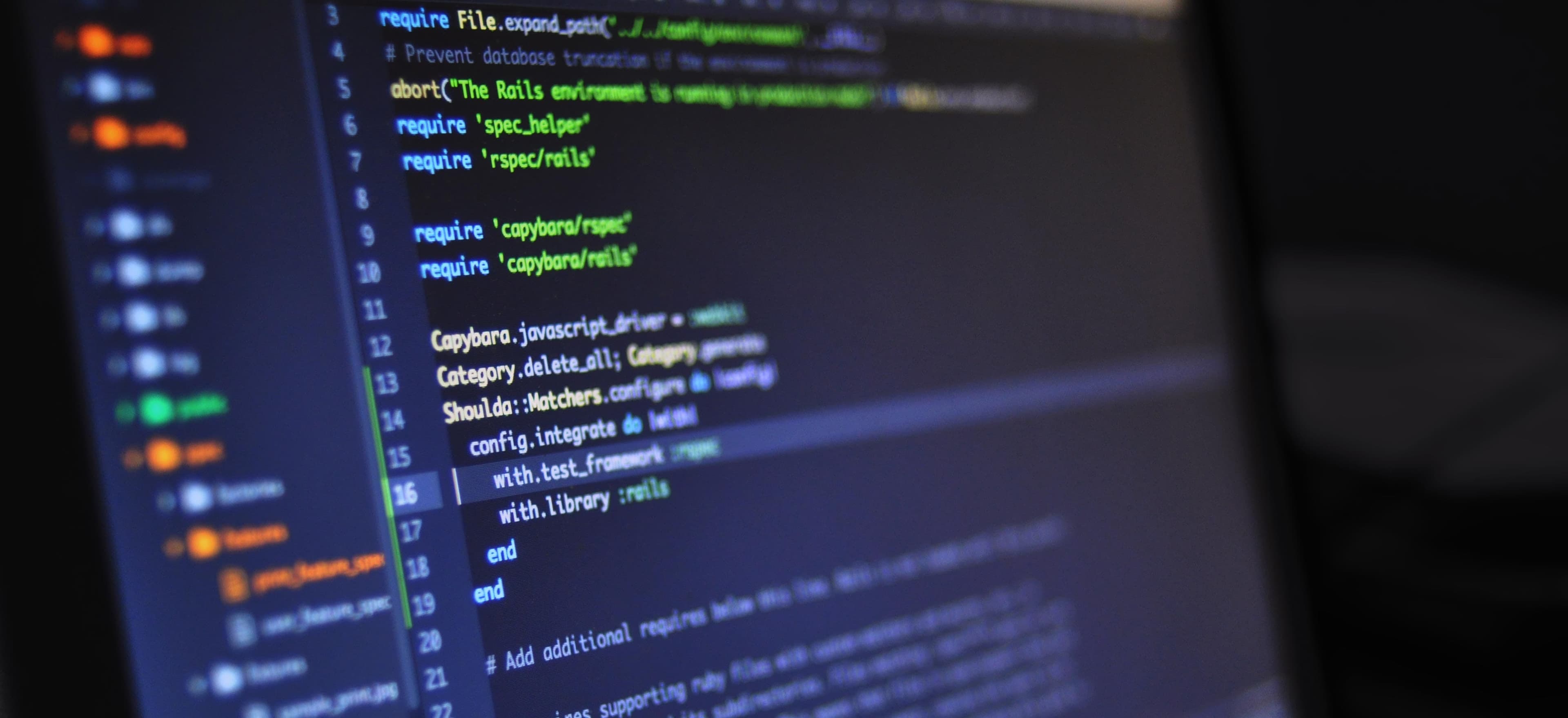
- Published on
Solving Microservices Data Consistency: A Chronicle
Microservices architecture has become the cornerstone of modern app development, providing agility and scalability. However, it brings along a new set of challenges, one of the most critical being data consistency. In this blog post, we will delve into the world of microservices and explore techniques and best practices for managing data consistency in a distributed environment.
Understanding the Challenge
In a microservices architecture, each service has its own private database, leading to a distributed data management model. This setup introduces complexities in maintaining data consistency across services, as the traditional ACID (Atomicity, Consistency, Isolation, Durability) properties of a single database are no longer directly applicable.
The CAP Theorem
Understanding the famous CAP (Consistency, Availability, Partition Tolerance) theorem is crucial in the context of microservices. The theorem states that in the event of a network partition, a system must choose between consistency and availability. Achieving both full consistency and availability during a partition is not possible.
Techniques for Managing Data Consistency
1. Synchronous Communication and Two-Phase Commits
Synchronous communication between microservices can help in achieving immediate data consistency. Two-phase commit protocols can be utilized to ensure that all services either commit or roll back a transaction. However, this approach has its drawbacks, such as increased complexity and decreased availability.
@Transactional
public void placeOrder(Order order) {
orderService.create(order);
paymentService.charge(order);
}
The synchronous nature can lead to cascading failures if one service becomes slow or unresponsive, affecting the entire system's performance.
2. Asynchronous Event-Driven Architecture
Employing an event-driven architecture with a message broker, such as Apache Kafka or RabbitMQ, allows microservices to communicate via events. This approach decouples services and enables eventual consistency, where each service reacts to events and updates its own database asynchronously.
@KafkaListener(topics = "orders")
public void handleOrderCreatedEvent(OrderCreatedEvent event) {
inventoryService.updateStock(event.getItem(), event.getQuantity());
}
Although this approach enhances scalability and fault tolerance, it introduces complexity in handling out-of-order events and ensuring idempotency.
3. CQRS Pattern
The Command Query Responsibility Segregation (CQRS) pattern separates the read and write operations, allowing for different models for each. Writes are handled by the command side, ensuring consistency within the service, while reads are served from the denormalized views on the query side, optimizing read performance.
// Command side
public void createProduct(Product product) {
productRepository.save(product);
eventPublisher.publishEvent(new ProductCreatedEvent(product.getId()));
}
By maintaining separate models for reading and writing data, the CQRS pattern facilitates better scalability and performance, especially in scenarios where the read and write loads differ significantly.
Best Practices
1. Design Atomic Business Transactions Carefully
When dealing with distributed transactions, it's vital to design atomic business transactions thoughtfully. Sagas, which are sequences of local transactions coordinated through asynchronous messaging, can help in maintaining consistency without the need for distributed transactions.
2. Versioning and Compatibility
As microservices evolve independently, it's imperative to manage data versioning and backward compatibility. Utilizing techniques such as schema evolution and consumer-driven contracts mitigates the risk of breaking changes and ensures smooth data interactions.
3. Embrace Polyglot Persistence
Each microservice should choose the most suitable database for its specific requirements, embodying the concept of polyglot persistence. Embracing different databases allows for better alignment with the service's needs, rather than a one-size-fits-all approach.
Final Thoughts
Navigating data consistency in a microservices architecture demands a combination of careful design, strategic patterns, and the right technological choices. While there's no one-size-fits-all solution, understanding the trade-offs and selecting the most suitable approach for each scenario is key to achieving data consistency in a distributed environment.
For further reading on implementing these techniques in Java microservices, consult Spring's guide to event-driven architecture and Axon Framework.
Remember, the road to data consistency in microservices might be riddled with complexities, but with the right strategies and a clear understanding of the underlying principles, it's a challenge that can be navigated successfully.