Bridging Gaps in the Data Knowledge Stack: A How-To
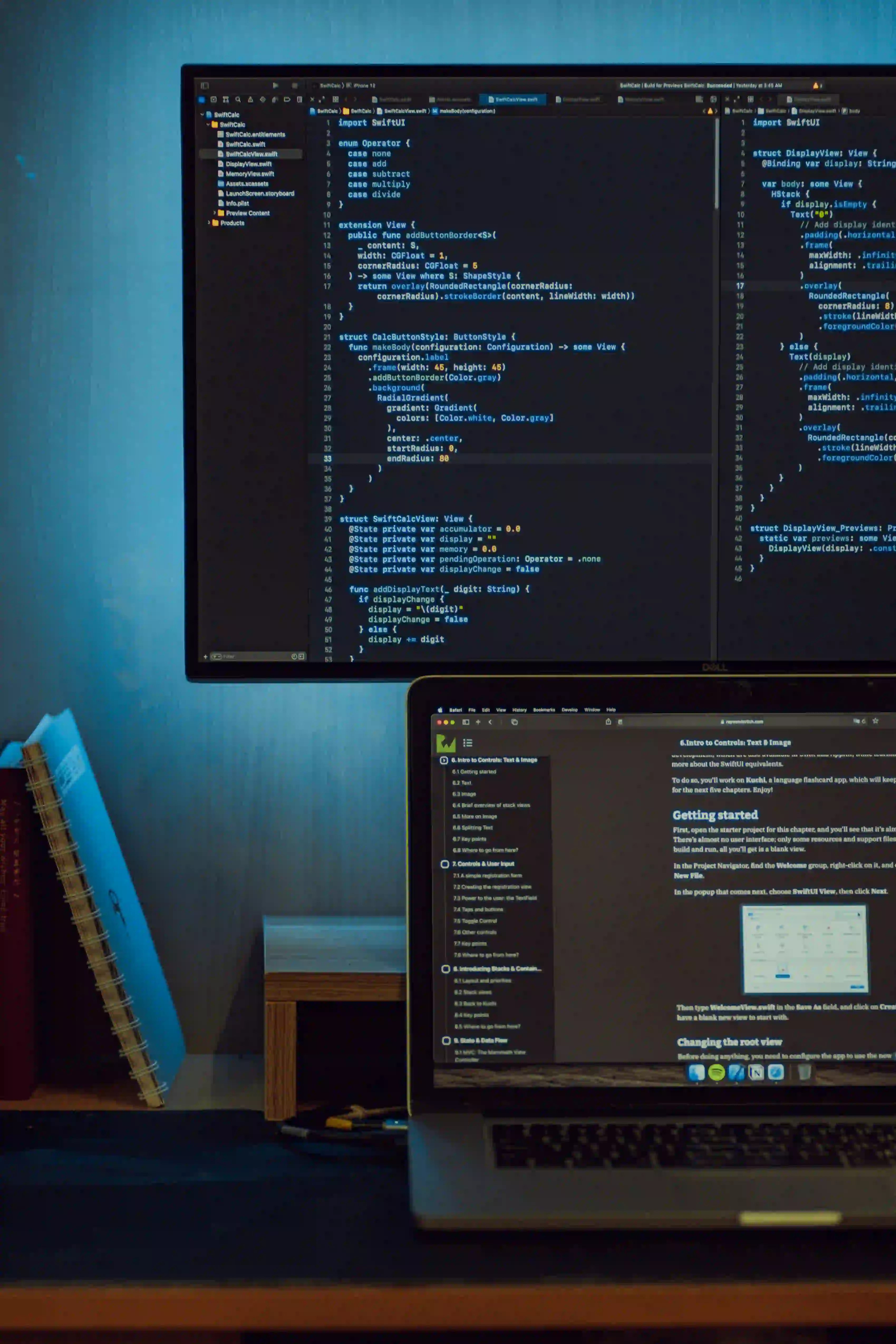
Bridging Gaps in the Data Knowledge Stack: A How-To
In today’s data-driven world, Java continues to serve as a powerful and versatile language for building high-performance applications. With its strong ecosystem and wide array of libraries, Java is an excellent choice for handling data-centric tasks. This blog post aims to provide a comprehensive guide on utilizing Java for data processing and analysis, thereby bridging the gap between traditional Java programming and the specialized world of data.
Why Java for Data Processing?
Java boasts several advantages when it comes to data processing, making it a robust choice for such tasks:
- Performance: Java’s efficient and optimized execution makes it suitable for handling large volumes of data.
- Rich Ecosystem: With libraries like Apache Commons Math and Weka, Java offers a rich ecosystem for mathematical and statistical operations.
- Scalability: Java’s robust concurrency support and the ability to leverage multi-threading make it suitable for scaling data processing tasks.
- Integration: Java can easily be integrated with Hadoop, Spark, and other big data frameworks, offering seamless data processing capabilities.
Getting Started with Java for Data Processing
Setting Up the Environment
Before diving into data processing, it is crucial to have the right tools and environment set up. For data processing in Java, the following tools are essential:
- Java Development Kit (JDK): Install the latest version of JDK from the official Oracle website or adopt OpenJDK.
- Integrated Development Environment (IDE): Choose an IDE like IntelliJ IDEA or Eclipse, offering robust support for Java development.
Once the environment is set up, you can begin leveraging Java for data processing.
Data Handling with Java Collections
Java’s built-in collections framework provides a powerful set of libraries for data handling. Let’s look at an example of how Java collections can be utilized to process and analyze data.
import java.util.ArrayList;
import java.util.List;
import java.util.stream.Collectors;
public class DataProcessing {
public static void main(String[] args) {
// Sample data
List<Integer> data = new ArrayList<>();
data.add(5);
data.add(10);
data.add(15);
// Processing data
List<Integer> result = data.stream()
.map(i -> i * 2)
.collect(Collectors.toList());
// Output the result
System.out.println(result);
}
}
In the above code snippet, we utilized Java collections along with the Stream API to process and transform the data. This exemplifies the versatility and ease of use of Java for data processing tasks.
Performing Statistical Analysis with Apache Commons Math
Apache Commons Math is a popular Java library that provides comprehensive mathematical and statistical components. Consider the following example to showcase how Apache Commons Math can be leveraged for statistical analysis.
import org.apache.commons.math3.stat.descriptive.DescriptiveStatistics;
public class StatisticalAnalysis {
public static void main(String[] args) {
// Sample data
double[] values = {1.2, 2.0, 3.5, 4.1, 5.3};
// Performing statistical analysis
DescriptiveStatistics stats = new DescriptiveStatistics();
for (double value : values) {
stats.addValue(value);
}
// Output the statistical results
System.out.println("Mean: " + stats.getMean());
System.out.println("Standard Deviation: " + stats.getStandardDeviation());
}
}
In this code snippet, Apache Commons Math is employed to compute the mean and standard deviation of a given set of data. This demonstrates the seamless integration of Java libraries for performing statistical operations.
Integrating Java with Big Data Frameworks
Java’s seamless integration with big data frameworks like Hadoop and Spark makes it a compelling choice for large-scale data processing. Let’s delve into a brief overview of utilizing Java with these frameworks.
Integrating with Hadoop
Hadoop, an Apache open-source framework, is widely used for distributed storage and processing of large datasets. Java, being the primary language for Hadoop’s MapReduce, offers native compatibility with Hadoop.
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.io.IntWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Job;
import org.apache.hadoop.mapreduce.Mapper;
import org.apache.hadoop.mapreduce.Reducer;
import org.apache.hadoop.mapreduce.lib.input.FileInputFormat;
import org.apache.hadoop.mapreduce.lib.output.FileOutputFormat;
import java.io.IOException;
import java.util.StringTokenizer;
public class WordCount {
public static class TokenizerMapper
extends Mapper<Object, Text, Text, IntWritable>{
private final static IntWritable one = new IntWritable(1);
private Text word = new Text();
public void map(Object key, Text value, Context context
) throws IOException, InterruptedException {
StringTokenizer itr = new StringTokenizer(value.toString());
while (itr.hasMoreTokens()) {
word.set(itr.nextToken());
context.write(word, one);
}
}
}
public static class IntSumReducer
extends Reducer<Text,IntWritable,Text,IntWritable> {
private IntWritable result = new IntWritable();
public void reduce(Text key, Iterable<IntWritable> values,
Context context
) throws IOException, InterruptedException {
int sum = 0;
for (IntWritable val : values) {
sum += val.get();
}
result.set(sum);
context.write(key, result);
}
}
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "word count");
job.setJarByClass(WordCount.class);
job.setMapperClass(TokenizerMapper.class);
job.setCombinerClass(IntSumReducer.class);
job.setReducerClass(IntSumReducer.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(IntWritable.class);
FileInputFormat.addInputPath(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
The above code snippet demonstrates a simple Word Count example using Hadoop’s MapReduce framework and Java. This showcases how Java seamlessly integrates with Hadoop for distributed data processing.
Leveraging Java with Apache Spark
Apache Spark, an in-memory data processing engine, offers excellent support for Java through its Spark Java API.
import org.apache.spark.api.java.JavaRDD;
import org.apache.spark.api.java.JavaSparkContext;
import org.apache.spark.sql.SparkSession;
public class SparkExample {
public static void main(String[] args) {
SparkSession spark = SparkSession
.builder()
.appName("Java Spark Example")
.config("spark.master", "local")
.getOrCreate();
JavaSparkContext jsc = new JavaSparkContext(spark.sparkContext());
// Sample data
JavaRDD<Integer> data = jsc.parallelize(List.of(1, 2, 3, 4, 5));
// Performing data transformation
JavaRDD<Integer> result = data.map(x -> x * 2);
// Output the result
System.out.println(result.collect());
spark.stop();
}
}
In the above code snippet, Java is used to create a Spark application for data transformation. This demonstrates the seamless integration of Java with Apache Spark for in-memory data processing.
My Closing Thoughts on the Matter
Java’s versatility and robustness make it an excellent choice for data processing and analysis. With its efficient performance, rich ecosystem, and seamless integration with big data frameworks, Java serves as a compelling option for bridging the gap between traditional Java programming and the specialized world of data.
In conclusion, by harnessing the power of Java for data processing, developers can leverage its strong capabilities to handle and analyze data effectively, contributing to the ever-expanding data-driven landscape.
Bridging the gaps with Java - welcoming it in the data knowledge stack is a step towards empowering powerful and efficient data processing solutions.
So, let Java lead the way in the world of data!
References:
Start your data journey with Java today!