Unlocking Code Stability: The Power of Immutability
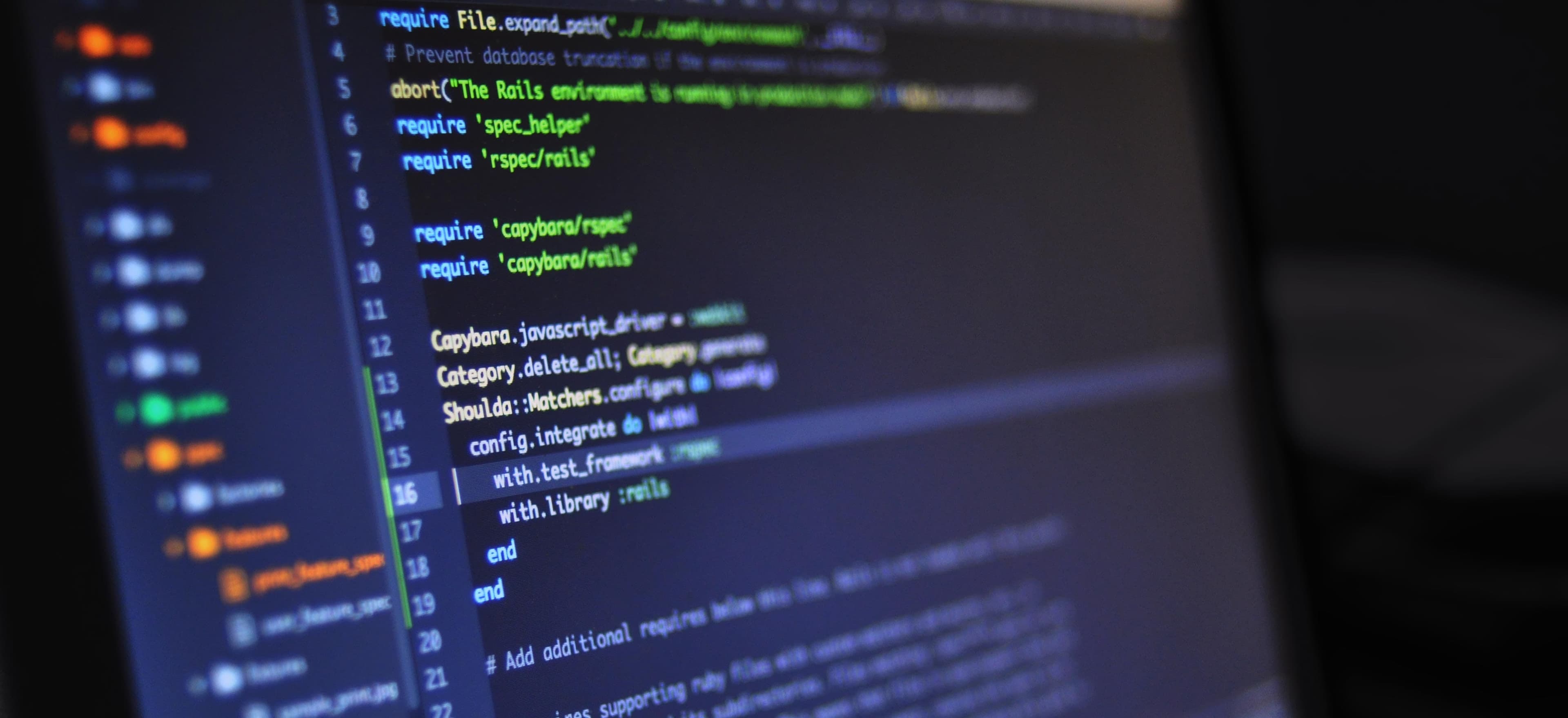
- Published on
Unlocking Code Stability: The Power of Immutability
In the realm of modern software development, writing code that is not only functional but also stable has become paramount. Java, being one of the most widely used programming languages, offers various features that aid in achieving code stability. One such feature is immutability, which plays a pivotal role in ensuring that an application's code remains robust and reliable.
What is Immutability?
In the context of Java, immutability refers to the inability of an object to be modified after it has been created. This means that once an immutable object is initialized with a set of values, those values cannot be altered.
The Power of Immutability
1. Thread Safety
Immutability inherently provides thread safety. Since immutable objects cannot be modified, they can be shared across multiple threads without the risk of data corruption. This eliminates the need for synchronization mechanisms such as locks or semaphores, thereby simplifying concurrent programming.
2. Code Simplicity and Clarity
By designing classes to be immutable, the complexity of the code is reduced. With immutable objects, there's no need to worry about unexpected changes to the object's state, leading to clearer and more predictable code.
3. Enhanced Security
Immutable objects are inherently more secure since their state cannot be altered after instantiation. This property makes them resistant to tampering and helps prevent security vulnerabilities such as injection attacks.
4. Promotes Reusability
Immutable objects are inherently shareable as they can be freely passed around without the risk of unintended modifications. This promotes reusability and interoperability within the codebase.
5. Facilitates Caching
Immutable objects are ideal candidates for caching. Once created, they can be safely cached without the risk of the cached data being modified inadvertently.
Example of Immutability in Java
Let's explore the concept of immutability through an example in Java. Consider the following Person
class:
public final class Person {
private final String name;
private final int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
In this example, the Person
class is designed to be immutable. The name
and age
fields are declared as final
, and once they are set via the constructor, they cannot be modified. By adhering to immutability, this class ensures that once a Person
object is created, its state remains constant throughout its lifecycle.
Why Immutability?
The choice of immutability for the Person
class is driven by the need to ensure that the fundamental attributes of a person, such as their name and age, remain consistent and unaltered. By enforcing immutability, the class provides robustness and a deterministic behavior, which is crucial in scenarios where data integrity is paramount.
Immutable Collections
Java provides immutable counterparts to the standard mutable collections through the Collections.unmodifiable...()
methods. For instance, Collections.unmodifiableList()
returns an unmodifiable view of the specified list. This is particularly useful in scenarios where you want to prevent the modification of a collection while still allowing it to be shared across different parts of the system.
How to Create Immutable Classes
Creating immutable classes in Java involves adhering to a set of principles:
1. Declare the class as final
By declaring a class as final
, you prevent it from being subclassed, which could potentially lead to mutability through inheritance.
2. Declare fields as private and final
Private and final fields, as shown in the Person
example, ensure that the state of the object cannot be modified once it's constructed.
3. Omit setter methods
Immutable classes should not contain setter methods that allow the modification of their state. Instead, the state should be initialized through the constructor.
4. Restrict mutable components
If an immutable class contains mutable components, such as mutable collections, extra care must be taken to ensure that these components do not compromise the overall immutability of the class.
By following these principles, you can design classes that exhibit the characteristics of immutability, thereby contributing to the stability and reliability of your codebase.
The Closing Argument
The concept of immutability in Java is not only a best practice but also a powerful tool for enhancing code stability. By leveraging immutability, you can simplify your code, improve thread safety, enhance security, and promote reusability. Immutable objects foster a sense of predictability and reliability, which are crucial aspects in the development of robust and maintainable software systems.
In the dynamic landscape of software development, embracing immutability can be a game-changer. It helps in crafting code that stands the test of time, maintains its integrity, and ultimately contributes to the creation of resilient and stable software solutions.
Incorporating the principles of immutability into your Java application's design can lead to a paradigm shift in how you approach code stability and robustness. By seizing the power of immutability, you pave the way for code that not only functions correctly but also withstands the trials of continuous evolution and expansion.
Immutability is not just a concept; it's a force that propels your code towards unparalleled stability and reliability.
So, embrace immutability, and unlock the true potential of code stability in your Java applications.