Boost Your JAX-RS Apps: Easy CDI Caching Tips!
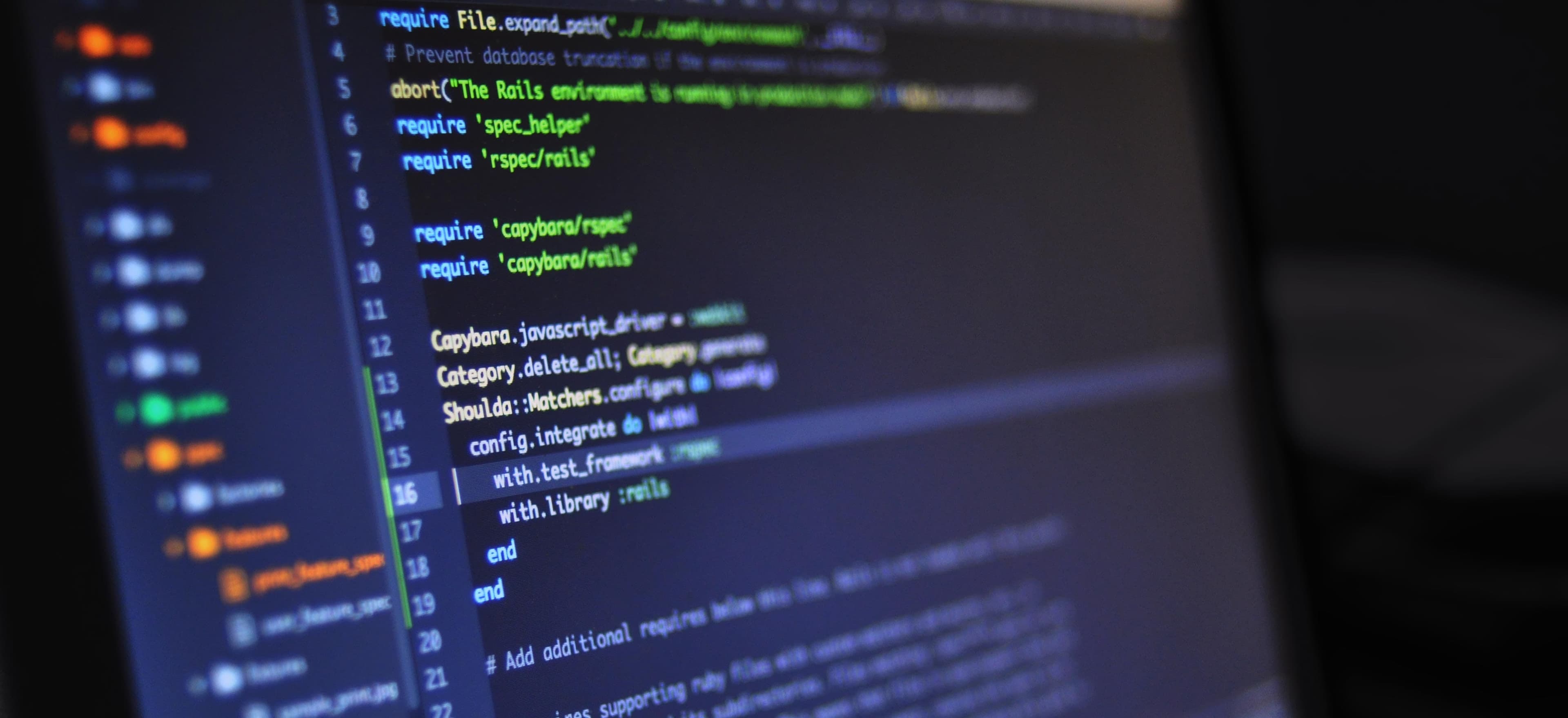
- Published on
Boost Your JAX-RS Apps: Easy CDI Caching Tips!
When it comes to developing Java RESTful web services, JAX-RS is a popular choice due to its simplicity and power. However, as your application grows, you may encounter performance issues, especially when dealing with database or external API calls. This is where the power of CDI (Contexts and Dependency Injection) caching comes into play. In this post, we will explore some easy tips to boost your JAX-RS applications using CDI caching.
What is CDI Caching?
CDI caching allows you to store the results of expensive method calls and reuse them when the same method is called with the same parameters. This can significantly improve the performance of your application by reducing the computational overhead of repeatedly executing the same logic. CDI caching is particularly beneficial in scenarios where the result of a method call remains constant for a given input.
Setting Up CDI Caching in JAX-RS
To illustrate how CDI caching can be integrated into a JAX-RS application, let's consider a basic example of a RESTful service that retrieves user details from a database. We'll use the CDI caching mechanism to cache the retrieved user details to improve the response time for subsequent requests.
Step 1: Add the CDI Cache Annotation
@RequestScoped
public class UserService {
@Inject
private UserDAO userDAO;
@CacheResult
public User getUserDetails(String userId) {
return userDAO.getUserById(userId);
}
}
In this example, the @CacheResult
annotation is used to mark the getUserDetails
method for caching. This means that the result of this method will be cached based on its input parameters.
Step 2: Enable CDI Caching in JAX-RS Resource
@Path("/users")
@RequestScoped
public class UserResource {
@Inject
private UserService userService;
@GET
@Path("/{userId}")
@Produces(MediaType.APPLICATION_JSON)
public Response getUserDetails(@PathParam("userId") String userId) {
User userDetails = userService.getUserDetails(userId);
return Response.ok(userDetails).build();
}
}
In the UserResource
class, we inject the UserService
and call the getUserDetails
method to retrieve user details. Thanks to the CDI caching, the result will be cached after the first call and reused for subsequent calls with the same userId
.
Step 3: Enable CDI Caching in JAX-RS Application
@ApplicationScoped
@ApplicationPath("/api")
public class MyApp extends Application {
@Override
public Set<Class<?>> getClasses() {
Set<Class<?>> resources = new HashSet<>();
// Add JAX-RS resource classes
resources.add(UserResource.class);
// Enable CDI caching interceptor
resources.add(CacheInterceptor.class);
return resources;
}
}
In the MyApp
class, we enable the CDI caching interceptor CacheInterceptor
to intercept the method calls marked with the @CacheResult
annotation and handle the caching logic.
Advantages of Using CDI Caching in JAX-RS
Now that we've implemented CDI caching in our JAX-RS application, let's explore the advantages it offers:
Improved Performance
By caching the results of method calls, CDI caching reduces the need to repeatedly execute the same logic, resulting in improved response times and overall performance of the application.
Reduced Database/External API Calls
In scenarios where the method results depend on database queries or external API calls, CDI caching can eliminate the need to make redundant calls, thereby reducing the load on the database or external services.
Simplified Implementation
Integrating CDI caching into your JAX-RS application is relatively straightforward, especially when leveraging annotations such as @CacheResult
. This simplifies the caching logic and makes the code more maintainable.
Best Practices for CDI Caching in JAX-RS
While CDI caching can greatly enhance the performance of your JAX-RS application, it's essential to adhere to best practices to ensure its effectiveness:
Identify Cacheable Methods
Not all methods are suitable for caching. Identify methods that have deterministic behavior and whose results remain constant for a given input. These are ideal candidates for CDI caching.
Monitor Cache Hit Ratio
Keep track of the cache hit ratio to ensure that the caching mechanism is effectively reducing the computational overhead. A high cache hit ratio indicates efficient caching, while a low ratio may necessitate a reevaluation of the caching strategy.
Set Cache Expiry and Eviction Policies
Configure the cache with appropriate expiry and eviction policies based on the nature of the cached data. For example, set an expiry time for transient data and implement eviction policies to remove stale cache entries.
The Closing Argument
In conclusion, integrating CDI caching into your JAX-RS applications can yield significant performance improvements by reducing computational overhead and minimizing redundant method executions. By following the easy tips outlined in this post and adhering to best practices, you can harness the power of CDI caching to optimize the responsiveness and efficiency of your RESTful web services.
Boost your JAX-RS applications with CDI caching today and experience the difference in performance!
Remember, the key to successful CDI caching lies in identifying cacheable methods, monitoring cache hit ratios, and applying appropriate cache expiry and eviction policies to ensure optimal performance. Happy coding!
To delve deeper into CDI caching and JAX-RS optimization, check out the CDI specification and JAX-RS documentation.
Checkout our other articles