Jackson & Kotlin: Solving Reified Type Challenges
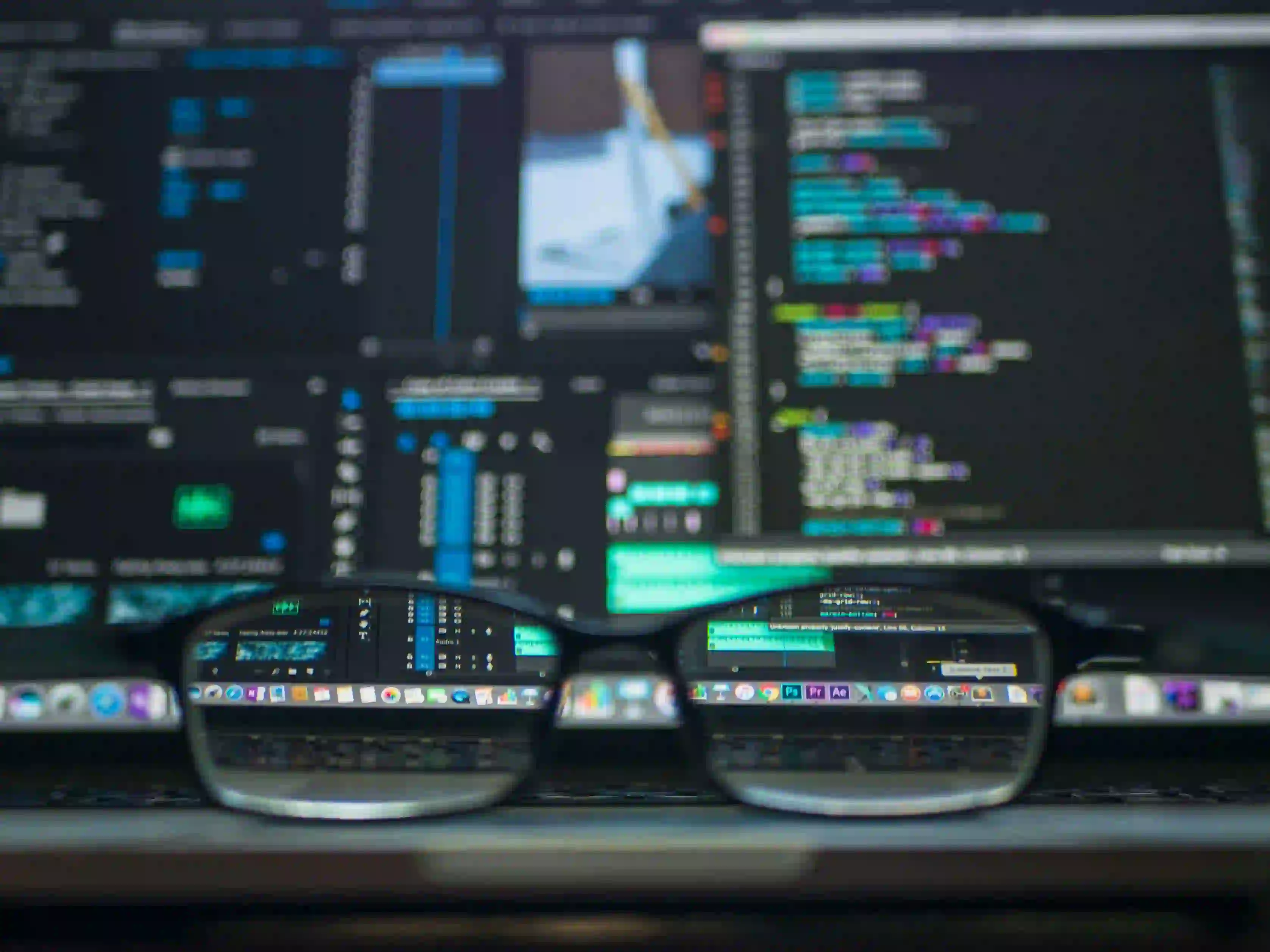
Solving Reified Type Challenges in Java with Jackson & Kotlin
When working with Java, you may come across scenarios where you need to work with generic types at runtime, often leading to the challenge of obtaining type information at runtime due to type erasure. This becomes especially pertinent when dealing with JSON serialization and deserialization using libraries like Jackson. In Kotlin, the reified
keyword comes to the rescue, enabling us to access and utilize type information at runtime, a feature that is not inherently available in Java. In this article, we will explore how Kotlin's reified
type can be leveraged to tackle the reified type challenges in Java when working with Jackson for JSON processing.
Understanding the Reified Type Challenge
In Java, due to type erasure, generic type information is not retained at runtime, making it challenging to work with generic types and obtain their type information. For example, when using Jackson to deserialize JSON into a generic type, obtaining the concrete type at runtime becomes a hurdle. This leads to the need for workarounds and compromises, often resulting in verbose and error-prone code.
Leveraging Kotlin's Reified Type
Kotlin introduces the reified
keyword, which allows us to access type information at runtime for generic types. This means that when using Kotlin in combination with Java, we can harness the power of reified
to overcome the reified type challenges that Java presents, especially when working with libraries like Jackson for JSON processing.
Implementing Reified Type Handling
Let's delve into an example to showcase how Kotlin's reified
type can be used to address the reified type challenges when working with Jackson in Java.
Consider the following Java class that uses Jackson for JSON deserialization:
import com.fasterxml.jackson.databind.ObjectMapper;
public class JsonDeserializer {
private final ObjectMapper objectMapper = new ObjectMapper();
public <T> T deserialize(String json, Class<T> valueType) throws IOException {
return objectMapper.readValue(json, valueType);
}
}
In this scenario, the Class<T>
parameter is used to pass the type information for deserialization. However, when working with generic types and attempting to deserialize JSON into a generic type, we encounter the reified type challenge where the type information is lost due to erasure.
Now, let's see how Kotlin's reified
type comes to the rescue. We can leverage an inline function in Kotlin to access the reified type information and pass it to the Java method for deserialization:
import com.fasterxml.jackson.databind.ObjectMapper
import com.fasterxml.jackson.module.kotlin.jacksonTypeRef
inline fun <reified T> JsonDeserializer.deserialize(json: String): T {
return deserialize(json, jacksonTypeRef<T>())
}
fun <T> JsonDeserializer.deserialize(json: String, valueType: Class<T>): T {
return objectMapper.readValue(json, valueType)
}
In this Kotlin code, the inline
function with reified
type parameter T
is used to deserialize JSON. The jacksonTypeRef
function is part of Jackson's Kotlin module and is used to capture the type information of T
. With this approach, we can now effectively deserialize JSON into a generic type without losing the type information.
The Power of Reified Types
By incorporating Kotlin's reified
type, we have successfully addressed the reified type challenges in Java when working with Jackson for JSON processing. This not only simplifies the code but also improves type safety and reduces the potential for runtime errors when dealing with generic types and JSON serialization/deserialization.
The Closing Argument
In conclusion, the introduction of reified
types in Kotlin opens up new possibilities for solving challenges that arise from type erasure in Java. By seamlessly integrating Kotlin's reified
types with Java code, we can overcome the limitations posed by type erasure and streamline processes such as JSON serialization and deserialization when using libraries like Jackson. Embracing the strengths of both Kotlin and Java allows for a more robust and elegant approach to tackling reified type challenges, ultimately enhancing the developer experience and the reliability of the codebase.
In your own projects, consider harnessing the power of Kotlin's reified
types to handle reified type challenges in Java, especially when working with JSON processing libraries like Jackson. Striking a balance between Kotlin and Java enables you to elevate the efficiency and robustness of your code while simplifying complex tasks.
By embracing the power of reified
types, you can conquer the intricacies of working with generic types in Java and pave the way for more expressive and concise code, ultimately bolstering the resilience and flexibility of your applications.
Remember, the combination of Kotlin's reified
types and Java offers a potent solution to the reified type challenges you encounter, empowering you to approach generic type handling with confidence and clarity.
So why not give it a try in your next project and experience the difference firsthand?
And for further insights into bridging Kotlin and Java, don't forget to explore the official Kotlin documentation, where you can uncover a wealth of knowledge to enrich your understanding and utilization of these powerful languages.
Here's to leveraging the prowess of Kotlin's reified
types to conquer reified type challenges and elevate your Java development journey!