Optimizing Spring Boot Startup Time
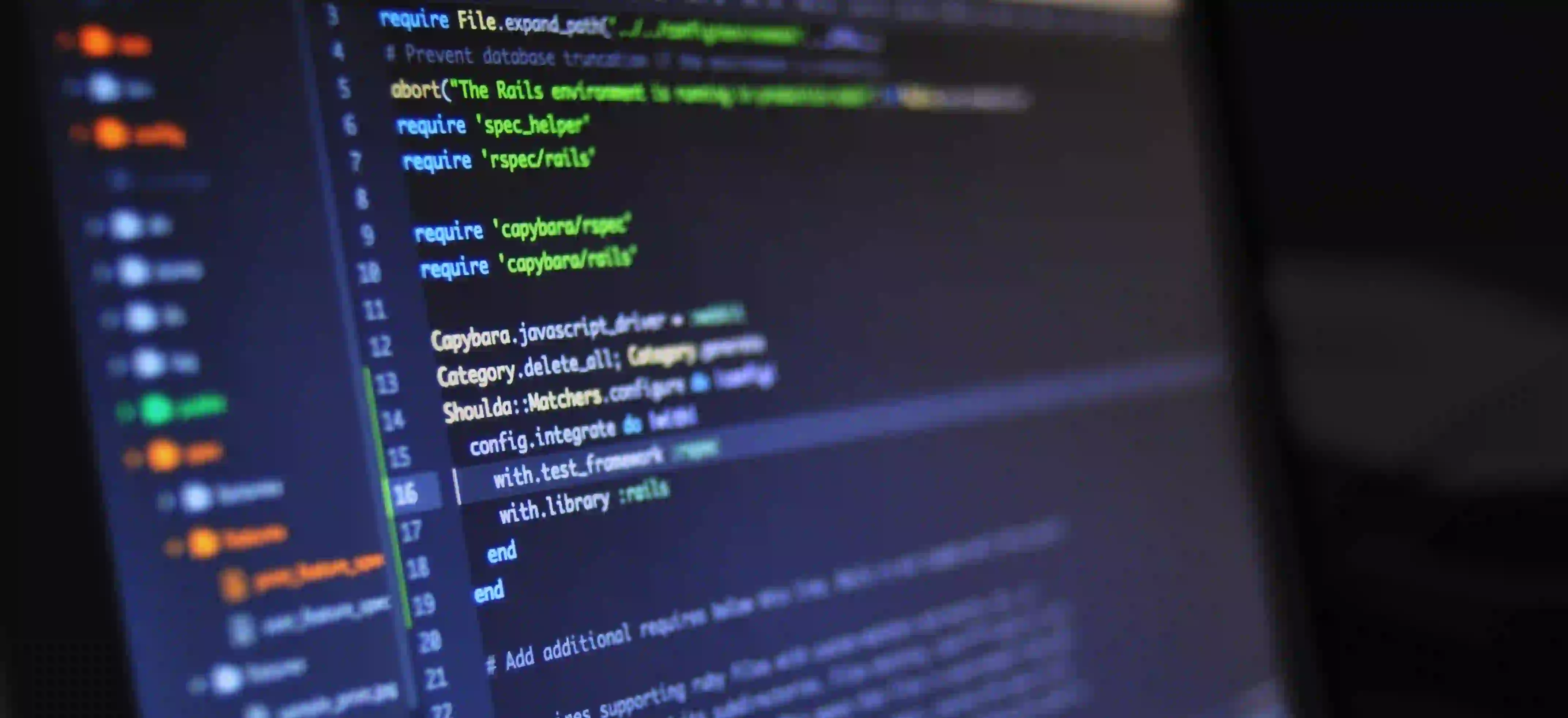
Optimizing Spring Boot Startup Time
Spring Boot is a popular Java-based framework for building standalone, production-grade applications. However, as your Spring Boot application grows, you may encounter longer startup times, which can impact user experience and scalability. In this post, we'll discuss strategies for optimizing Spring Boot startup time to ensure efficient performance.
1. Minimize Classpath Scanning
Spring Boot performs classpath scanning to discover beans, components, and configurations. However, extensive classpath scanning can significantly impact startup time, especially in large applications with numerous dependencies.
To mitigate this, utilize the @ComponentScan
annotation with specific packages to limit the scope of scanning. This approach reduces the time spent on scanning irrelevant components and boosts startup performance.
@ComponentScan(basePackages = "com.yourpackage")
public class YourApplication {
// ...
}
By specifying the base package, Spring Boot focuses on scanning only the necessary components, thereby accelerating startup time.
2. Analyze Dependencies
Analyze your application's dependencies using tools like JFrog Xray or JDepend. Identify and eliminate unnecessary dependencies and transitive dependencies that contribute to bloating the application. Trim down your dependencies to the essentials to streamline the startup process.
Additionally, consider using the --thin
or --executable
options with Spring Boot's Gradle or Maven plugins to create a lightweight, optimized JAR file that excludes unnecessary dependencies.
3. Utilize Spring Initializr
Spring Initializr is a powerful tool for generating Spring Boot projects with tailored dependencies and configurations. By selecting only the necessary dependencies and features during project initialization, you can prevent unnecessary bloat and ensure a lean, efficient project setup, ultimately reducing startup time.
4. Leverage Spring Profiles
Spring profiles enable you to define different configurations for various environments, such as development, testing, and production. By carefully managing profiles and activating only the required components for each environment, you can minimize startup overhead and enhance performance.
5. Implement Lazy Initialization
Leverage Spring's lazy initialization feature to defer bean creation until they are explicitly requested. By annotating beans with @Lazy
, you instruct Spring to create them only when they are first accessed. This approach can be particularly beneficial for large applications with extensive bean dependencies, effectively deferring the initialization of non-essential components until necessary.
@Lazy
@Component
public class YourComponent {
// ...
}
6. Enable Caching
Utilize caching mechanisms, such as Spring's built-in caching abstraction or third-party caching frameworks like Ehcache or Redis, to cache computationally expensive or frequently accessed data. Caching can significantly reduce the workload during startup by providing precomputed results and minimizing resource-intensive operations.
7. Optimize Database Access
Efficient database access is crucial for minimizing startup time. Utilize connection pooling techniques, optimize database queries, and consider asynchronous initialization of database-related components to prevent blocking the application's startup process.
Additionally, tools like Hibernate's second-level caching and Spring Data JPA's query result caching can enhance database performance and reduce initialization overhead.
Final Considerations
Optimizing Spring Boot startup time is essential for delivering responsive, scalable applications. By implementing strategies such as minimizing classpath scanning, analyzing dependencies, leveraging Spring Initializr, and embracing lazy initialization, you can significantly enhance your application's startup performance.
Prioritize a lean, efficient setup, and fine-tune configurations to eliminate unnecessary overhead. Additionally, leverage caching, optimize database access, and utilize profiling tools to identify bottlenecks and areas for improvement.
Through careful optimization and strategic utilization of Spring Boot's features, you can ensure that your application starts up swiftly and delivers exceptional performance.
For further reference, consider exploring the official Spring Boot documentation and the Spring Boot performance tips.