Common Mistakes in Generating Fibonacci Streams
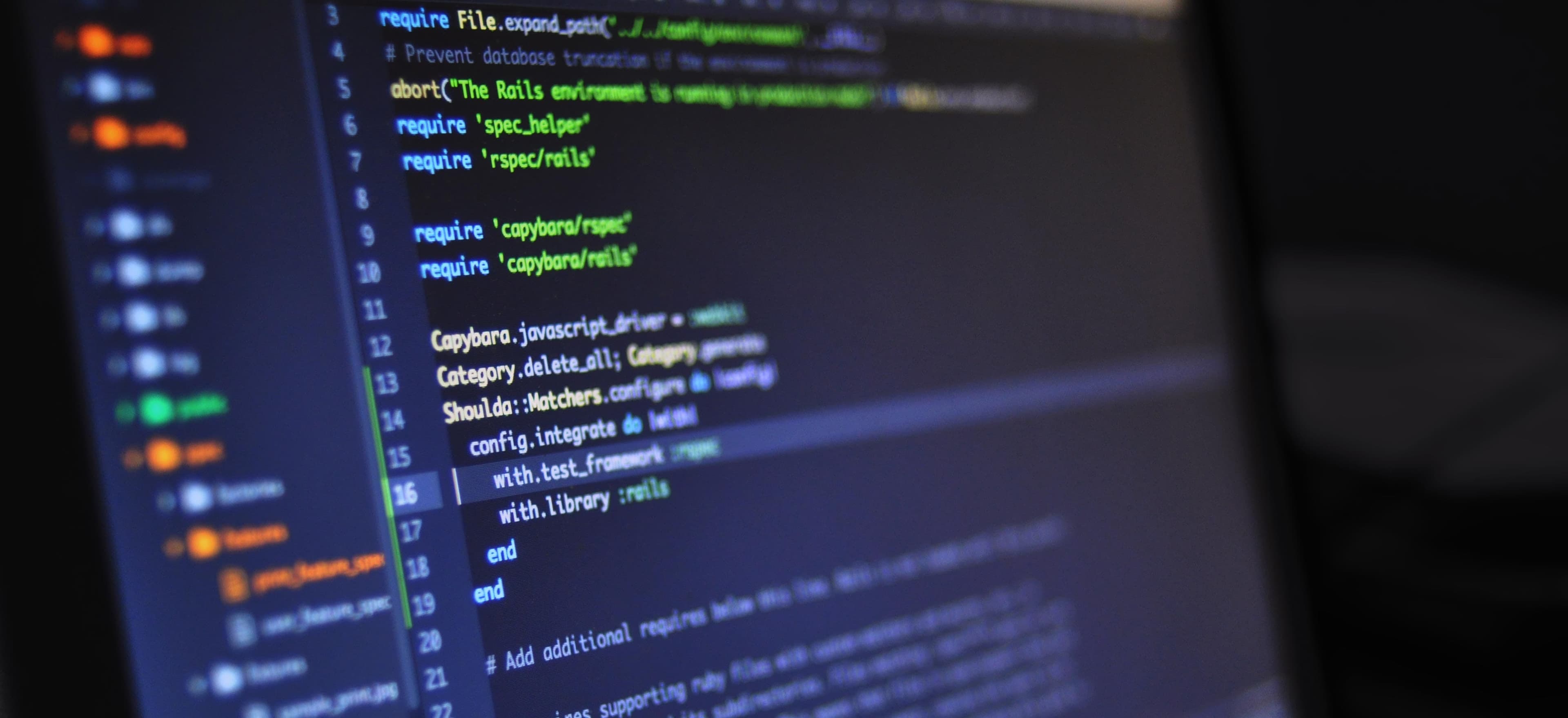
- Published on
Common Mistakes in Generating Fibonacci Streams
The Fibonacci sequence is one of the most celebrated numerical patterns found in nature, mathematics, and computer science. Generating Fibonacci streams can be deceptively simple, yet there are several common pitfalls that many developers encounter. In this blog post, we will explore these mistakes, providing insights and code snippets in Java that highlight how to avoid them.
The Fibonacci Sequence Overview
Before delving into the mistakes, let's clarify the Fibonacci sequence. It starts with two numbers, 0 and 1, and each subsequent number is the sum of the two preceding ones. Mathematically, it can be represented as:
- F(0) = 0
- F(1) = 1
- F(n) = F(n-1) + F(n-2) for n > 1
Here’s how the first few terms of the Fibonacci sequence look: 0, 1, 1, 2, 3, 5, 8, 13, 21, ... Knowing this sequence is essential for generating Fibonacci streams effectively.
Mistake 1: Inefficient Recursive Implementations
One of the most common mistakes developers make is relying on a naive recursive implementation of the Fibonacci sequence. While this approach is straightforward, it suffers from exponential time complexity.
Here's a naive recursive implementation example:
public class Fibonacci {
public static int fibonacciRecursive(int n) {
if (n <= 1) {
return n;
}
return fibonacciRecursive(n - 1) + fibonacciRecursive(n - 2);
}
public static void main(String[] args) {
int n = 10; // Position in the Fibonacci sequence
System.out.println("Fibonacci number at position " + n + " is: " + fibonacciRecursive(n));
}
}
The Issue
This code can lead to excessive function calls as it recalculates F(n-1) and F(n-2) multiple times. For larger values of n, this becomes prohibitively slow.
Why is this a problem?
The time complexity is O(2^n), making it infeasible for values greater than about 30.
Solution: Memoization
A more efficient approach is to use memoization, which stores already computed values for reuse.
import java.util.HashMap;
public class Fibonacci {
private static HashMap<Integer, Integer> memo = new HashMap<>();
public static int fibonacciMemo(int n) {
if (n <= 1) {
return n;
}
if (memo.containsKey(n)) {
return memo.get(n);
}
int result = fibonacciMemo(n - 1) + fibonacciMemo(n - 2);
memo.put(n, result);
return result;
}
public static void main(String[] args) {
int n = 10;
System.out.println("Fibonacci number at position " + n + " is: " + fibonacciMemo(n));
}
}
Why this works:
- Time complexity is reduced to O(n) due to saved state.
- You only compute each Fibonacci number once, making the algorithm significantly faster.
Mistake 2: Forgetting Base Cases
Another frequent mistake is neglecting base cases in recursive functions. Skipping these will lead to infinite recursion or incorrect results.
When implementing a recursive method to generate Fibonacci numbers, ensure that you include appropriate base case checks.
Correct Implementation Example
public class Fibonacci {
public static int fibonacci(int n) {
if (n < 0) {
throw new IllegalArgumentException("Input cannot be negative");
}
if (n == 0) {
return 0;
}
if (n == 1) {
return 1;
}
return fibonacci(n - 1) + fibonacci(n - 2);
}
public static void main(String[] args) {
System.out.println(fibonacci(10)); // Output: 55
}
}
Why it's critical:
Proper handling of base cases can prevent infinite loops and ensure your program functions correctly, yielding the right results for edge cases like F(0) and F(1).
Mistake 3: Using Unbounded Data Types
Sometimes, developers make the error of choosing data types that cannot hold large Fibonacci numbers, leading to integer overflow.
Correct Implementation Example
Use long
or BigInteger
for larger sequences:
import java.math.BigInteger;
public class Fibonacci {
public static BigInteger fibonacci(int n) {
if (n == 0) return BigInteger.ZERO;
if (n == 1) return BigInteger.ONE;
BigInteger a = BigInteger.ZERO;
BigInteger b = BigInteger.ONE;
BigInteger result = BigInteger.ZERO;
for (int i = 2; i <= n; i++) {
result = a.add(b);
a = b;
b = result;
}
return result;
}
public static void main(String[] args) {
int n = 100; // Example for larger value
System.out.println("Fibonacci number at position " + n + " is: " + fibonacci(n));
}
}
Why use BigInteger
?
- It can handle extremely large values without overflow.
- This is crucial for generating Fibonacci numbers, especially for indices greater than 93 where
long
might exceed its limits.
Mistake 4: Not Using Iterative Approaches
Many seem to gravitate towards recursion due to its elegance without considering the iterative approach, which is much more efficient for this particular problem.
Correct Implementation Example
public class Fibonacci {
public static int fibonacciIterative(int n) {
if (n < 0) throw new IllegalArgumentException("Input cannot be negative");
if (n <= 1) return n;
int a = 0, b = 1, result = 0;
for (int i = 2; i <= n; i++) {
result = a + b;
a = b;
b = result;
}
return result;
}
public static void main(String[] args) {
int n = 10;
System.out.println("Fibonacci number at position " + n + " is: " + fibonacciIterative(n));
}
}
Why choose an iterative approach?
- Iterative methods can often be more space-efficient.
- They avoid the overhead associated with recursive function calls.
- The time complexity remains O(n), similar to memoization.
To Wrap Things Up
Generating Fibonacci streams may seem intuitive, but the common mistakes outlined above can lead to inefficient and incorrect implementations. By recognizing these pitfalls and applying solutions such as memoization, proper data types, and iterative approaches, you can optimize your calculations effectively.
For further reading on the Fibonacci sequence and its applications, consider visiting Wikipedia's Fibonacci Number page.
Embrace these best practices, avoid the common traps, and make your Fibonacci implementations robust and efficient! Happy coding!