Decoding JDK8 Lambdas: A Glossary for Beginners
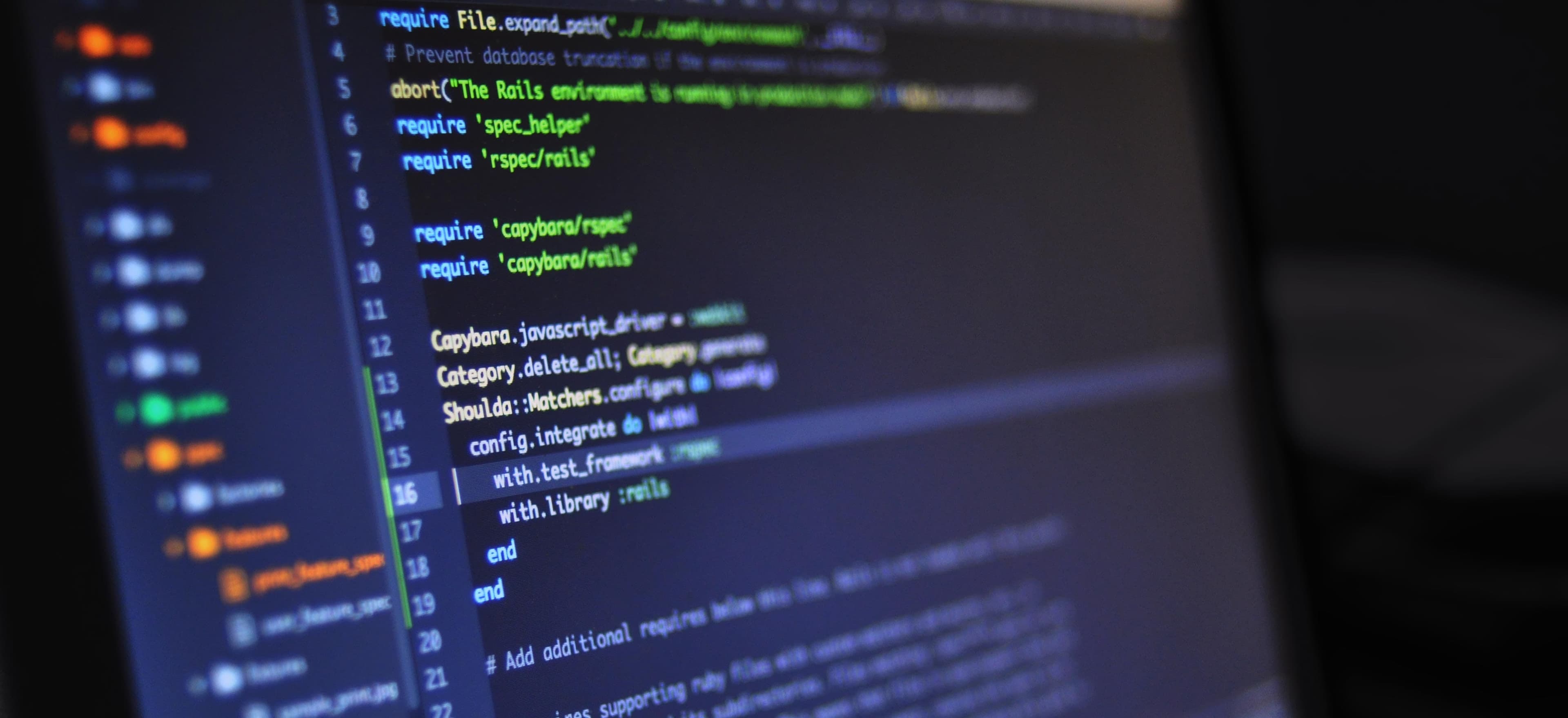
- Published on
Decoding JDK8 Lambdas: A Glossary for Beginners
If you are new to Java programming and have heard the term "lambda expressions" being thrown around, you might be wondering what they are and why they are important. In this article, we will delve into the world of JDK8 lambdas, breaking down the concept into bite-sized, digestible pieces for beginners to understand and appreciate.
What are JDK8 Lambdas?
In simple terms, lambda expressions in Java are a concise way to represent an anonymous function. They provide a clear and compact syntax for writing functions that can be passed around, just like any other object.
With the introduction of lambdas in JDK8, Java embraced a more functional style of programming, taking a significant step forward from traditional object-oriented paradigms.
Anatomy of a Lambda Expression
Let's start by dissecting a basic lambda expression to understand its structure:
(parameter) -> expression
In the above syntax, the lambda expression consists of the following parts:
- Parameter: Represents the input arguments to the function
- Arrow operator (->): Separates the parameter list from the body of the lambda expression
- Expression/Statement Block: Defines the behavior of the lambda function
The 'Why' Behind Lambda Expressions
Now that we have a basic understanding of the syntax, let's discuss why lambda expressions are a valuable addition to the Java language.
Conciseness and Readability
Traditional anonymous inner classes in Java often led to verbose and unwieldy code. Lambda expressions provide a more succinct and readable alternative, especially when dealing with functional interfaces.
Enhanced API
Lambdas significantly enhance the usability of Java's API by enabling a more expressive and streamlined way of working with collections, streams, and functional interfaces.
Parallel Processing
With the introduction of lambdas, Java's streams API gained the ability to perform parallel processing with ease, boosting performance in multi-core environments.
Functional Interfaces
To effectively use lambda expressions, it is crucial to understand the concept of functional interfaces. Simply put, a functional interface is an interface that contains exactly one abstract method. These interfaces serve as the target types for lambda expressions and method references.
Let's consider an example of a simple functional interface:
@FunctionalInterface
interface Calculator {
int calculate(int a, int b);
}
In the above example, the Calculator
interface is annotated with @FunctionalInterface
, indicating that it is intended to be used as a functional interface. It defines a single abstract method calculate
, which makes it a functional interface.
Putting Lambdas into Action
To illustrate the practical usage of lambda expressions, let's consider a scenario where we want to sort a list of strings in descending order of length using Comparator
.
Traditional approach using anonymous inner class:
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "David");
Collections.sort(names, new Comparator<String>() {
public int compare(String s1, String s2) {
return Integer.compare(s2.length(), s1.length());
}
});
By leveraging lambda expressions, the same operation can be achieved with greater brevity:
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "David");
names.sort((s1, s2) -> Integer.compare(s2.length(), s1.length()));
In this revised approach, the lambda expression (s1, s2) -> Integer.compare(s2.length(), s1.length())
serves as a concise representation of the compare
method in the Comparator
interface.
The Future of Java and Lambdas
As Java continues to evolve, the adoption of lambdas and functional programming concepts paves the way for more expressive, readable, and efficient code. Moreover, the introduction of features like method references further enriches the functional programming capabilities of Java.
In conclusion, JDK8 lambdas mark a significant leap forward for the Java language, empowering developers with tools to write more elegant and concise code. Embracing the power of functional programming, Java opens new avenues for exploring modern programming paradigms.
If you are eager to deepen your understanding of lambdas and functional programming in Java, exploring comprehensive resources like Oracle's official documentation and Baeldung's in-depth tutorials is highly recommended.
Stay curious, keep coding, and embrace the power of Java 8 lambdas!
Checkout our other articles