Skyrocket Your Java App: 10 Simple Speed Boosts!
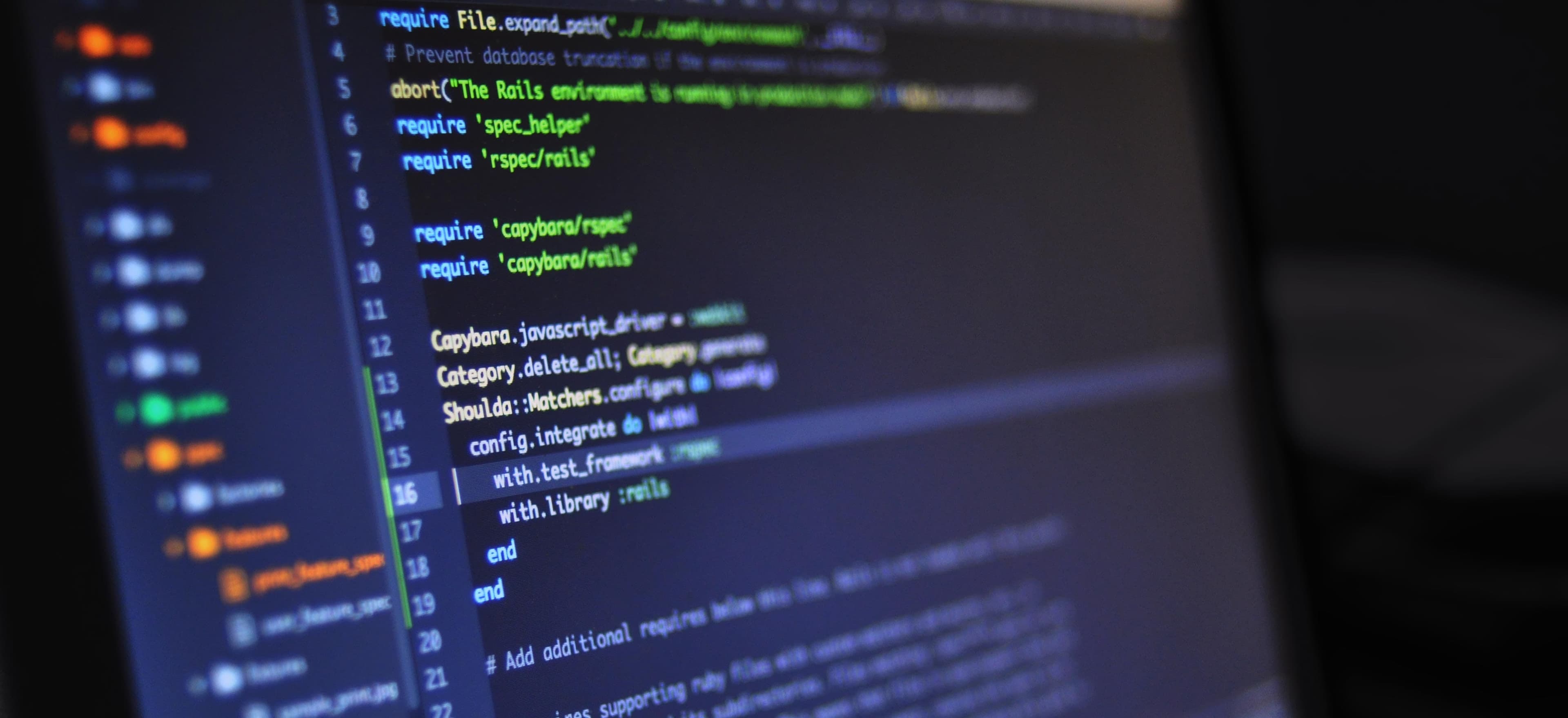
- Published on
10 Simple Ways to Boost the Speed of Your Java Application
When it comes to developing Java applications, speed is a critical factor. Users expect responsive and snappy applications, and any lag can result in a poor user experience. Fortunately, there are several techniques and best practices that you can employ to boost the speed of your Java application. In this post, we'll explore 10 simple and effective ways to supercharge the performance of your Java app.
1. Use StringBuilder for String Manipulation
String manipulation is a common operation in Java applications, and inefficient string concatenation can lead to performance bottlenecks. Instead of using the +
operator for concatenating strings, consider using the StringBuilder
class.
StringBuilder sb = new StringBuilder();
sb.append("This is");
sb.append(" a");
sb.append(" much faster");
sb.append(" approach");
String result = sb.toString();
Using StringBuilder
reduces the overhead of creating multiple intermediate String objects, resulting in improved performance for string manipulation operations.
2. Leverage Proper Collection Types
Choosing the right collection type can significantly impact the performance of your Java application. For example, when dealing with a large number of elements and frequent insertions or removals, LinkedList
can outperform ArrayList
. On the other hand, ArrayList
is more efficient for random access and iteration.
List<String> arrayList = new ArrayList<>();
List<String> linkedList = new LinkedList<>();
Understanding the characteristics and performance implications of different collection types will help you make informed decisions to optimize your application's performance.
3. Employ Effective Multithreading
Multithreading can enhance the responsiveness and overall speed of your Java application by utilizing the available hardware resources more effectively. However, improper handling of multithreading can lead to synchronization issues and performance degradation.
Using java.util.concurrent
utilities such as ExecutorService
and ForkJoinPool
can simplify multithreaded programming and improve the performance of parallel tasks.
ExecutorService executor = Executors.newFixedThreadPool(5);
executor.submit(() -> {
// Perform parallel task
});
executor.shutdown();
By leveraging multithreading effectively, you can harness the power of modern multi-core processors and accelerate your application's processing capabilities.
4. Optimize Database Interactions
Efficient database interactions are crucial for the overall performance of Java applications that rely on database access. Employing techniques such as batch processing, indexing, and using appropriate query optimizations can significantly reduce the database retrieval time and enhance the application's speed.
PreparedStatement statement = connection.prepareStatement("SELECT * FROM records WHERE id = ?");
statement.setInt(1, 1001);
ResultSet resultSet = statement.executeQuery();
// Process the resultSet
Carefully crafting efficient and optimized database queries can lead to substantial performance improvements in your Java application.
5. Utilize Effective Caching Strategies
Caching frequently accessed or computationally expensive data can dramatically improve the responsiveness of your Java application. Consider leveraging caching libraries such as Ehcache or Guava Cache to store and retrieve frequently accessed data from memory, reducing the need for repetitive computations or database access.
LoadingCache<Key, Graph> graphs = CacheBuilder.newBuilder()
.maximumSize(1000)
.expireAfterWrite(10, TimeUnit.MINUTES)
.build(
new CacheLoader<Key, Graph>() {
public Graph load(Key key) throws AnyException {
return createExpensiveGraph(key);
}
});
By implementing effective caching strategies, you can minimize latency and enhance the overall performance of your Java application.
6. Profile and Optimize Code
Profiling your Java application using tools like JVisualVM, YourKit, or JProfiler can help identify performance bottlene and resource-intensive areas of your code. Once you've identified the bottlenecks, you can focus on optimizing those specific segments to achieve significant performance gains.
// Profiled code segment
Optimizations can include algorithmic improvements, memory management enhancements, and refactoring to eliminate redundant operations, leading to a faster and more efficient application.
7. Employ Effective Memory Management
Efficient memory management is crucial for optimizing the performance of Java applications. Avoiding memory leaks, minimizing object creation, and utilizing appropriate data structures can help in efficient memory allocation and deallocation, leading to improved application speed.
Using tools like JVisualVM or Java Mission Control can aid in monitoring memory usage and identifying areas where memory optimizations are necessary.
// Memory-efficient code segment
Effective memory management can significantly contribute to the overall speed and responsiveness of your Java application.
8. Utilize Just-In-Time (JIT) Compilation
Java's JIT compiler converts bytecode into native machine code at runtime, optimizing the performance of the application. By default, the JIT compiler kicks in after a certain threshold of interpreted executions.
You can influence the behavior of the JIT compiler by tuning options such as -XX:CompileThreshold
and -XX:+AggressiveOpts
based on the specific workload and performance requirements of your application.
java -XX:CompileThreshold=1000 -XX:+AggressiveOpts YourApp
Fine-tuning JIT compilation can lead to improved runtime performance and reduced startup times for your Java application.
9. Optimize I/O Operations
Efficient I/O operations are crucial for the overall performance of Java applications, especially those involving file handling, network communication, or input/output streams.
Utilizing NIO (New I/O) and asynchronous I/O can significantly enhance the throughput and responsiveness of your application by minimizing blocking and leveraging non-blocking I/O operations.
// NIO code segment
By optimizing I/O operations, you can mitigate latency issues and boost the overall speed of your Java application.
10. Maintain Code Consistency and Simplicity
Maintaining clean, concise, and consistent code can indirectly contribute to the performance of your Java application. Well-structured and easily understandable code can facilitate faster debugging, maintenance, and refactoring, leading to better overall performance in the long run.
Adhering to established coding conventions, employing meaningful variable names, and avoiding unnecessary complexity can all contribute to the readability and maintainability of your codebase.
// Well-structured and consistent code snippet
By prioritizing code consistency and simplicity, you can indirectly support the performance and agility of your Java application in the long term.
Final Thoughts
Optimizing the speed of your Java application involves a combination of efficient coding practices, smart resource management, and a thorough understanding of performance optimization techniques. By implementing the 10 simple speed boosts discussed in this post, you can enhance the responsiveness and efficiency of your Java application, delivering a better user experience and gaining a competitive edge in the market.
Embrace these speed-boosting strategies and propel your Java application to new performance heights!
Checkout our other articles