Mastering Groovy: Overcoming Operator Overload Confusion
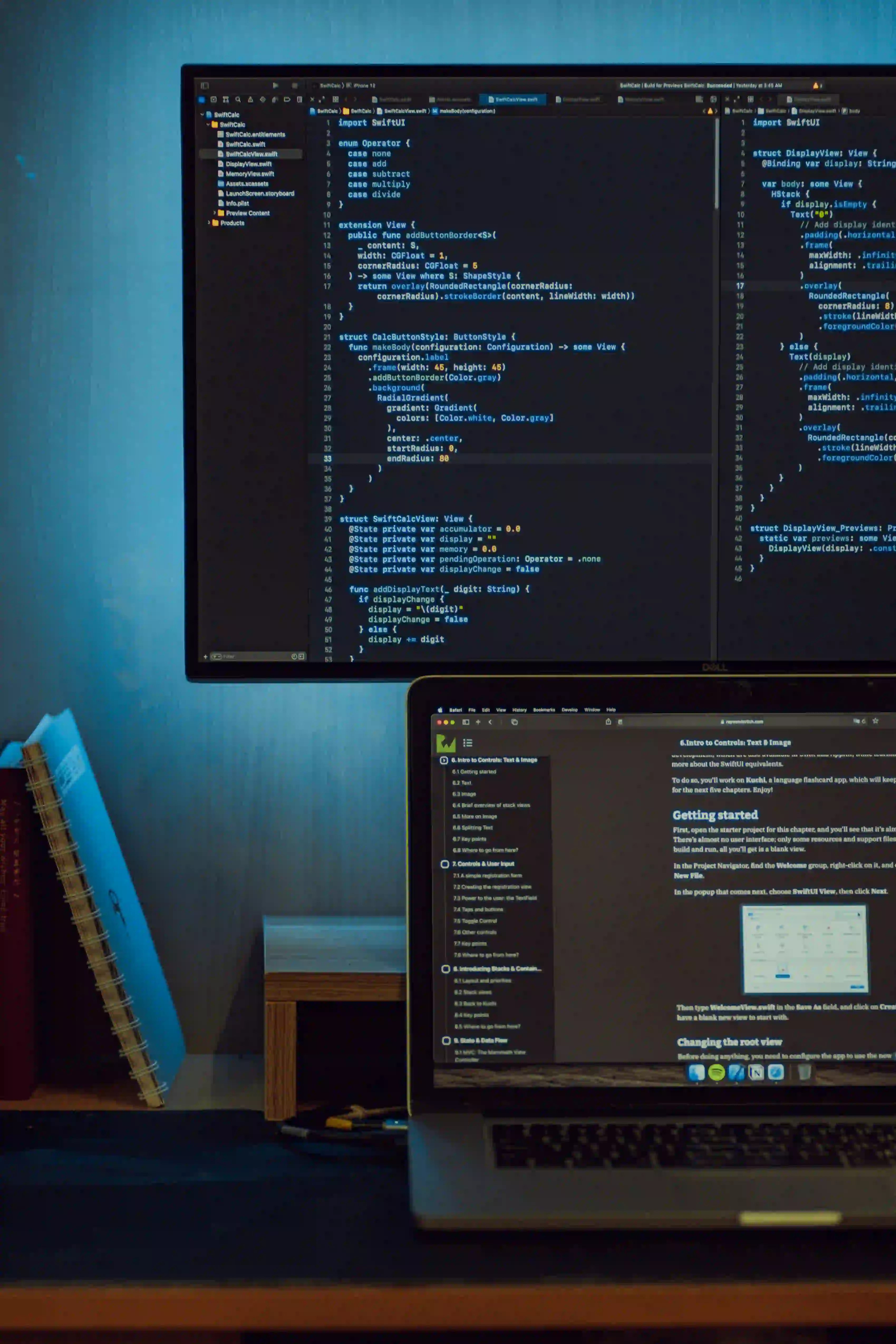
Mastering Groovy: Overcoming Operator Overload Confusion
As a Java developer, it's crucial to have a solid understanding of Groovy, which is a powerful, dynamic language that runs on the Java Virtual Machine (JVM). One of the unique features of Groovy is operator overloading, which allows you to define custom behaviors for operators such as +
, -
, *
, and /
. While operator overloading can greatly enhance the expressiveness of your code, it can also lead to confusion if not used carefully. In this blog post, we'll dive into the world of Groovy operator overloading, discuss best practices, and provide examples to help you master this powerful feature.
What is Operator Overloading?
Operator overloading is the ability to redefine the behavior of built-in operators in a programming language. In Groovy, you can overload operators by defining special methods with predefined names. For example, to overload the +
operator for a custom class, you can define a method named plus
:
class ComplexNumber {
double real
double imaginary
ComplexNumber plus(ComplexNumber other) {
new ComplexNumber(real + other.real, imaginary + other.imaginary)
}
}
In this example, when you use the +
operator with two ComplexNumber
instances, Groovy will call the plus
method defined in the ComplexNumber
class.
Best Practices for Operator Overloading
Before we delve into advanced examples, let's discuss some best practices for using operator overloading in Groovy:
-
Use Overloading Sparingly: While operator overloading can be a powerful tool, it should be used sparingly and judiciously. Overusing operator overloading can lead to code that is difficult to understand and maintain.
-
Follow Conventions: Groovy has established naming conventions for overloaded operators, such as
plus
for the+
operator,minus
for the-
operator, and so on. It's essential to follow these conventions to make your code more readable and predictable for other developers. -
Keep it Intuitive: When overloading operators, strive to maintain intuitive behavior. The overloaded operators should mimic the behavior of their built-in counterparts as closely as possible to avoid surprising behavior.
Now, let's explore some practical examples to solidify our understanding of Groovy operator overloading.
Example 1: Overloading the Plus Operator
In this example, we'll demonstrate how to overload the +
operator for a custom Vector
class. When you add two Vector
instances, we want to calculate the vector sum of their components.
class Vector {
double x
double y
Vector plus(Vector other) {
new Vector(x + other.x, y + other.y)
}
}
def v1 = new Vector(x: 3, y: 4)
def v2 = new Vector(x: 1, y: 2)
def v3 = v1 + v2
println "Vector Sum: (${v3.x}, ${v3.y})"
In this example, we've defined the plus
method in the Vector
class to perform the vector addition when the +
operator is used with two Vector
instances. This allows for a more natural and expressive syntax when working with vectors.
Example 2: Overloading the Multiply Operator
Now, let's explore how to overload the *
operator for matrix multiplication. We'll create a Matrix
class and define the multiply
method to perform matrix multiplication when the *
operator is used.
class Matrix {
int rows
int columns
int[][] data
Matrix(int rows, int columns, int[][] data) {
this.rows = rows
this.columns = columns
this.data = data
}
Matrix multiply(Matrix other) {
// Perform matrix multiplication
// ...
}
}
def m1 = new Matrix(2, 2, [[1, 2], [3, 4]])
def m2 = new Matrix(2, 2, [[5, 6], [7, 8]])
def result = m1 * m2
In this example, we've defined the multiply
method in the Matrix
class to handle matrix multiplication when the *
operator is used with two Matrix
instances. This makes the code more expressive and readable when working with matrices.
Example 3: Overloading the Comparison Operators
Groovy also allows you to overload the comparison operators (<
, <=
, >
, >=
, ==
, !=
) for custom classes. Let's consider a Date
class and define the compareTo
method to enable date comparisons.
class Date implements Comparable<Date> {
int year
int month
int day
int compareTo(Date other) {
// Implement comparison logic
// ...
}
boolean equals(Object obj) {
// Implement equality logic
// ...
}
}
def today = new Date(year: 2022, month: 10, day: 20)
def tomorrow = new Date(year: 2022, month: 10, day: 21)
if (tomorrow > today) {
println "Tomorrow comes after today"
}
In this example, we've implemented the compareTo
method to enable date comparisons using the comparison operators. Additionally, we've overridden the equals
method to provide custom equality logic for Date
instances.
My Closing Thoughts on the Matter
Operator overloading in Groovy is a powerful feature that can enhance the expressiveness and readability of your code. However, it should be used judiciously and with careful consideration for maintaining intuitive behavior. By following best practices and using operator overloading sparingly, you can leverage this feature to write elegant and concise code in Groovy.
In this blog post, we've explored practical examples of overloading the +
, *
, and comparison operators in Groovy, demonstrating how operator overloading can be used to create more expressive and intuitive code. By mastering operator overloading, you can take your Groovy programming skills to the next level and unlock the full potential of this dynamic language.
Remember, while operator overloading can be a useful tool, it should always serve to enhance the clarity and maintainability of your code. With practice and a solid understanding of the underlying principles, you can confidently wield the power of operator overloading in Groovy.
So go ahead, experiment with operator overloading in Groovy, and unleash the full potential of this dynamic language!
Learn more about operator overloading in Groovy documentation.