Unlock Java Powers: Mastering Custom Serialization with Externalizable!
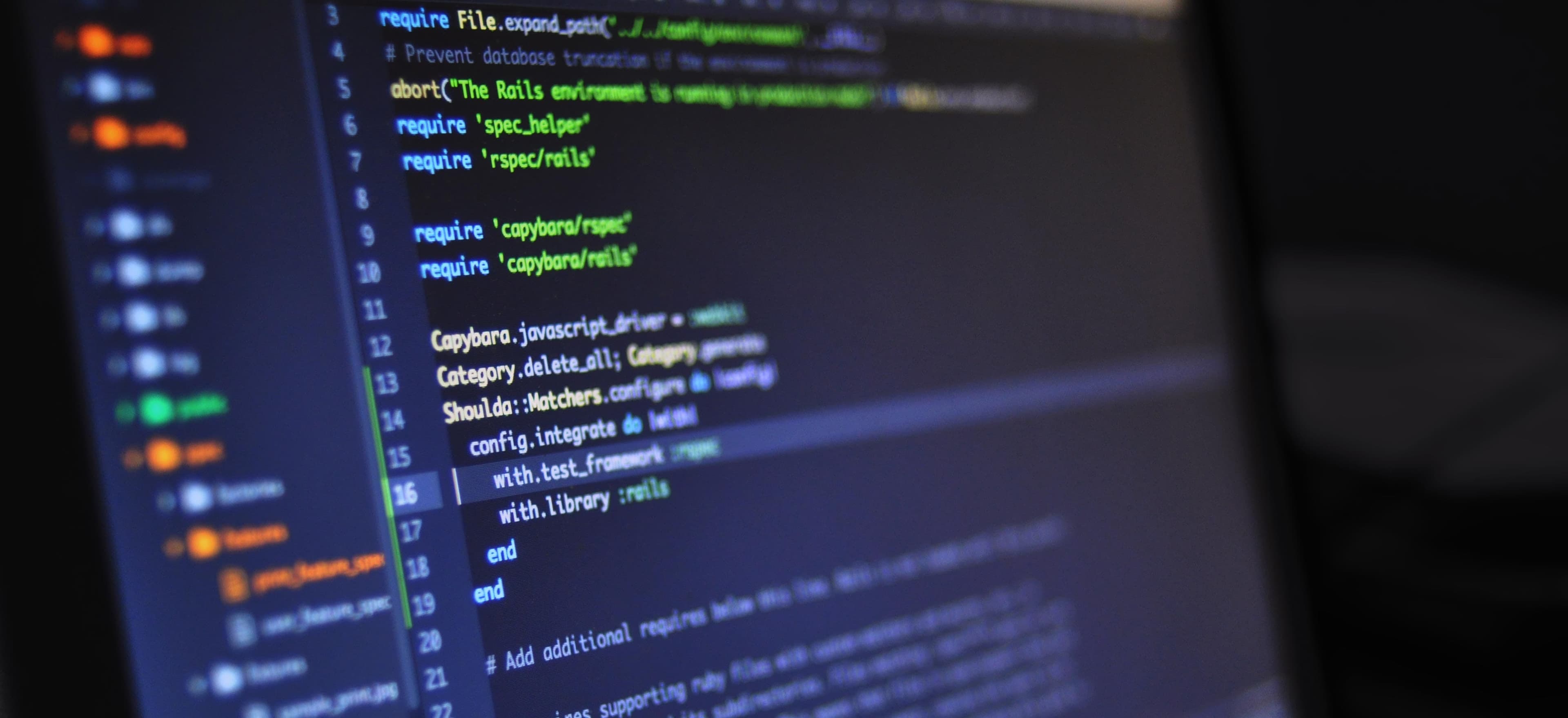
- Published on
Unlock Java Powers: Mastering Custom Serialization with Externalizable
Serialization is a powerful tool in Java that allows you to convert objects into byte streams for storage or transmission, and then reconstruct them at a later time. While Java provides default serialization through the Serializable
interface, it also allows for custom serialization using the Externalizable
interface. This custom approach gives you more control over the serialization process and can lead to improved performance and storage efficiency.
In this post, we will dive into the world of custom serialization with the Externalizable
interface in Java. We will explore its usage, benefits, and best practices, and provide code examples to demonstrate its implementation.
Understanding the Externalizable Interface
The Externalizable
interface in Java is used to create custom serialization for an object. Unlike the Serializable
interface, which automatically handles serialization and deserialization, the Externalizable
interface requires you to explicitly implement the serialization and deserialization process for your objects.
By implementing the Externalizable
interface, you gain control over how your objects are serialized and deserialized, allowing you to optimize the process for better performance and more efficient object storage.
Implementing the Externalizable Interface
To implement the Externalizable
interface, a class must provide implementations for the writeExternal()
and readExternal()
methods. These methods define the custom serialization and deserialization logic for the object, respectively.
Let's take a look at an example to understand the implementation of the Externalizable
interface:
import java.io.Externalizable;
import java.io.ObjectInput;
import java.io.ObjectOutput;
import java.io.IOException;
public class CustomObject implements Externalizable {
private int id;
private String name;
// Default constructor (mandatory for Externalizable)
public CustomObject() {}
// Parameterized constructor
public CustomObject(int id, String name) {
this.id = id;
this.name = name;
}
@Override
public void writeExternal(ObjectOutput out) throws IOException {
out.writeInt(id);
out.writeUTF(name);
}
@Override
public void readExternal(ObjectInput in) throws IOException, ClassNotFoundException {
id = in.readInt();
name = in.readUTF();
}
}
In this example, the CustomObject
class implements the Externalizable
interface and provides custom serialization and deserialization logic in the writeExternal()
and readExternal()
methods, respectively. Inside the writeExternal()
method, the object's fields are explicitly written to the ObjectOutput
stream, and in the readExternal()
method, the fields are read from the ObjectInput
stream to reconstruct the object.
Benefits of Using Externalizable
Implementing custom serialization with the Externalizable
interface offers several benefits:
-
Performance Optimization: By defining custom serialization and deserialization logic, you can optimize the process to better suit the structure and data of your objects, potentially leading to improved performance compared to default serialization.
-
Storage Efficiency: Custom serialization allows you to control which fields are serialized and in what format, potentially reducing the size of the serialized object and improving storage efficiency.
-
Versioning Control: With custom serialization, you have more control over the serialization format, making it easier to handle versioning and backward compatibility of serialized objects.
Best Practices for Using Externalizable
While custom serialization with the Externalizable
interface offers great flexibility, it comes with the responsibility of handling the entire serialization process. Here are some best practices to keep in mind:
-
Handle Class Evolution: Take into account the evolution of your object's class when designing the serialization format. Be mindful of adding/removing fields and changing their types, and implement proper handling to maintain compatibility with previously serialized objects.
-
Performance Testing: Measure the performance impact of custom serialization in your specific use case. While it can often lead to performance improvements, it's important to validate this through testing and benchmarking.
-
Security Considerations: When implementing custom serialization, ensure that you handle sensitive data appropriately to avoid security vulnerabilities.
Closing Remarks
Custom serialization with the Externalizable
interface in Java empowers you to take control of the serialization and deserialization process for your objects, leading to potential improvements in performance, storage efficiency, and versioning control. By implementing the Externalizable
interface and following best practices, you can tailor the serialization process to suit the specific requirements of your application.
Incorporating the Externalizable
interface into your Java application's serialization strategy can unlock new possibilities and efficiencies, making it an essential tool for any Java developer's arsenal.
Now that you have a solid understanding of custom serialization with Externalizable, it's time to explore further and deepen your skills. Check out the official Oracle documentation for more in-depth details on the Externalizable interface.
Happy coding!
Checkout our other articles