Mastering Functional Style in Programming: A Guide
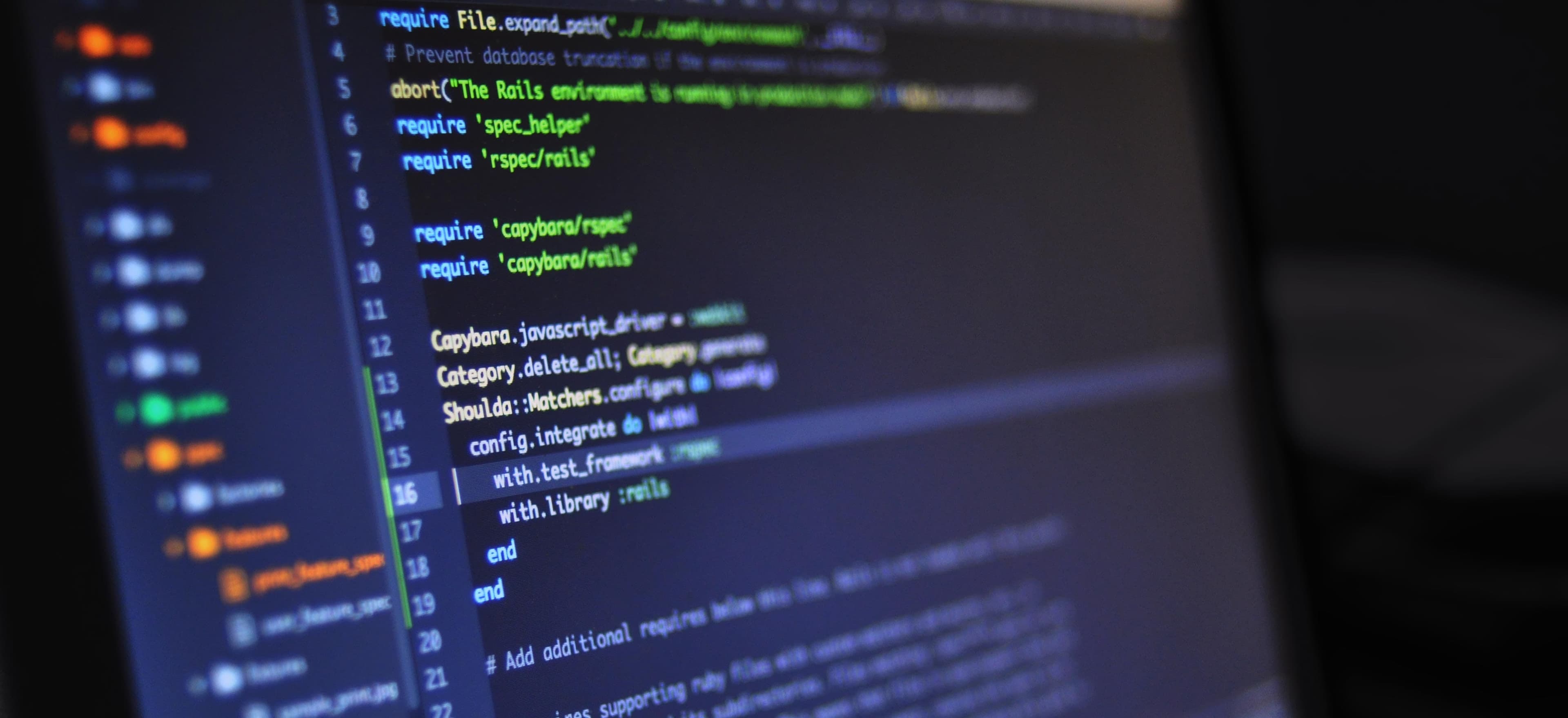
- Published on
Mastering Functional Style in Programming: A Guide
Functional programming has become increasingly popular in recent years due to its focus on clarity, maintainability, and the ability to handle complex operations. Java, traditionally an object-oriented language, has also embraced functional programming paradigms through the introduction of features such as lambda expressions and the Stream API. In this guide, we will explore the principles of functional programming and how to apply them effectively in Java.
Understanding Functional Programming
Functional programming is a programming paradigm that treats computation as the evaluation of mathematical functions and avoids changing-state and mutable data. It emphasizes the use of functions that produce consistent and predictable results without modifying shared state. Key concepts include:
-
Immutability: Data is immutable, meaning it cannot be changed after it's created. This reduces the chance of unexpected side effects.
-
Pure Functions: Functions that always produce the same output for the same input and have no side effects. They do not rely on external state.
-
First-Class Functions: Treating functions as first-class citizens, allowing functions to be passed as arguments, returned from other functions, and assigned to variables.
Functional Concepts in Java
Lambda Expressions
Lambda expressions, introduced in Java 8, allow functions to be passed around as values. They enable a more functional style of coding by allowing the representation of a function as an expression.
List<String> names = Arrays.asList("John", "Sarah", "Mark");
names.removeIf(name -> name.length() > 5);
In this example, the removeIf
method takes a predicate, which is written as a lambda expression. It removes elements from the list if the provided condition is true.
Stream API
The Stream API provides a way to process collections of objects in a functional manner. It allows for declarative processing of elements and supports operations such as map
, filter
, and reduce
.
List<String> languages = Arrays.asList("Java", "Python", "JavaScript");
long count = languages.stream()
.filter(lang -> lang.length() > 4)
.count();
Here, the stream
method converts the collection into a stream, and the filter
method uses a lambda expression to define the filtering criteria. The count
method then returns the number of elements that match the criteria.
Optional
The Optional
class was introduced to deal with potential null pointer exceptions. It encourages the use of functional principles by forcing developers to consider a value being absent.
Optional<String> name = Optional.ofNullable(getName());
String result = name.orElse("Default");
In this example, if the name
is not present, the orElse
method returns the default value, thus avoiding a null pointer exception.
Advantages of Functional Programming in Java
-
Conciseness: Functional programming encourages writing compact, concise code, reducing the amount of boilerplate and ceremony compared to traditional Java.
-
Readability: The emphasis on immutability and pure functions leads to code that is easier to reason about and understand.
-
Parallelism: Functional programming facilitates easier parallel processing, as pure functions with no side effects are inherently thread-safe.
-
Testability: Code written in a functional style tends to be easier to test, as functions are isolated and deterministic.
Applying Functional Style in Java
Immutability
In Java, you can achieve immutability by using final
keyword for variables, and creating immutable objects by ensuring that their state cannot be modified after construction.
final int x = 10;
// x = 20; // Compilation error - cannot reassign a final variable
Pure Functions
To create pure functions in Java, ensure that functions do not have any side effects and always produce the same output for the same input. Avoid modifying state outside the function’s scope.
public int square(int x) {
return x * x;
}
The square
method here is a pure function as it returns the same output for the same input.
Function Composition
Functional composition involves combining simple functions to build more complex ones. In Java, this can be achieved using method references or by creating new functions through composition.
Function<Integer, Integer> increment = x -> x + 1;
Function<Integer, Integer> doubleIt = x -> x * 2;
Function<Integer, Integer> incrementAndDouble = increment.andThen(doubleIt);
int result = incrementAndDouble.apply(5); // Result: 12
Here, we compose increment
and doubleIt
functions to create a new function that increments the input and then doubles the result.
Avoiding Mutability
When working with collections in Java, prefer using immutable collections from the java.util.Collections
class or using the Collectors
utility class in the Stream API.
List<String> immutableList = Collections.unmodifiableList(Arrays.asList("Apple", "Banana", "Orange"));
Here, the unmodifiableList
method returns an immutable list, preventing any modifications to the list.
The Bottom Line
In this guide, we have explored the principles of functional programming and how to apply them effectively in Java. By embracing functional style in Java, developers can write code that is concise, readable, and easier to test. With the introduction of features such as lambda expressions, the Stream API, and the Optional
class, Java has become more aligned with functional programming paradigms, allowing developers to leverage the benefits of this approach in their code.
To master functional programming in Java, it is important to practice writing code in a functional style, focusing on immutability, pure functions, and functional composition. By doing so, developers can build robust and maintainable applications that take full advantage of the functional programming paradigm.
Start incorporating functional programming concepts into your Java projects and experience the benefits firsthand. Happy coding!
For further reading, check out:
- Java Functional Programming: A Complete Guide
- Effective Java Streams: Best Practices and Tips
Remember, the journey to mastering functional programming in Java is a continuous learning process, so keep exploring and experimenting with these powerful concepts!