Mapping WCF to Java: Navigating the Compatibility Maze
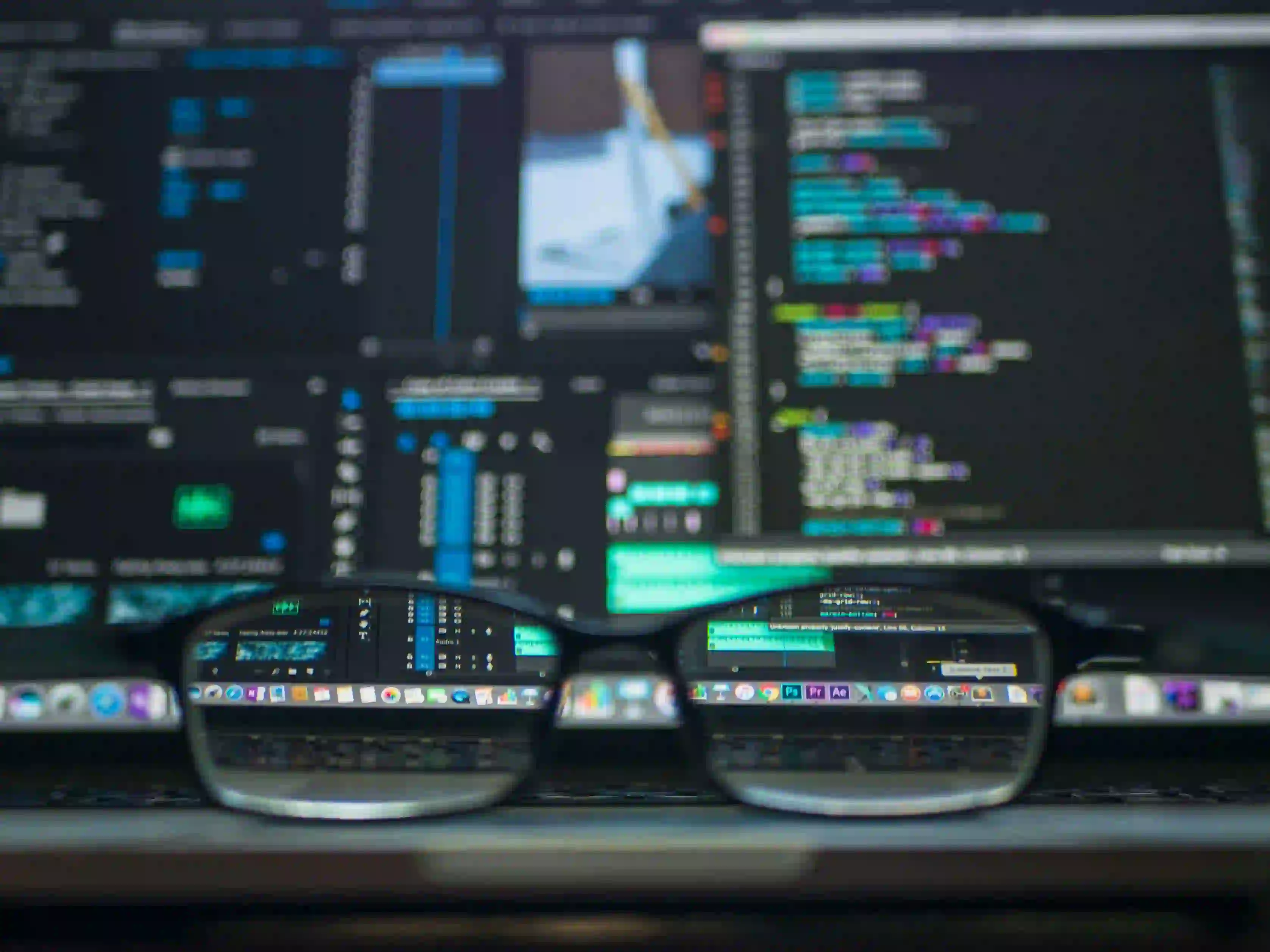
Mapping WCF to Java: Navigating the Compatibility Maze
When it comes to building enterprise-level applications, Java and WCF (Windows Communication Foundation) are two popular platforms that developers often choose. However, integrating these technologies can present challenges due to their inherent differences. This post will guide you through the process of mapping WCF concepts to their Java equivalents, helping you navigate the compatibility maze.
Understanding WCF
Windows Communication Foundation, a part of the .NET framework, provides a platform for building secure, reliable, and transacted web services. It supports various communication protocols and message formats, making it a versatile choice for developing distributed systems on the Windows platform.
WCF Concepts and Their Java Equivalents
Service Contracts and Interfaces
In WCF, service contracts define the operations that a service exposes to clients. These contracts are defined using interfaces annotated with attributes to specify their behavior. When working with Java, the equivalent concept is defining a service interface using annotations such as @WebService
to mark it as a web service endpoint.
@WebService
public interface MyService {
@WebMethod
String performOperation();
}
This Java code snippet showcases the use of @WebService
and @WebMethod
annotations to declare a service interface and its operations, mirroring the functionality of WCF service contracts.
Data Contracts and JavaBeans
In WCF, data contracts represent the types that are exchanged between the service and the client. These are defined using Plain Old CLR Objects (POCO) and are annotated with attributes to fine-tune serialization behavior. Java's equivalent to data contracts is creating JavaBeans, which are plain Java classes with private fields, public getters and setters, and optional annotations for serialization control.
public class MyData {
private String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
In this Java example, MyData
class is a JavaBean with a private field and corresponding getters and setters, aligning with the principles of WCF data contracts.
Bindings and Endpoints
WCF uses bindings to define the communication protocols, encoding formats, and transport protocols for services. Endpoints are then configured with a specific binding to expose the service and specify its address. In Java, the equivalent concept involves using frameworks like JAX-WS or Apache CXF to define and configure endpoints with specific bindings and transport options.
@WebService
public class MyServiceEndpoint {
public MyServiceEndpoint() {
// Configure binding and endpoint
}
}
This Java class depicts the configuration of a service endpoint using JAX-WS, where the binding and endpoint details can be set up programmatically or through annotations.
Hosting the Service
In WCF, services are typically hosted in IIS (Internet Information Services), Windows Services, or self-hosted environments. Java services can be hosted using servlet containers like Apache Tomcat or web servers like Jetty. Additionally, Java EE application servers such as WildFly and GlassFish provide robust environments for hosting enterprise-level Java services.
Bridging the Gap: Interoperability Challenges
While understanding the equivalents between WCF and Java is crucial, bridging the gap and ensuring interoperability brings its own set of challenges. One common challenge involves handling differences in message format and serialization between WCF and Java web services. WCF often uses SOAP (Simple Object Access Protocol) with XML-based message formats, whereas Java web services support both SOAP and REST with JSON and XML message formats.
To address this challenge, tools like Apache CXF and Metro provide support for integrating Java with SOAP-based WCF services. These tools offer features to handle message format conversions, allowing Java clients to communicate seamlessly with WCF services.
Another interoperability challenge arises from differences in security features and protocols between WCF and Java. WCF supports Windows-integrated security mechanisms and WS-Security standards, while Java provides support for various security protocols such as SSL, OAuth, and SAML. Bridging this gap requires careful consideration of the security requirements and the use of compatible security protocols and mechanisms on both the WCF and Java sides.
Final Thoughts
Integrating WCF with Java involves understanding the mapping of WCF concepts to their Java equivalents and addressing interoperability challenges. By leveraging the right tools, frameworks, and best practices, developers can bridge the compatibility gap and build robust, interoperable solutions that harness the strengths of both platforms.
In conclusion, mapping WCF to Java is a complex but essential task for organizations looking to integrate disparate systems and technologies. By understanding the fundamental concepts and challenges, developers can navigate the compatibility maze and create seamless integration solutions that empower their enterprise applications.
Remember, understanding the nuances of WCF and Java integration is an ongoing process, but with the right knowledge and tools, it's a challenge that can be conquered.
To further explore the capabilities of Java in seamlessly integrating with other platforms, check out this comprehensive guide on Java interop and expand your understanding of cross-platform development.
Ready to dive deeper into WCF? Learn more about its features and use cases in this insightful article on WCF in the enterprise.