Overcoming JSON Parsing Challenges in Akka HTTP Apps
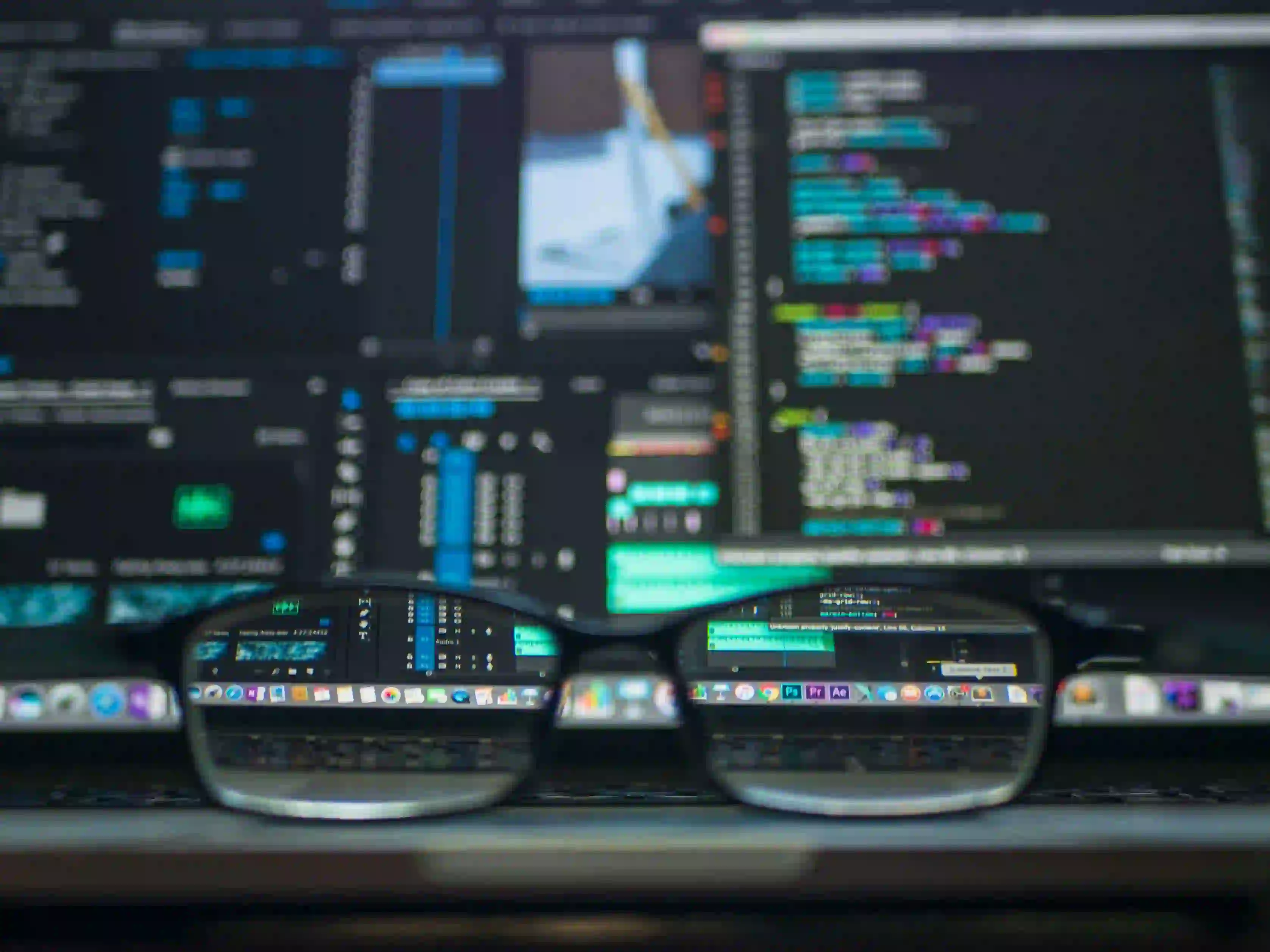
Overcoming JSON Parsing Challenges in Akka HTTP Apps
When developing web applications in Java, Akka HTTP is a prominent choice due to its powerful capabilities for building RESTful APIs and handling HTTP requests. While Akka HTTP simplifies many aspects of web app development, working with JSON data can pose certain challenges. In this article, we will explore some common JSON parsing challenges in Akka HTTP apps and demonstrate effective strategies to overcome them.
Understanding JSON Parsing in Akka HTTP
JSON (JavaScript Object Notation) is a widely used format for exchanging data between a server and a client. In the context of Akka HTTP, JSON parsing involves serializing and deserializing JSON data to and from Java objects. This process is crucial for effectively transmitting and processing data in web applications.
Akka HTTP provides support for JSON parsing through libraries such as Jackson, Spray JSON, and others. These libraries offer convenient APIs for converting JSON data to Java objects and vice versa. While these libraries simplify the parsing process, developers often encounter challenges related to handling JSON data in Akka HTTP apps.
Common JSON Parsing Challenges
1. Different JSON Libraries
When working on a project with multiple contributors, each developer may have their preferred JSON parsing library. This can lead to inconsistency and potential conflicts within the codebase. It's essential to standardize the JSON parsing approach to ensure uniformity and maintainability.
2. Nested JSON Structure
Dealing with nested JSON structures can be complex, especially when mapping them to Java objects. Ensuring that the structure of the JSON data aligns with the Java object model requires careful attention to detail.
3. Error Handling
Effective error handling during JSON parsing is crucial for maintaining the stability and reliability of the application. Handling exceptions and providing meaningful error messages to the client are essential aspects of JSON parsing in Akka HTTP.
Strategies for Overcoming JSON Parsing Challenges
1. Standardize JSON Library Usage
To address the challenge of different JSON libraries, it's beneficial to standardize the usage of a specific library across the project. Jackson, a popular JSON processing library for Java, is widely compatible with Akka HTTP and offers comprehensive features for JSON parsing. By standardizing the use of Jackson within the project, developers can ensure consistency and mitigate potential conflicts arising from the use of multiple libraries.
Here's an example of using Jackson for JSON parsing in Akka HTTP:
// Define a case class representing the JSON data
public class User {
public String name;
public int age;
}
// Deserialize JSON to Java object using Jackson
ObjectMapper objectMapper = new ObjectMapper();
User user = objectMapper.readValue(jsonString, User.class);
In this example, the ObjectMapper class from Jackson is used to deserialize JSON data into a Java object.
2. Handling Nested JSON Structure
When dealing with nested JSON structures, it's essential to carefully design the corresponding Java object model to reflect the hierarchy of the JSON data. Utilizing annotations provided by the chosen JSON processing library, such as Jackson's @JsonProperty
and @JsonIgnore
, can help in mapping nested JSON attributes to Java object properties.
Here's an example of mapping nested JSON structure to Java objects using Jackson annotations:
public class Address {
@JsonProperty("street")
public String street;
@JsonProperty("city")
public String city;
}
public class User {
public String name;
public int age;
@JsonProperty("address")
public Address address;
}
In this example, the @JsonProperty
annotation is used to map JSON attributes to Java object properties, facilitating the handling of nested JSON structures.
3. Error Handling in JSON Parsing
Effective error handling during JSON parsing can be achieved by leveraging Akka HTTP's directives for handling exceptions and providing meaningful responses to clients. By utilizing directives such as handleExceptions
and complete
, developers can ensure robust error handling in JSON parsing scenarios.
Here's an example of error handling during JSON parsing in Akka HTTP:
path("parseJson") {
post {
entity(as[JsValue]) { json =>
val result = Try(json.convertTo[User])
result match {
case Success(user) => complete(s"Parsed user: $user")
case Failure(ex) => complete(BadRequest, s"Failed to parse JSON: ${ex.getMessage}")
}
}
}
}
In this example, the Akka HTTP directive complete
is used to provide a response based on the result of JSON parsing, effectively handling errors and communicating the outcome to the client.
To Wrap Things Up
In Akka HTTP applications, effective JSON parsing is essential for seamless data exchange and processing. By standardizing JSON library usage, carefully handling nested JSON structures, and implementing robust error handling mechanisms, developers can effectively overcome JSON parsing challenges in Akka HTTP apps. Embracing best practices and leveraging the capabilities of libraries such as Jackson can significantly streamline the JSON parsing process, leading to more robust and maintainable web applications.
Mastering JSON parsing in Akka HTTP is an important aspect of web development, and with the right strategies and tools, developers can successfully navigate and overcome the challenges associated with parsing JSON data in their applications.
For further in-depth understanding of Akka HTTP and JSON parsing, the official Akka documentation provides comprehensive resources and examples.
In conclusion, mastering JSON parsing in Akka HTTP is crucial for building robust web applications, and by standardizing JSON library usage, managing nested JSON structures, and implementing solid error handling, developers can effectively address and overcome the challenges associated with JSON parsing in Akka HTTP apps.