Cracking the Code: Spring's Constructor Injection Mystery
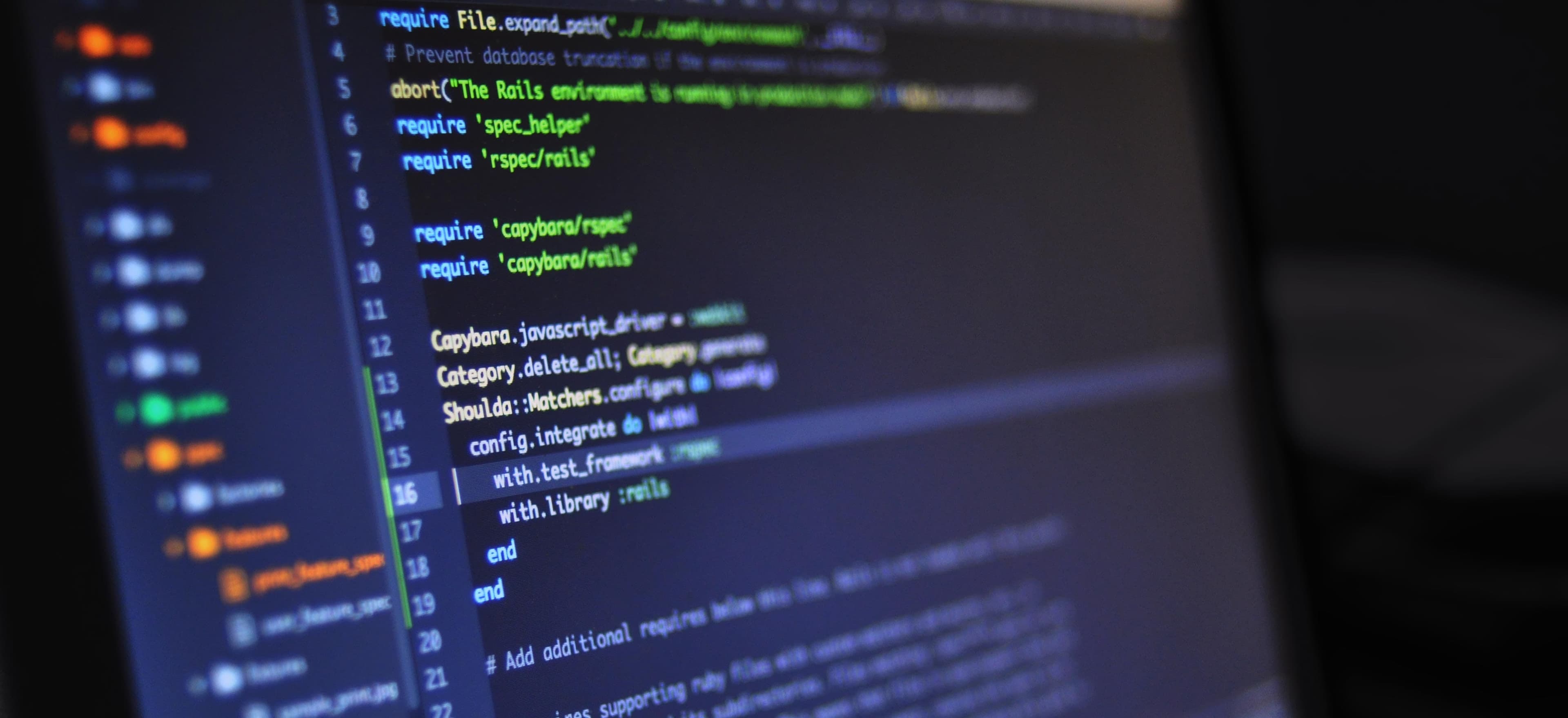
- Published on
Cracking the Code: Spring's Constructor Injection Mystery
When it comes to dependency injection in the world of Java development, Spring framework stands as a powerful and popular choice for managing dependencies. One of the key aspects of dependency injection in Spring is constructor injection, which provides numerous benefits for managing dependencies in a clear and concise manner. In this post, we will unravel the mystery of constructor injection in Spring, its advantages, and how to effectively utilize it in your Java applications.
Understanding Constructor Injection
Constructor injection is a method of providing dependencies to a class by passing them through the constructor. This is in contrast to setter injection, where dependencies are set using setter methods. In Spring, constructor injection allows you to declare the dependencies of a class in its constructor, enabling the Spring container to create the instances and inject the necessary dependencies when the bean is initialized.
Why Constructor Injection?
Constructor injection offers several advantages over other forms of dependency injection, including:
-
Immutability: When dependencies are injected via the constructor, the class is instantiated with all its dependencies, making it immutable after creation. This promotes a more robust and thread-safe codebase.
-
Clarity: Constructor injection makes the dependencies of a class explicit and enforces them at the time of instantiation, leading to clearer and self-documenting code.
-
Forced Dependency: With constructor injection, you can ensure that all mandatory dependencies are provided at the time of object creation, reducing the risk of null or uninitialized dependencies.
-
Testing: Constructor-injected dependencies are easier to mock and test, as they are passed in during object creation.
Implementing Constructor Injection in Spring
To demonstrate constructor injection in a Spring application, let's consider a simple scenario where we have a UserService
class that depends on a UserRepository
to fetch user data from a database.
public class UserService {
private final UserRepository userRepository;
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
// Other methods and business logic
}
In this example, the UserService
class declares a constructor that takes a UserRepository
as a parameter. The UserRepository
dependency is then assigned to a final field within the class.
Inversion of Control (IoC) and Constructor Injection
In Spring, the process of constructor injection is facilitated by the Inversion of Control (IoC) container, which is responsible for managing the instantiation and injection of dependencies. By defining the dependencies through constructor parameters, the IoC container can resolve and inject them when creating instances of the respective classes.
Configuring Constructor Injection in Spring
To configure constructor injection in a Spring application, you can utilize XML-based or annotation-based configuration.
XML-based Configuration
In the XML configuration file, you can define the beans and their dependencies using constructor-arg elements within the bean definitions.
<bean id="userService" class="com.example.UserService">
<constructor-arg ref="userRepository"/>
</bean>
<bean id="userRepository" class="com.example.UserRepository"/>
In this setup, the UserService
bean is configured with a constructor argument referencing the userRepository
bean. When the UserService
bean is initialized, the IoC container injects the userRepository
bean into its constructor.
Annotation-based Configuration
With annotation-based configuration, you can use the @Autowired
annotation on the constructor, eliminating the need for explicit XML configuration.
@Service
public class UserService {
private final UserRepository userRepository;
@Autowired
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
// Other methods and business logic
}
In this case, the @Autowired
annotation on the constructor instructs the Spring framework to perform constructor injection for the UserService
bean, resolving the UserRepository
dependency.
Best Practices for Constructor Injection
To make the most out of constructor injection in Spring, consider the following best practices:
-
Use Constructor Injection for Mandatory Dependencies: Reserve constructor injection for mandatory dependencies that are essential for the functioning of the class. Optional or configurable dependencies can be injected via setter methods.
-
Limit the Number of Dependencies: Aim to keep the number of constructor parameters to a manageable level, as an excessive number of dependencies can indicate design issues such as low cohesion and high coupling.
-
Prefer Immutable Objects: Utilize constructor injection to create immutable objects with injected dependencies, promoting better encapsulation and immutability.
-
Leverage IoC Container Features: Take advantage of Spring's IoC container to manage complex dependencies and their configuration, allowing for flexible and modular application design.
My Closing Thoughts on the Matter
Constructor injection in Spring brings numerous advantages to the table, from promoting immutability and clarity to facilitating easier testing and reliable dependency management. By harnessing the power of constructor injection, you can build robust and maintainable Java applications with Spring's dependency injection capabilities.
In this blog post, we've covered the fundamentals of constructor injection in Spring, its benefits, and best practices to follow when implementing it in your projects. Whether you opt for XML-based configuration or embrace the simplicity of annotation-based configuration, constructor injection remains a valuable tool in the arsenal of Spring developers. Embrace constructor injection, tame your dependencies, and unlock the full potential of your Spring-powered applications.
For further exploration of dependency injection and Spring framework, be sure to check out the official Spring documentation for comprehensive insights into best practices and advanced features. Happy coding!