Unlocking Speed: Mastering PostgreSQL Data Types
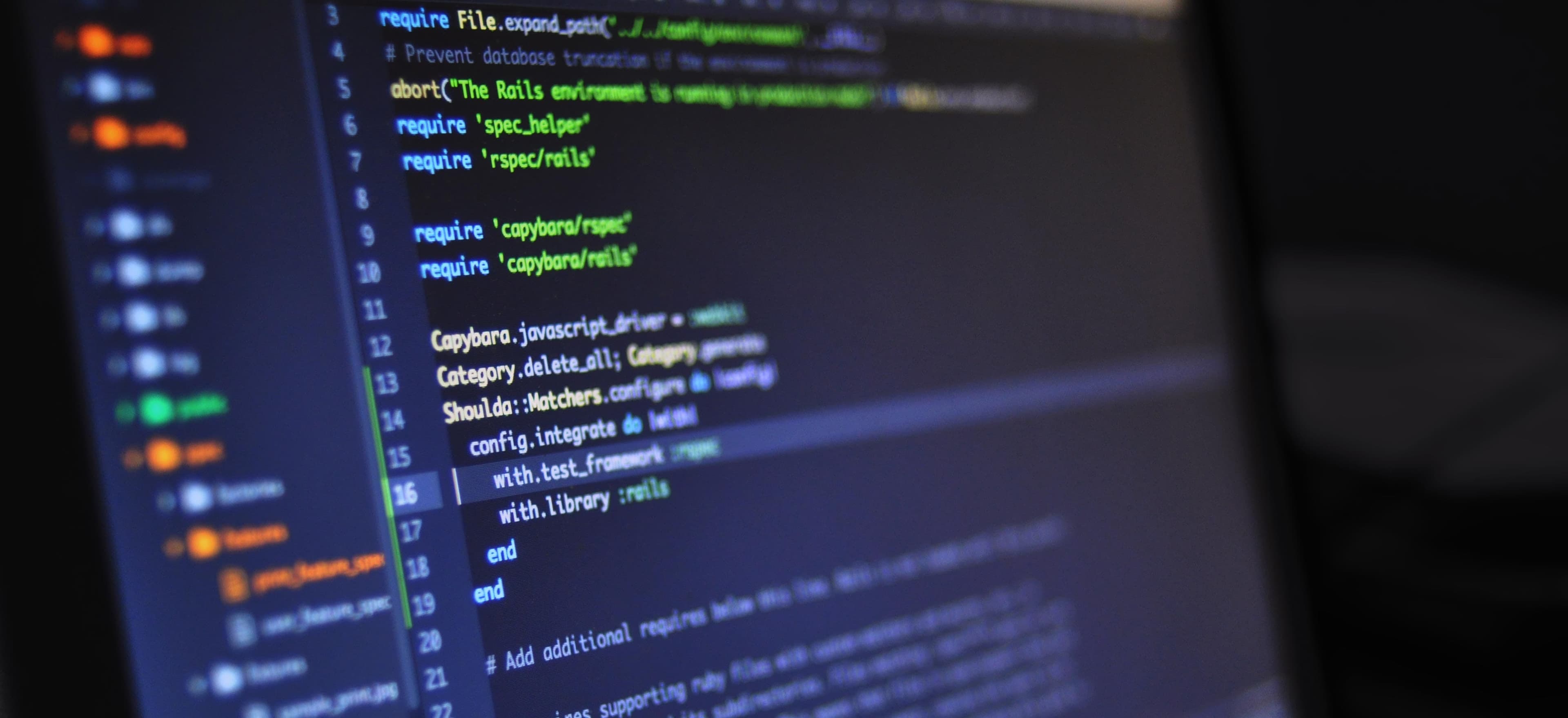
- Published on
Unlocking Speed: Mastering PostgreSQL Data Types
In the world of database management systems, speed is often of the essence. For Java developers, PostgreSQL is a popular choice due to its performance, robustness, and extensibility. However, even with a powerful database management system like PostgreSQL, the choice of data types can significantly impact the speed and efficiency of your application. In this blog post, we will explore how the selection of appropriate data types in PostgreSQL can unlock speed for your Java applications.
Why Data Types Matter
Data types play a crucial role in database design and performance optimization. Choosing the right data type can lead to efficient storage, faster retrieval, and better query performance. In PostgreSQL, a wide range of data types is available, each designed for specific use cases. By understanding and utilizing the appropriate data types, you can optimize the speed and efficiency of your Java applications.
Integer Data Types
When dealing with numerical data, choosing the right integer data type is essential for performance. PostgreSQL offers several integer data types, including smallint
, integer
, and bigint
. The choice of integer data type should be based on the range of values the column is expected to hold. For example, if a column will only store small integers, using smallint
instead of integer
or bigint
can save storage space and improve query performance.
// Using smallint data type in PostgreSQL
CREATE TABLE sample_table (
id smallint,
name varchar(50)
);
By choosing the appropriate integer data type, you not only optimize storage but also enhance the overall performance of your Java application when interacting with the database.
Text Data Types
In Java applications, textual data is pervasive, and choosing the right data type for text fields in PostgreSQL can impact performance. PostgreSQL offers several text data types, including char
, varchar
, and text
. The choice between these data types depends on the length and variability of the textual data. Using the most suitable text data type can lead to efficient storage and faster query processing.
// Using varchar data type for variable-length textual data
CREATE TABLE text_table (
id serial,
content varchar(255)
);
By selecting the appropriate text data type, you can ensure that your Java application interacts with text fields in the database with optimal speed and efficiency.
Indexing Considerations
In PostgreSQL, indexing plays a crucial role in query performance. When choosing data types, it's important to consider the impact on indexing. Data types such as varchar
and text
can be indexed, but indexing long text fields may lead to performance overhead. Understanding the trade-offs between different data types and their indexability is essential for optimizing query speed in your Java applications.
Using Composite Data Types
PostgreSQL allows the creation of composite data types, which can be beneficial for organizing and querying structured data efficiently. Java developers can leverage composite data types to model complex objects in the database, leading to improved query performance and streamlined data retrieval.
// Creating a composite type in PostgreSQL
CREATE TYPE address AS (
street_address text,
city varchar(50),
postal_code varchar(10)
);
CREATE TABLE employee (
id serial,
emp_name varchar(100),
emp_address address
);
By utilizing composite data types, you can enhance the speed and agility of your Java applications when dealing with complex, structured data in PostgreSQL.
Understanding Array Data Types
Array data types in PostgreSQL provide a powerful way to store and query arrays of values. Java developers can benefit from array data types when dealing with collections of data in their applications. By utilizing array data types efficiently, you can improve the performance of operations involving multiple related values.
// Using array data type in PostgreSQL
CREATE TABLE student (
id serial,
subjects varchar(50)[]
);
By incorporating array data types where appropriate, you can streamline data storage and retrieval, ultimately enhancing the speed of your Java applications when working with collections of values.
The Last Word
In conclusion, mastering PostgreSQL data types is essential for unlocking speed in Java applications. By choosing the right data types, understanding their impact on storage and indexing, and leveraging advanced features such as composite and array data types, you can optimize the performance of your Java applications when interacting with PostgreSQL. Remember, thoughtful consideration of data types can lead to significant speed improvements, making your applications more efficient and responsive.
Unlock the full potential of PostgreSQL data types and supercharge the speed of your Java applications today!
For further reading on PostgreSQL data types, check out the official PostgreSQL documentation and the PostgreSQL Data Types Tutorial.
Happy coding!