Boost Heap Size? Avoid the Cobra Effect Trap!
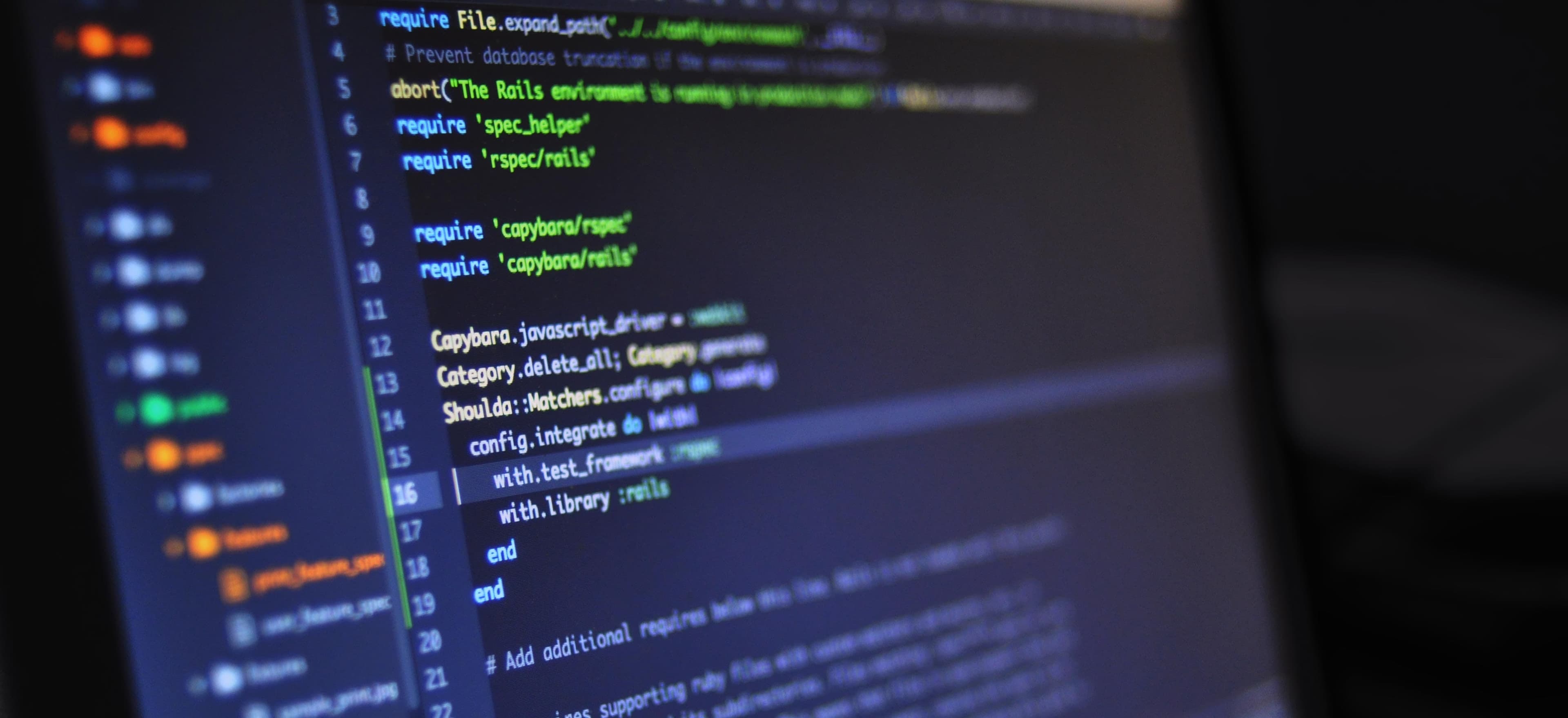
- Published on
Boosting Java Heap Size: Avoiding the Cobra Effect
In the world of Java development, one of the most common performance-related issues that developers face is memory management. When dealing with large and complex applications, adjusting the Java Heap Size becomes crucial for maintaining optimal performance. However, increasing the heap size without proper understanding can lead to what's known as the "Cobra Effect" - a solution that creates more problems than it solves. This blog post aims to decipher the intricacies of heap size management and provide guidance on avoiding potential pitfalls.
Understanding Java Heap Size
In Java, the heap is the runtime data area where objects are stored. When the application runs out of heap space, it throws java.lang.OutOfMemoryError
. Understanding and managing the heap size is essential for preventing this error and optimizing the application's performance.
The Cobra Effect
The "Cobra Effect" refers to the phenomenon where an attempted solution to a problem actually exacerbates the problem. In the context of Java heap management, this can occur when developers increase the heap size indiscriminately as a knee-jerk reaction to OutOfMemoryError
without fully understanding the root cause. This can lead to inefficient memory usage, longer garbage collection pauses, and ultimately, degraded performance.
Factors to Consider Before Increasing Heap Size
Before rushing to boost the Java heap size, it's imperative to consider a few factors:
-
Memory Leak Analysis: Conduct a thorough analysis to identify any potential memory leaks within the application. A memory leak is a common culprit behind
OutOfMemoryError
and simply increasing the heap size would only mask the underlying issue. -
GC Analysis: Understand the garbage collection behavior and patterns within the application. Increasing heap size without understanding GC behavior can lead to longer pause times and increased resource consumption.
-
Application Profiling: Utilize profiling tools to gain insights into the memory usage patterns and identify areas that may benefit from optimization before resorting to increasing the heap size.
How to Properly Adjust the Java Heap Size
When increasing the Java heap size becomes necessary, it's crucial to approach it methodically to avoid the Cobra Effect. Here are the steps to follow:
Step 1: Understanding the Current Heap Size
Start by determining the current heap size configuration. This can be done by executing the following command:
java -XX:+PrintFlagsFinal -version | grep MaxHeapSize
This command will print the current maximum heap size. Understanding the existing configuration serves as a baseline for making informed adjustments.
Step 2: Analyzing Application's Memory Requirements
Conduct an in-depth analysis of the application's memory requirements under varying workloads. This involves using tools like JConsole, Java Mission Control, or VisualVM to monitor memory usage patterns and identify the peak memory consumption.
Step 3: Choosing the Right Heap Size
Based on the memory analysis, determine the appropriate heap size required to accommodate the application's memory demands. It's crucial to strike a balance between providing enough memory to the application and avoiding excessive allocation that could lead to longer garbage collection pauses.
Step 4: Setting the New Heap Size
Once the appropriate heap size is determined, it can be adjusted using the -Xmx
flag during application startup, specifying the maximum heap size. For example:
java -Xmx4g -jar yourApplication.jar
In this example, the maximum heap size is set to 4 gigabytes.
Step 5: Monitoring and Fine-tuning
After adjusting the heap size, monitor the application's performance, memory usage, and garbage collection behavior. Fine-tune the heap size as necessary based on the observed metrics.
Best Practices for Effective Heap Management
In addition to adjusting the heap size, adhering to best practices for effective heap management is essential for maintaining optimal performance:
-
Memory Optimization: Implement efficient data structures and algorithms to minimize memory consumption.
-
Object Lifecycle Management: Ensure proper management of object lifecycle, avoiding unnecessary object retention and promoting timely garbage collection.
-
Tune Garbage Collection: Configure garbage collection settings based on the application's memory characteristics to minimize pause times and resource overhead.
-
Memory Profiling: Regularly perform memory profiling to identify and address memory inefficiencies and potential leaks.
By incorporating these best practices, developers can proactively manage memory utilization and minimize the reliance on simply increasing the heap size as a solution.
The Bottom Line
In the realm of Java development, as with any other technology, understanding the core principles behind performance optimization is crucial for avoiding the Cobra Effect. While adjusting the Java heap size can provide a short-term remedy for memory-related issues, it should be approached with careful consideration and supplemented with proactive memory management practices. By understanding the application's memory requirements, optimizing memory usage, and continuously monitoring performance, developers can steer clear of falling into the trap of the Cobra Effect when it comes to boosting Java heap size.
Remember, increasing the heap size should never be the default solution - it should be a well-informed decision based on thorough analysis and best practices.
For further understanding of the Java heap and memory management, refer to the Java documentation on Garbage Collection Basics.
Now, armed with the knowledge of effective heap management, let's optimize those Java applications while avoiding the Cobra Effect trap!
Checkout our other articles