Boost Your API: Secrets to Enhance RESTful Service Discoverability
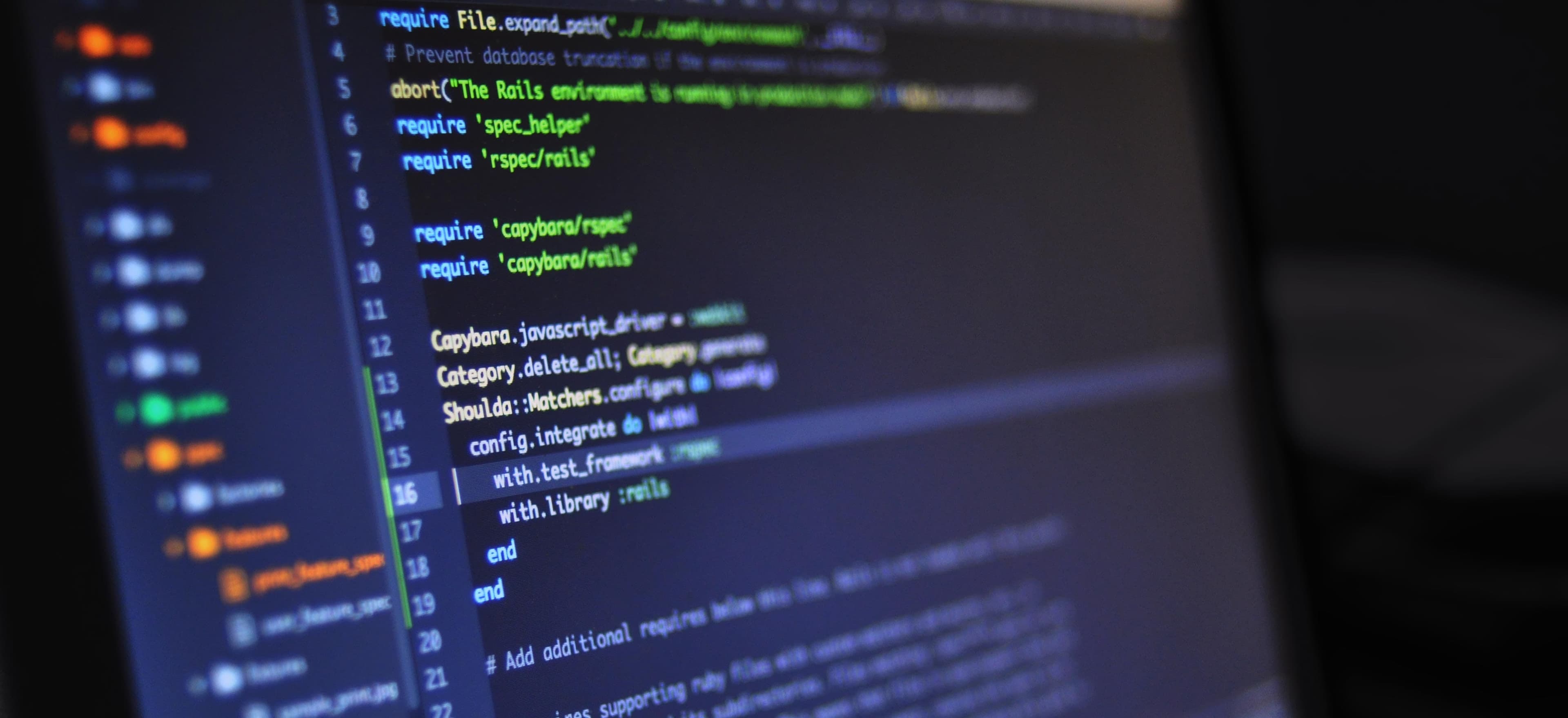
- Published on
Boost Your API: Secrets to Enhance RESTful Service Discoverability
In the world of web development, creating a robust and discoverable API is a crucial aspect of building a successful application. RESTful services, when designed effectively, can significantly enhance the discoverability of your API and streamline the process for developers to integrate with your application. In this article, we will delve into the secrets of enhancing the discoverability of your RESTful services using Java, and explore the best practices to make your API stand out.
What is API Discoverability?
API discoverability refers to the ease with which developers can find, explore, and understand the capabilities of an API. A discoverable API communicates its features, resources, and endpoints effectively, making it intuitive for developers to integrate and build upon. In the realm of RESTful services, discoverability plays a pivotal role in attracting developers to engage with your API.
Utilizing Java for Building a Discoverable API
Java, with its robust and scalable ecosystem, provides an excellent foundation for building high-performance RESTful services. Leveraging Java for API development empowers developers to craft discoverable services that adhere to industry standards and best practices. The following sections will explore various techniques and best practices in Java to enhance the discoverability of your RESTful API.
RESTful Service Documentation with Swagger
Documentation serves as the primary gateway for developers to understand and integrate with an API. Swagger, now known as OpenAPI, simplifies the process of designing, documenting, and visualizing RESTful services. By integrating Swagger with your Java-based RESTful service, you can automatically generate interactive documentation that showcases the available endpoints, request parameters, response schemas, and example requests. This intuitive documentation significantly enhances the discoverability of your API by providing a clear and structured overview of its functionalities.
Integrating Swagger into Your Java Service
import io.swagger.annotations.Api;
import io.swagger.annotations.ApiOperation;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@Api(value = "Example API")
@RestController
@RequestMapping("/example")
public class ExampleController {
@ApiOperation(value = "Get all examples")
@GetMapping("/")
public List<Example> getAllExamples() {
// Implementation to retrieve all examples
}
}
In the above example, annotations provided by the Swagger library enable developers to document the API endpoints, operations, and response models directly within the Java code. This approach ensures that the API documentation stays synchronized with the actual implementation, fostering discoverability and reducing the maintenance overhead of keeping documentation up-to-date.
By incorporating Swagger into your Java-based RESTful service, you equip developers with a comprehensive and interactive resource for exploring and understanding the capabilities of your API.
HATEOAS for Navigable APIs
Hypermedia as the Engine of Application State (HATEOAS) is a fundamental principle of RESTful architecture that enables the construction of navigable APIs. By imbuing your API with HATEOAS, you provide developers with links to related resources within the API responses, allowing for dynamic exploration and traversal of the API structure. This approach enhances the discoverability by enabling developers to seamlessly navigate between different resources, thus gaining a deeper understanding of the API's capabilities.
Implementing HATEOAS in Java
import org.springframework.hateoas.Resource;
import org.springframework.hateoas.mvc.ControllerLinkBuilder;
Resource<Example> exampleResource = new Resource<>(example);
exampleResource.add(ControllerLinkBuilder.linkTo(ControllerLinkBuilder.methodOn(ExampleController.class).getAllExamples()).withRel("examples"));
In the above snippet, the use of Spring HATEOAS allows for the creation of resource representations with embedded links to related endpoints. When a developer interacts with your API, these embedded links serve as guideposts, facilitating seamless traversal through the API resources. By incorporating HATEOAS in your Java-based RESTful service, you empower developers with the ability to explore and comprehend the API's structure dynamically.
Versioning and API Stability
Maintaining backward compatibility and ensuring the stability of your API are paramount for fostering trust and reliability among developers. Versioning your API provides a clear delineation of changes and allows for the coexistence of multiple API versions, preventing disruptions to existing integrations. In Java, employing robust versioning strategies, such as URI-based versioning or custom request headers, ensures that developers can seamlessly adapt to updates while preserving the discoverability of different API versions.
Final Considerations
In the realm of API development, enhancing the discoverability of your RESTful services is pivotal for attracting and empowering developers to integrate with your application. Leveraging Java and adhering to industry best practices enable you to craft discoverable APIs that resonate with developers and streamline the integration process. By incorporating techniques such as interactive documentation with Swagger, HATEOAS for navigable APIs, and strategic versioning, you can elevate the discoverability of your RESTful services, ultimately fostering a thriving developer ecosystem around your API.
Incorporate these best practices into your Java-based RESTful services and witness the profound impact on the discoverability and integration experience of your API.
Remember, the key to a successful API lies in its discoverability!
So, what are your thoughts on enhancing RESTful service discoverability in Java? Share your experiences and insights!
Checkout our other articles