JAX-RS vs. Spring MVC: Mastering XML List Responses
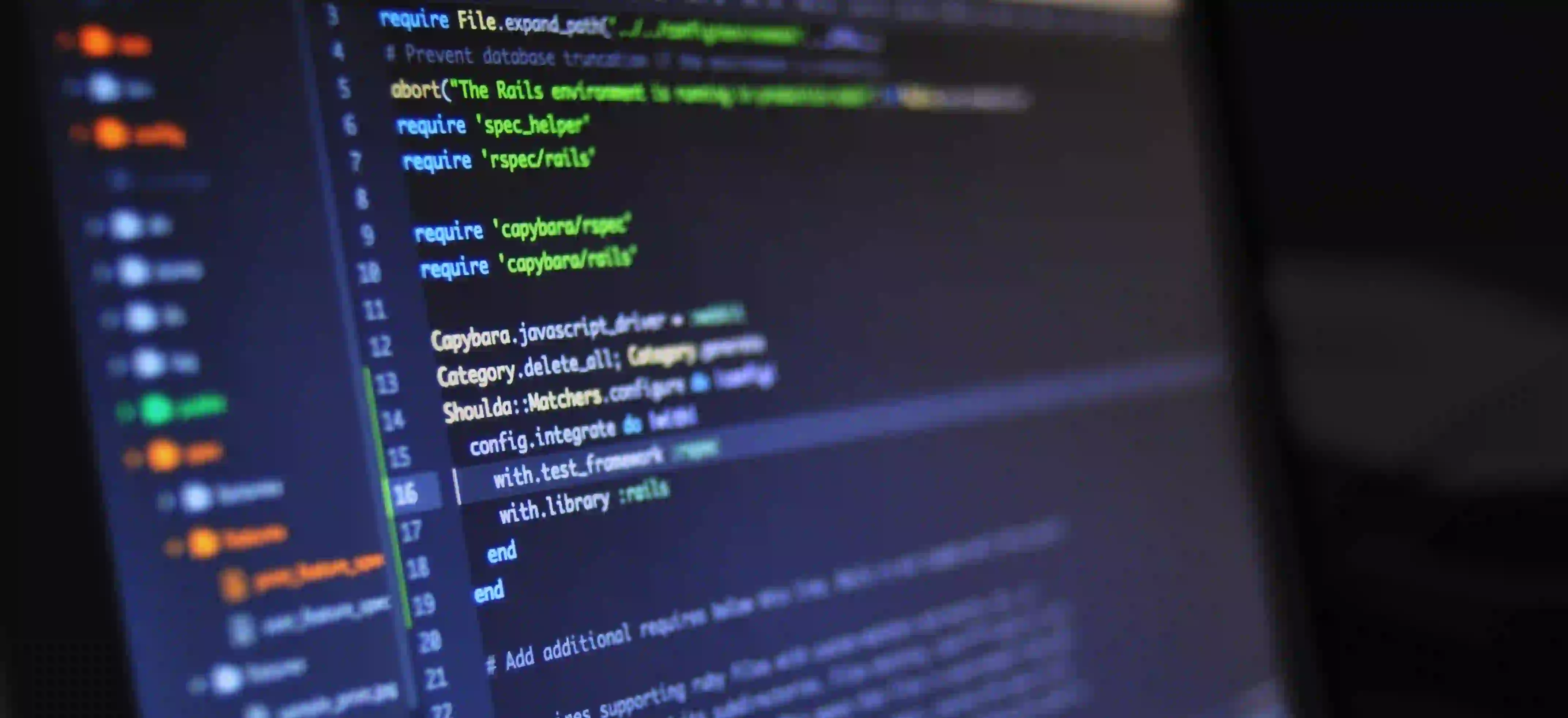
JAX-RS vs. Spring MVC: Mastering XML List Responses
When it comes to building RESTful web services in Java, JAX-RS and Spring MVC are two popular choices. Both frameworks provide powerful tools for creating APIs that deliver XML responses. In this post, we'll compare how JAX-RS and Spring MVC handle the task of producing XML lists and explore best practices for mastering XML list responses in each framework.
Understanding XML List Responses
Before we dive into the details of working with XML list responses in JAX-RS and Spring MVC, let's clarify what we mean by an XML list response. In the context of a RESTful API, an XML list response refers to a collection of data serialized in XML format. This collection could be an array of objects, a list of key-value pairs, or any other structured data set.
XML is a widely used data format for APIs due to its readability, extensibility, and compatibility with a wide range of systems. When designing a RESTful API that returns a list of resources, it's crucial to ensure that the XML representation is well-formed and follows best practices for readability and consistency.
Producing XML List Responses in JAX-RS
JAX-RS, which is part of the Java EE platform, provides a powerful and flexible way to build RESTful web services. When it comes to producing XML list responses, JAX-RS offers several approaches. One common way is to use JAXB (Java Architecture for XML Binding) to marshal Java objects into XML.
Here's an example of a JAX-RS resource method that returns a list of objects as an XML response:
@GET
@Produces(MediaType.APPLICATION_XML)
public List<SomeObject> getXmlList() {
List<SomeObject> objectList = // retrieve objects from data source
return objectList;
}
In this example, the @Produces(MediaType.APPLICATION_XML)
annotation indicates that the method produces XML responses. When the getXmlList
method is invoked, JAX-RS will automatically marshal the List<SomeObject>
into XML using JAXB.
One of the key advantages of using JAX-RS for producing XML list responses is its seamless integration with JAXB. By leveraging JAXB annotations on the Java object model, developers can fine-tune how the XML representation is generated, including customizing element names, handling relationships between objects, and controlling the overall XML structure.
Mastering XML List Responses in Spring MVC
Spring MVC, a part of the Spring Framework, is another popular choice for building RESTful web services in Java. Like JAX-RS, Spring MVC provides features for producing XML responses, including support for generating XML list responses.
In Spring MVC, you can use the @ResponseBody
annotation to indicate that a method's return value should be serialized directly to the HTTP response body. When returning a list of objects as an XML response, you can leverage Spring's built-in support for XML marshalling.
Here's an example of a Spring MVC controller method that returns a list of objects as an XML response:
@GetMapping(value = "/xmlList", produces = MediaType.APPLICATION_XML_VALUE)
@ResponseBody
public List<SomeObject> getXmlList() {
List<SomeObject> objectList = // retrieve objects from data source
return objectList;
}
In this example, the @ResponseBody
annotation instructs Spring MVC to serialize the List<SomeObject>
directly to the HTTP response body as XML. The produces
attribute of the @GetMapping
annotation specifies that the method produces XML responses.
One of the strengths of Spring MVC for handling XML list responses is its integration with JAXB or other XML marshalling libraries. Spring's flexibility allows developers to choose the XML binding approach that best fits their requirements, whether it's JAXB, Jackson, or another library.
Best Practices for Mastering XML List Responses
Regardless of whether you're using JAX-RS or Spring MVC, there are several best practices to keep in mind when working with XML list responses in your RESTful APIs:
1. Use Proper Object Modeling
Ensure that the Java object model you're serializing to XML is well-designed and follows best practices for representing the underlying data structure. This includes using appropriate data types, defining relationships between objects, and considering the XML representation when designing the object model.
2. Leverage JAXB Annotations
If you're using JAX-RS, take advantage of JAXB annotations to customize the XML representation of your Java objects. This allows you to exert fine-grained control over how the XML is generated and ensure that it aligns with your API's requirements.
3. Choose the Right XML Binding Approach
In Spring MVC, consider the XML binding approach that best suits your project, whether it's JAXB, Jackson, or another library. Evaluate the features and capabilities of each library to determine which one aligns with your XML serialization needs.
4. Ensure Consistency and Readability
Regardless of the framework you're using, strive for consistency and readability in your XML list responses. Follow XML best practices, such as consistent indentation, clear element naming, and proper handling of nested structures, to make the XML responses more understandable for clients.
Final Considerations
In the realm of RESTful web services in Java, both JAX-RS and Spring MVC offer robust capabilities for producing XML list responses. Whether you prefer the seamless JAXB integration of JAX-RS or the flexibility of XML marshalling in Spring MVC, mastering XML list responses is essential for delivering well-designed and maintainable APIs.
By understanding the nuances of working with XML list responses in each framework and following best practices for object modeling and XML binding, you'll be well-equipped to create APIs that excel in delivering structured XML data to clients.
In this post, we've only scratched the surface of XML list responses in JAX-RS and Spring MVC. For further exploration, consider looking into advanced topics such as handling complex XML structures, optimizing XML performance, and integrating XML schema validation into your RESTful APIs.
For a deep dive into XML binding with JAX-RS and Spring MVC, check out the following resources:
Mastering XML list responses is a valuable skill for any Java developer building RESTful APIs, and with the right knowledge and tools at your disposal, you can elevate the quality and usability of your XML-based web services.