Securing Your Code: Stop Log Message Exploits!
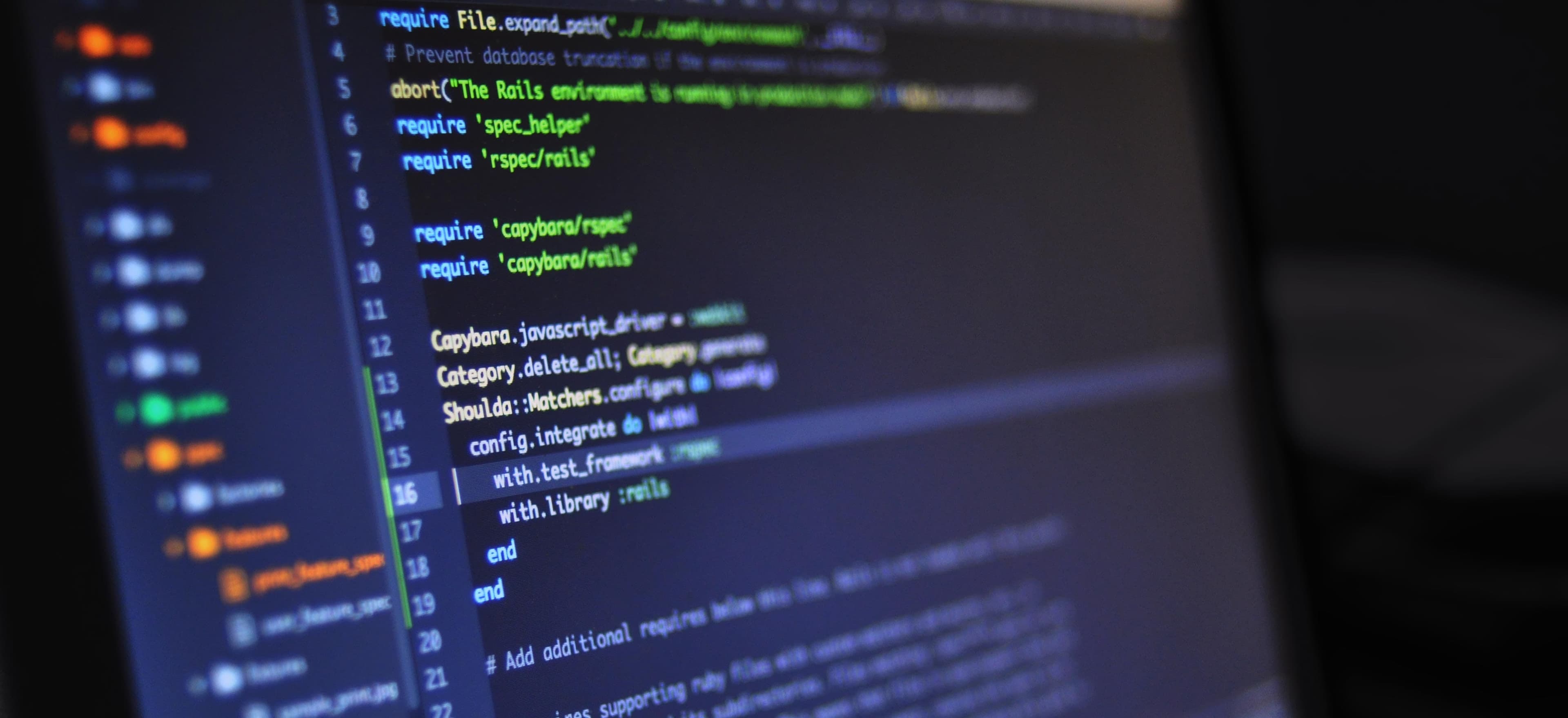
- Published on
Securing Your Code: Stop Log Message Exploits!
When it comes to ensuring the security of your Java applications, preventing log message exploits is a crucial aspect that should not be underestimated. Many developers focus on securing the core functionality of their applications, but they often overlook the potential vulnerabilities within their logging mechanisms. In this article, we will dive into the concept of log message exploits, explore the potential risks they pose, and discuss best practices for preventing them in your Java applications.
Understanding Log Message Exploits
Log message exploits occur when sensitive or confidential information is inadvertently logged in an application's log files. This can include a wide range of data, such as user credentials, personally identifiable information (PII), API keys, or other sensitive configuration details. If these log files are not adequately secured, an attacker who gains access to them could potentially exploit the exposed information to compromise the application's security.
Additionally, excessive or verbose logging can inadvertently disclose implementation details or internal workings of the application, which could aid attackers in identifying potential vulnerabilities or weaknesses to exploit.
Potential Risks of Log Message Exploits
The risks associated with log message exploits are significant and can have far-reaching consequences for both the application and its users. Some of the potential risks include:
- Data Breaches: Exposed sensitive information in log files can lead to data breaches, resulting in compromised user accounts, legal ramifications, and damage to the organization's reputation.
- Regulatory Compliance Violations: Violating regulations such as the General Data Protection Regulation (GDPR) or the Health Insurance Portability and Accountability Act (HIPAA) due to mishandling of sensitive data.
- Security Threats: Attackers leveraging exposed information to launch targeted attacks, gain unauthorized access to systems, or escalate their privileges within the application.
Given the severity of these risks, it is imperative to implement robust measures to prevent log message exploits within your Java applications.
Best Practices for Preventing Log Message Exploits
1. Avoid Logging Sensitive Information
When writing log messages, exercise caution to avoid including sensitive information such as passwords, API keys, or personally identifiable information. Instead, consider logging only non-sensitive metadata or using placeholders in place of actual sensitive data.
// Avoid logging sensitive information directly
logger.info("User login attempt with username: " + username);
// Use placeholders for sensitive information
logger.info("User login attempt with username: {}", username);
Using placeholders not only mitigates the risk of exposing sensitive data but also improves the readability of log messages without compromising security.
2. Implement Log Level Control
Carefully control the log levels for different types of messages. Avoid logging sensitive information at levels that are excessively verbose, such as DEBUG or TRACE. Reserve these levels for debugging purposes only and ensure that sensitive details are only logged at appropriate levels, such as INFO or higher.
// Log sensitive information at the INFO level
if (loginSuccessful) {
logger.info("User logged in successfully: {}", username);
} else {
logger.warn("Login failed for user: {}", username);
}
By implementing strict control over log levels, you can minimize the exposure of critical information in log files.
3. Sanitize Log Entries
Before logging any data, especially data from external sources, ensure that it is properly sanitized to remove any potential sensitive information. For example, when logging HTTP request details, sanitize the request parameters to exclude any sensitive data before logging the request details.
// Sanitize HTTP request parameters before logging
String sanitizedParameters = sanitize(parameters);
logger.info("Received HTTP request - Path: {}, Parameters: {}", path, sanitizedParameters);
Sanitizing log entries adds an extra layer of protection against inadvertently logging sensitive information.
4. Secure Log File Access
Ensure that access to log files is restricted to authorized personnel and processes. Set appropriate file permissions to prevent unauthorized users from accessing or tampering with the log files. Additionally, consider encrypting sensitive log files to provide an added layer of security.
5. Use Secure Logging Libraries
Utilize secure and reputable logging frameworks such as Log4j 2, Logback, or JUL (Java Util Logging) with a focus on their security features and best practices. Stay updated with the latest releases and security patches for the chosen logging library to address any potential vulnerabilities.
By adhering to these best practices, you can significantly reduce the risk of log message exploits in your Java applications, enhancing their overall security posture.
A Final Look
Securing your Java applications goes beyond addressing the primary functionalities and also involves safeguarding against potential vulnerabilities within the logging mechanisms. Log message exploits pose a significant threat to the security and integrity of your applications, making it essential to adopt proactive measures to prevent them. By adhering to best practices such as avoiding logging sensitive information, implementing log level control, sanitizing log entries, securing log file access, and using secure logging libraries, you can effectively mitigate the risks associated with log message exploits and ensure the confidentiality and integrity of your application's log data. Incorporating these security measures will not only protect your application and its users but also contribute to maintaining regulatory compliance and safeguarding your organization's reputation.
Incorporate these best practices into your Java projects to ensure that your log files remain a valuable tool for debugging and auditing, without inadvertently becoming a liability due to log message exploits. Safeguard your application's logs, and rest assured that your sensitive information remains secure.
Remember, proactive security measures today prevent potential breaches and headaches tomorrow!
Checkout our other articles