Poka-Yoke in Dev: Avoiding Costly Coding Errors
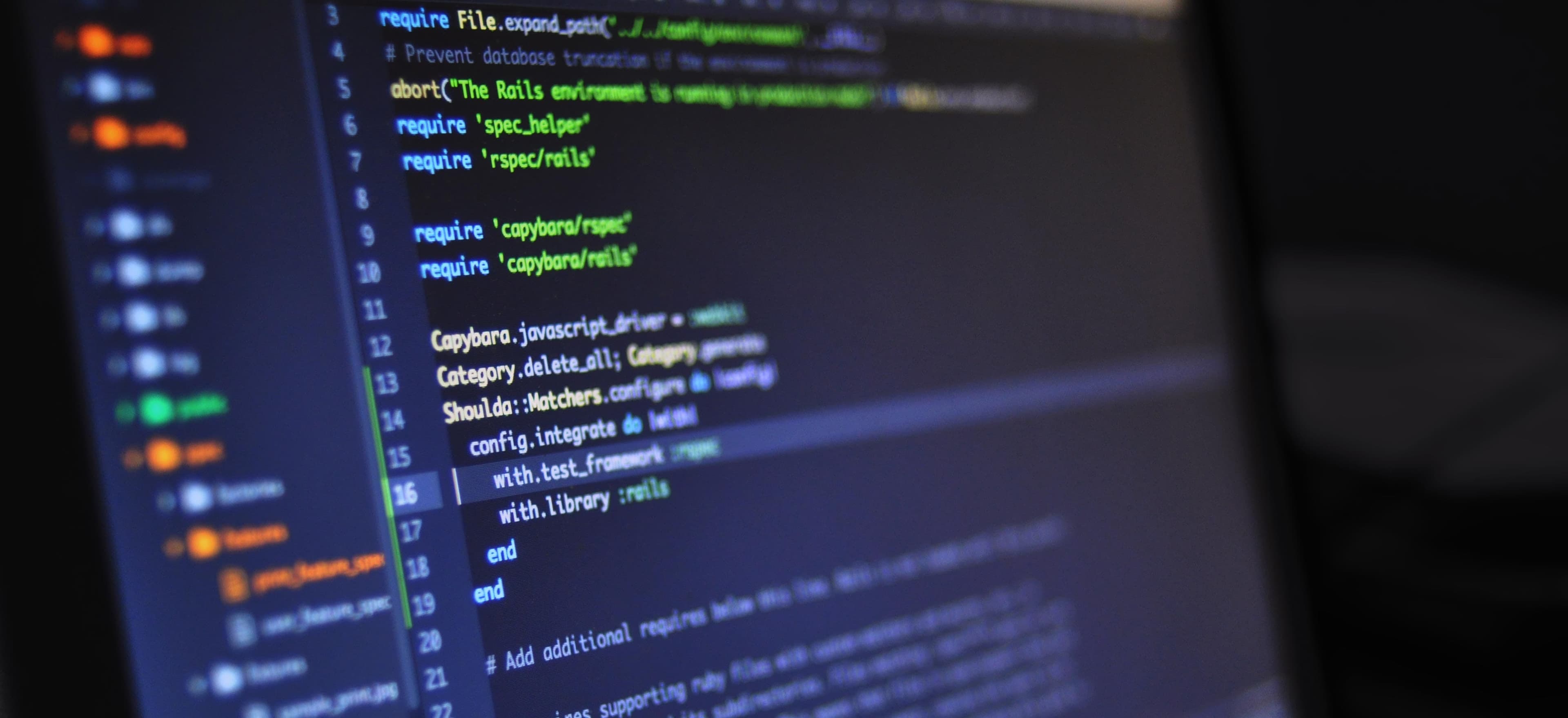
- Published on
Poka-Yoke in Dev: Avoiding Costly Coding Errors
In the world of software development, errors and bugs are inevitable. However, their impact can be mitigated through a concept that originates from lean manufacturing - Poka-Yoke, which roughly translates to "mistake-proofing" in Japanese. In the context of software development, Poka-Yoke refers to the practice of incorporating mechanisms that prevent mistakes from occurring in the first place. This can save valuable time and resources that would otherwise be spent on identifying and rectifying errors. In this blog post, we'll explore how Poka-Yoke principles can be applied to Java development to avoid costly coding errors.
Understanding Poka-Yoke in Software Development
Poka-Yoke in software development involves implementing safeguards to minimize the risk of errors during coding, testing, and execution. By anticipating potential mistakes and designing the system to prevent them, developers can significantly reduce the likelihood of bugs slipping into the codebase.
Applying Poka-Yoke in Java Development
1. Input Validation
One of the most common sources of errors in software applications is invalid user input. In Java, input validation can be enforced using classes from the java.util.regex
package or by leveraging frameworks like Hibernate Validator. By validating user input at the entry points of the system, developers can prevent invalid data from propagating through the application and causing errors downstream.
import java.util.regex.Pattern;
public class InputValidator {
private static final Pattern EMAIL_PATTERN = Pattern.compile("^[A-Za-z0-9+_.-]+@(.+)$");
public boolean validateEmail(String email) {
return EMAIL_PATTERN.matcher(email).matches();
}
}
In the above example, the InputValidator
class uses a regular expression pattern to validate email addresses. By enforcing this validation at the point of entry, the system ensures that only valid email addresses are processed, reducing the risk of errors related to malformed input.
2. Null Checking
Handling null values improperly can lead to NullPointerExceptions, a common source of runtime errors in Java applications. By adopting a defensive programming approach and validating the presence of expected values, developers can preemptively prevent these errors.
public class DataProcessor {
public void processData(String data) {
if (data != null) {
// Process the data
} else {
// Handle the absence of data
}
}
}
In the DataProcessor
class, the processData
method explicitly checks for null input before proceeding with data processing. This simple validation prevents potential null-related errors, safeguarding the application against unexpected runtime failures.
3. Immutable Objects
Incorporating immutability in Java objects can act as a Poka-Yoke mechanism to prevent unintended state changes. By declaring classes as final
and initializing their fields in the constructor, developers can create objects that are inherently resistant to accidental modifications.
public final class ImmutableObject {
private final String value;
public ImmutableObject(String value) {
this.value = value;
}
public String getValue() {
return value;
}
}
The ImmutableObject
class only allows the value
to be set once during construction, ensuring that its state remains constant throughout its lifecycle. This design choice eliminates the risk of mutable object state leading to unexpected behavior or errors.
4. Enumerations for Type Safety
Using enumerations to represent a fixed set of constants can enhance type safety in Java applications. By defining clear and distinct types for different categories, developers can avoid errors stemming from ambiguous or mismatched values.
public enum DayOfWeek {
MONDAY,
TUESDAY,
WEDNESDAY,
THURSDAY,
FRIDAY,
SATURDAY,
SUNDAY
}
In the DayOfWeek
enumeration, each constant represents a specific day of the week. By utilizing enums for such categorizations, developers can eliminate the risk of using incorrect or undefined values, thereby reducing potential errors related to type mismatches.
Lessons Learned
Applying Poka-Yoke principles in Java development allows developers to proactively address potential sources of errors and enhance the robustness of their code. By incorporating input validation, null checking, immutability, and type safety measures, developers can prevent costly coding errors from manifesting in production systems. Embracing Poka-Yoke not only contributes to the reliability of software applications but also fosters a proactive mindset towards error prevention in the development process.
In a nutshell, Poka-Yoke in Java development boils down to anticipating, preventing, and safeguarding against potential errors, thereby bolstering the resilience of software systems.
By implementing these practices, developers can build a more robust and reliable codebase, minimizing the likelihood of errors slipping through the cracks and surfacing in production environments.
So let's embrace Poka-Yoke principles and strive to make our Java code more resilient and error-resistant!