Navigating Pitfalls: Mastering Microservices Best Practices
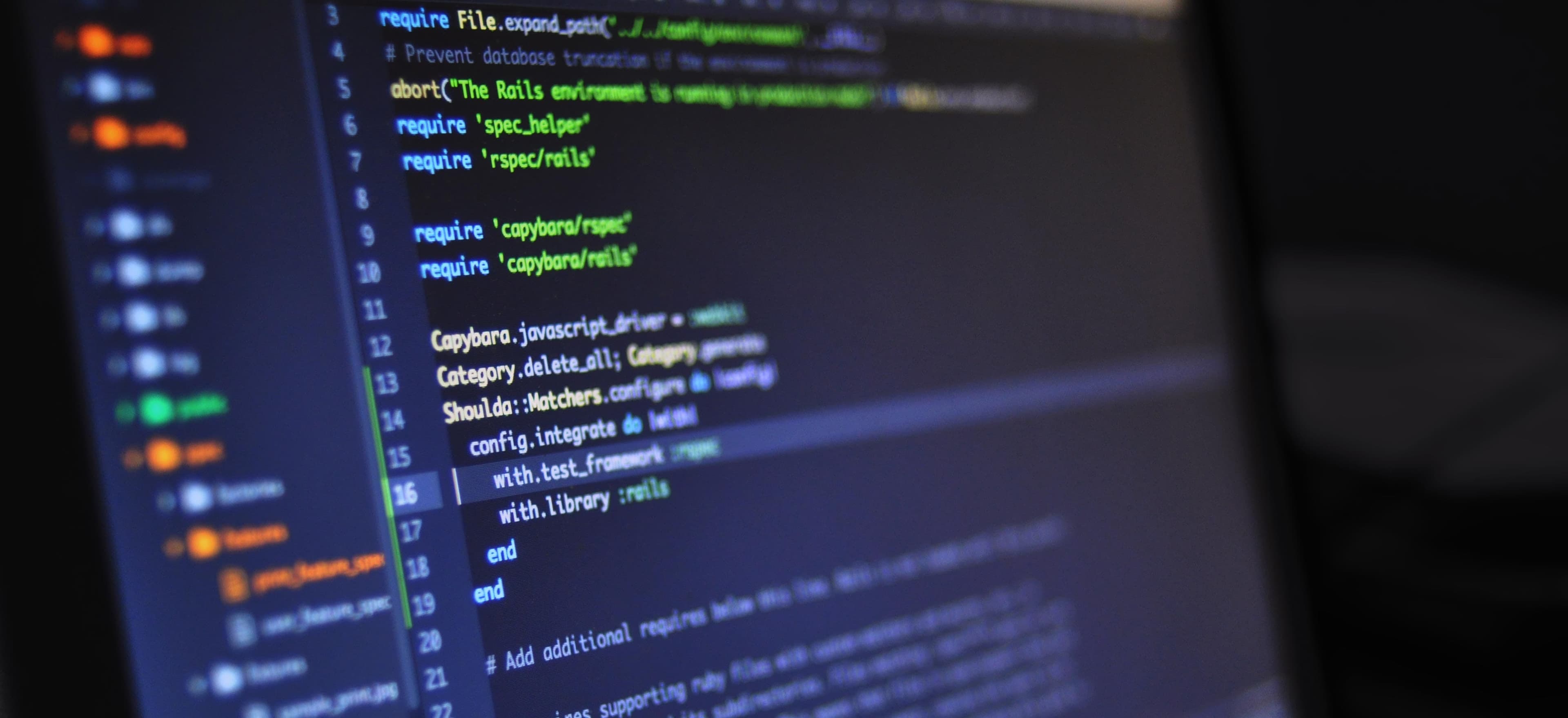
- Published on
Navigating Pitfalls: Mastering Microservices Best Practices
Microservices have become increasingly popular in the world of software development due to their ability to promote scalability, flexibility, and maintainability. However, the implementation of microservices also comes with its own set of challenges and pitfalls. In this article, we will explore some best practices for mastering microservices to navigate these pitfalls effectively.
Understanding Microservices
Before delving into best practices, it's crucial to have a solid understanding of what microservices are. Microservices are a software development technique that structures an application as a collection of loosely coupled services. Each service is highly maintainable and testable, and they operate independently from each other. This architecture enables continuous delivery and deployment of large, complex applications.
Best Practices for Mastering Microservices
1. Domain-Driven Design (DDD)
When embarking on a microservices journey, it is essential to embrace Domain-Driven Design. DDD focuses on modeling based on the business domain, which is crucial for establishing clear boundaries for microservices. By defining the bounded contexts and ubiquitous language, DDD helps to identify the individual microservices and their interactions within the application.
2. Communication Between Microservices
Communication between microservices is a critical aspect of their functionality. Utilizing lightweight protocols like HTTP/REST or messaging queues like RabbitMQ ensures efficient inter-service communication. It's important to carefully consider the communication patterns to avoid tight coupling between services.
3. Containerization with Docker
Docker has become a cornerstone in the world of microservices. It allows services to be isolated and packaged along with their dependencies, providing consistency across different environments. Docker also simplifies deployment and scaling, which are fundamental aspects of microservice architecture.
// Example: Dockerfile for a Java microservice
FROM openjdk:11-jre-slim
COPY ./target/microservice.jar /app/
CMD ["java", "-jar", "/app/microservice.jar"]
In this example, the Dockerfile specifies the deployment steps for a Java microservice, including copying the application JAR and running it with the Java runtime.
4. Decentralized Data Management
Each microservice should have its own database to maintain independence. While this introduces challenges such as data consistency and transactions, it also provides the flexibility to choose the most suitable database for each service's requirements. Implementing database per service pattern helps in minimizing the impact of changes and optimizing performance.
5. Monitoring and Observability
Implementing robust monitoring and observability practices is vital in a microservices environment. Tools like Prometheus, Grafana, and Jaeger aid in tracking the performance, identifying bottlenecks, and troubleshooting issues across the distributed system. Incorporating distributed tracing allows for better visibility into the flow of requests across microservices.
6. Automated Testing and CI/CD
Continuous Integration and Continuous Deployment (CI/CD) pipelines are essential for microservices to ensure the rapid and reliable delivery of changes. Automated testing, including unit tests, integration tests, and contract tests, holds paramount importance in validating the behavior of individual services and their interactions. This prevents regressions and integration issues when deploying changes.
// Example: JUnit test for a Java microservice
@Test
public void whenValidInput_thenCreateResource() {
// Arrange
...
// Act
...
// Assert
...
}
This JUnit test showcases the typical structure of a unit test for a Java microservice, where the input is provided, the operation is performed, and the outcome is verified.
My Closing Thoughts on the Matter
Mastering microservices involves a blend of architectural principles, design patterns, and operational practices. Embracing domain-driven design, enabling effective communication, leveraging containerization, decentralizing data management, prioritizing monitoring and observability, and implementing automated testing are crucial components of successful microservice implementation.
By adhering to these best practices, developers and organizations can navigate the potential pitfalls of microservices and harness their full potential to build scalable and resilient applications.
Incorporating microservices best practices ensures that the advantages of this architecture outweigh the challenges, paving the way for robust, agile, and maintainable systems. Whether you are venturing into microservices for the first time or seeking to optimize your existing microservice architecture, these best practices serve as a guiding light in your journey towards mastering microservices.