Choosing the Right Garbage Collector: Speed vs. Memory
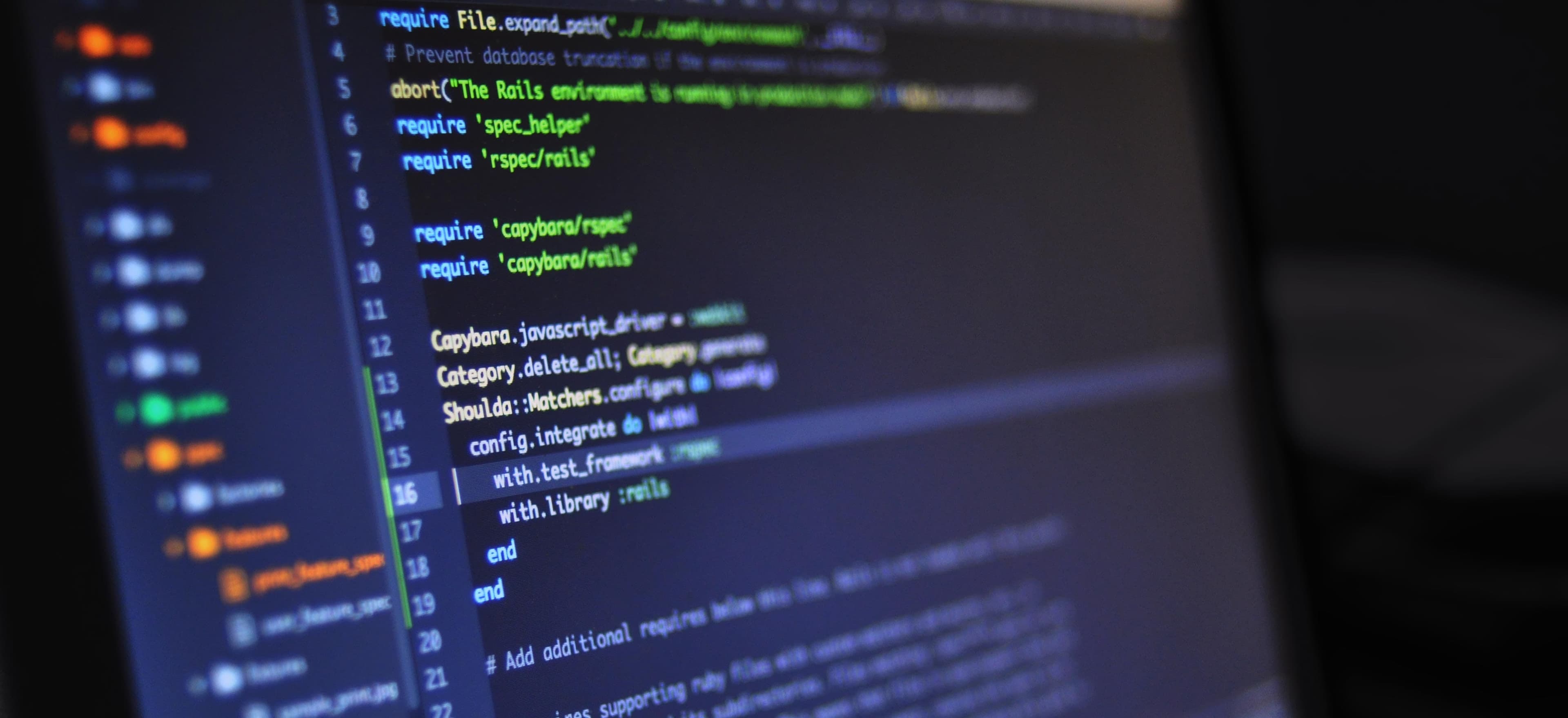
- Published on
Choosing the Right Garbage Collector: Speed vs. Memory
In Java, memory management is primarily handled by the Garbage Collector (GC). The GC is responsible for automatically reclaiming memory that is no longer in use by the program. However, not all garbage collectors are created equal, and choosing the right one can have a significant impact on the performance and memory usage of your Java application.
Understanding Garbage Collection
Before delving into the different types of garbage collectors, let's briefly understand how garbage collection works. In Java, objects that are no longer referenced by the program are considered garbage. The garbage collector's main job is to identify and reclaim this memory so that it can be reused by the application.
When the GC runs, it goes through the heap, identifies unreferenced objects, and reclaims their memory. The efficiency and speed of this process are determined by the garbage collector algorithm being used.
Types of Garbage Collectors
Java offers several garbage collector implementations that are tailored to different application requirements. The most commonly used garbage collectors are:
1. Serial Garbage Collector
The Serial Garbage Collector uses a single thread to perform garbage collection. It is best suited for smaller applications or environments with limited resources. This collector is ideal for single-threaded applications and can be explicitly enabled using the -XX:+UseSerialGC
flag.
Example:
// Enable the Serial Garbage Collector
java -XX:+UseSerialGC MyApp
The Serial Garbage Collector is a good choice when memory footprint is a priority, as it tends to use less memory compared to other collectors. However, it may lead to longer pause times as it halts all application threads during garbage collection.
2. Parallel Garbage Collector
The Parallel Garbage Collector, also known as the throughput collector, is designed for applications with medium to large-sized heaps. It uses multiple threads to perform garbage collection, making it suitable for multi-core systems. This collector can be enabled using the -XX:+UseParallelGC
flag.
Example:
// Enable the Parallel Garbage Collector
java -XX:+UseParallelGC MyApp
The Parallel Garbage Collector aims to maximize throughput by utilizing multiple threads for garbage collection tasks. While it may cause slightly longer pause times compared to the Serial Garbage Collector, it is generally more efficient for larger applications.
3. CMS (Concurrent Mark-Sweep) Garbage Collector
The CMS Garbage Collector is designed to minimize pause times by performing most of the garbage collection work concurrently with the application threads. It is well-suited for large-scale applications where low-latency is critical. To enable the CMS Garbage Collector, you can use the -XX:+UseConcMarkSweepGC
flag.
Example:
// Enable the CMS Garbage Collector
java -XX:+UseConcMarkSweepGC MyApp
The CMS Garbage Collector excels in scenarios where minimizing pause times is essential, making it a preferred choice for applications requiring low-latency and responsiveness.
4. G1 (Garbage-First) Garbage Collector
The G1 Garbage Collector is designed for large heap sizes and aims to provide both high throughput and low-latency garbage collection. It is suitable for applications requiring a balanced performance in terms of both speed and memory management. To enable the G1 Garbage Collector, you can use the -XX:+UseG1GC
flag.
Example:
// Enable the G1 Garbage Collector
java -XX:+UseG1GC MyApp
The G1 Garbage Collector is known for its ability to adapt to different heap sizes and dynamically adjust its garbage collection strategies based on application demands. It is a good choice for applications with large heaps and where predictability of pause times is important.
Choosing the Right Garbage Collector
When it comes to selecting the appropriate garbage collector, there is no one-size-fits-all solution. The choice largely depends on the specific requirements and characteristics of your application.
Factors to Consider
- Throughput vs. Latency: Consider whether your application prioritizes high throughput or minimal pause times.
- Heap Size: Take into account the size of your application's heap, as different collectors are optimized for varying heap sizes.
- Hardware and Environment: Consider the hardware and execution environment where the application will run, such as the number of cores and available memory.
Tuning and Monitoring
It's important to note that garbage collector settings can be tuned based on the specific needs of your application. Monitoring the garbage collection behavior using tools like VisualVM or Java Mission Control can provide valuable insights into the performance of the chosen collector.
Additionally, newer versions of Java often introduce enhancements and optimizations to existing garbage collectors, so staying updated with the latest releases is crucial for leveraging improvements in garbage collection mechanisms.
The Last Word
In conclusion, choosing the right garbage collector for your Java application involves a careful consideration of factors such as throughput, latency, heap size, and the execution environment. By understanding the characteristics of different garbage collectors and their suitability for specific scenarios, you can make an informed decision that optimizes both speed and memory usage in your application.
Remember that the choice of garbage collector is not set in stone and can be revisited as the application's requirements evolve. Continuously monitoring and fine-tuning garbage collection parameters can play a pivotal role in optimizing the performance of your Java application.
In the end, the right garbage collector can make a substantial difference in the overall efficiency and responsiveness of your Java application.
For more in-depth insights into Java garbage collection and memory management, you can refer to the official Java Platform, Standard Edition HotSpot Virtual Machine Garbage Collection Tuning Guide.
Checkout our other articles