Building a Robust Spam Filter with Apache Mahout
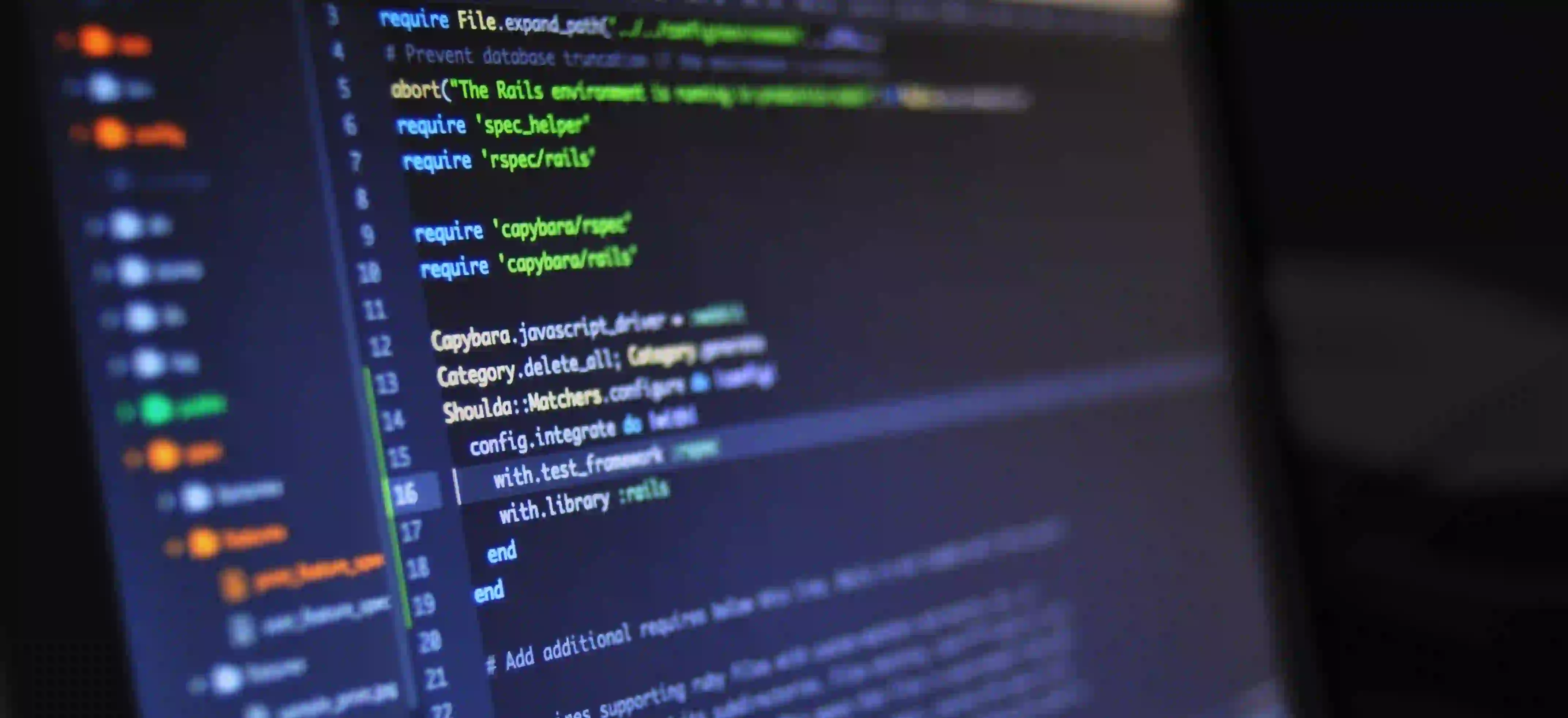
Building a Robust Spam Filter with Apache Mahout
Spam emails - we all receive them, and we all loathe them. But what if there was a way to automatically filter out these pesky messages? Enter Apache Mahout, a powerful machine learning library that can be used to build robust spam filters. In this blog post, we'll dive into the world of Apache Mahout and explore how we can leverage its capabilities to create an effective spam filter.
Understanding Apache Mahout
Apache Mahout is a machine learning library that is designed to work with large-scale, distributed datasets. It provides an extensive set of algorithms for clustering, classification, and recommendation, making it an ideal choice for building spam filters.
One of the key advantages of using Apache Mahout for spam filtering is its ability to handle large volumes of data. With the ever-increasing amount of email traffic, a robust spam filter needs to be able to process and analyze a massive number of messages in real-time. Apache Mahout's scalability and performance make it well-suited for this task.
Preparing the Dataset
Before we can start building our spam filter, we need a dataset of labeled emails. This dataset will consist of two classes: spam and non-spam (referred to as ham). Each email in the dataset will be labeled accordingly.
To illustrate, let's assume we have a dataset consisting of thousands of emails. Each email will have a label indicating whether it is spam or ham. This labeled dataset will serve as the foundation for training our spam filter model.
Training the Spam Filter Model
With our labeled dataset in hand, we can now proceed to train our spam filter model using Apache Mahout. The first step in this process is to preprocess the data. This involves tokenizing the text of each email, removing stop words, and converting the text into a numerical representation that can be used by the machine learning algorithms.
// Preprocess the data
String inputPath = "path_to_input_dataset";
String preprocessedOutputPath = "path_to_preprocessed_data";
EmailToWordCountVectorizer.tokenizeDocs(inputPath, preprocessedOutputPath, new SimpleTokenizer(), 1, 1);
In the code snippet above, we use the EmailToWordCountVectorizer
class from Apache Mahout to tokenize the text of each email and convert it into a word count vector. This vector represents the frequency of each word in the email, which will be the input for our machine learning model.
Next, we can use Apache Mahout's algorithms to train our spam filter model. One popular algorithm for text classification tasks like spam filtering is the Naive Bayes algorithm.
// Train the spam filter model
String modelPath = "path_to_trained_model";
NaiveBayesModel model = NaiveBayes.train(preprocessedOutputPath, 2, 2, 1, false, true, true);
model.serializeTo(modelPath);
In the code above, we use the NaiveBayes algorithm to train our model using the preprocessed dataset. We then serialize the trained model to a file for later use.
Evaluating the Spam Filter Model
Once our spam filter model is trained, we need to evaluate its performance. This involves testing the model on a separate dataset of labeled emails to measure its accuracy in classifying spam and ham.
// Evaluate the spam filter model
String testDatasetPath = "path_to_test_dataset";
LabeledDataset testDataset = DataLoader.loadDataFromText(testDatasetPath);
ConfusionMatrix confusionMatrix = NaiveBayesModelUtils.testSequential(model, testDataset, 2);
double accuracy = confusionMatrix.getAccuracy();
In the code snippet above, we load a separate test dataset and use it to evaluate the performance of our trained spam filter model. We calculate the accuracy of the model using a confusion matrix, which provides insights into its performance in classifying spam and ham emails.
Using the Spam Filter
With a trained and evaluated spam filter model in hand, we can now use it to classify new, unlabeled emails.
// Use the spam filter to classify new emails
String newEmailPath = "path_to_new_email";
String text = getEmailText(newEmailPath);
Map<String, Double> scores = model.classifyFull(text);
double spamProbability = scores.get("spam");
In the code snippet above, we use the trained model to classify a new, unlabeled email. The model assigns a spam probability score to the email, which can be used to determine whether it should be classified as spam or ham.
The Last Word
In this blog post, we've explored how Apache Mahout can be used to build a robust spam filter. We've covered the process of preparing a labeled dataset, training a spam filter model, evaluating its performance, and using it to classify new emails. By leveraging the capabilities of Apache Mahout, we can create an effective spam filter that can help combat the deluge of unwanted emails.
If you're interested in learning more about Apache Mahout and machine learning, be sure to check out the official Apache Mahout website for additional resources and documentation.
Now, armed with the knowledge of Apache Mahout, go forth and conquer the world of spam with your robust spam filter!