Master Local Tests: Run Docker PostgreSQL Like a Pro!
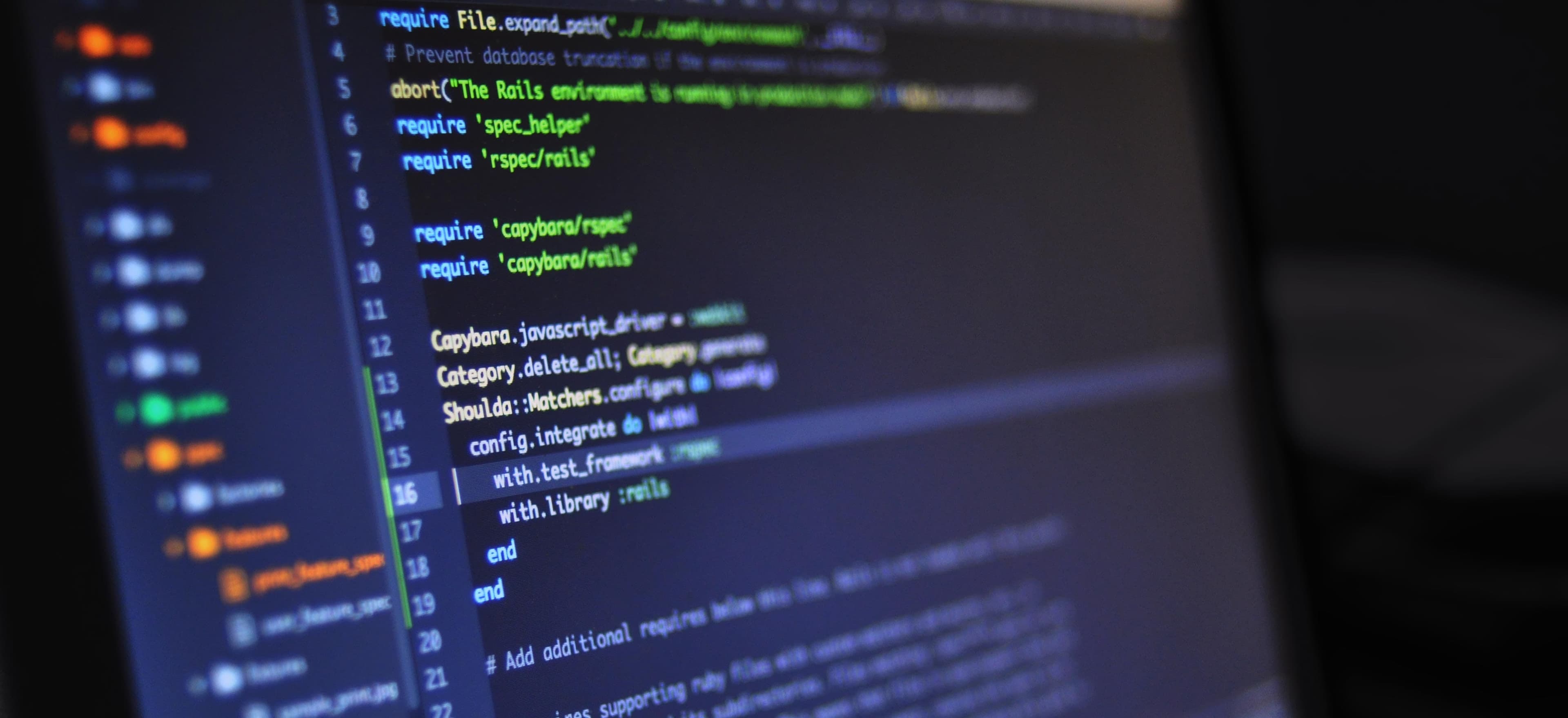
- Published on
Master Local Tests: Running Docker PostgreSQL Like a Pro!
In modern software development, having a robust and reliable testing environment is crucial. When it comes to testing databases, such as PostgreSQL, using Docker can greatly simplify the process and provide a consistent environment across different development machines. In this blog post, we'll explore how to effectively set up and utilize Docker PostgreSQL for local testing of Java applications.
Why Docker PostgreSQL for Local Testing?
Traditional methods of testing against a shared development database or using an in-memory database can lead to inconsistencies and dependencies on external environments. Docker provides a lightweight alternative by allowing us to spin up isolated, reproducible PostgreSQL instances on our local machines. This ensures that our tests run against an environment that closely mirrors production, leading to more reliable test results.
Setting up Docker PostgreSQL for Local Testing
Step 1: Install Docker
Before we can start using Docker PostgreSQL for local testing, we need to have Docker installed on our machine. Docker provides comprehensive installation guides for various operating systems on their official website.
Step 2: Pull the PostgreSQL Docker Image
Docker provides an official PostgreSQL image on Docker Hub, which we can pull using the following command:
docker pull postgres
Step 3: Run a PostgreSQL Container
Once the PostgreSQL image is pulled, we can create and start a new container using the following command:
docker run --name postgresql-container -e POSTGRES_PASSWORD=yourpassword -d -p 5432:5432 postgres
In this command:
--name postgresql-container
assigns a specific name to the container for easy reference.-e POSTGRES_PASSWORD=yourpassword
sets the password for the PostgreSQL user.-d
runs the container in the background.-p 5432:5432
maps the container's PostgreSQL port to the same port on the host machine.
Step 4: Verify the PostgreSQL Container
We can verify that the PostgreSQL container is running by using the command:
docker ps
This should display the running PostgreSQL container along with its details.
Connecting a Java Application to Docker PostgreSQL
Now that we have a Dockerized PostgreSQL instance up and running, let's see how we can connect a Java application to it for local testing.
Using JDBC to Connect
Java Database Connectivity (JDBC) provides a standard API for connecting to databases. We can use a JDBC driver to connect our Java application to the Docker PostgreSQL instance. Below is an example of connecting to the PostgreSQL database using JDBC:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class PostgreSQLJDBC {
public static void main(String[] args) {
try {
Connection connection = DriverManager.getConnection("jdbc:postgresql://localhost:5432/yourdatabase", "yourusername", "yourpassword");
// Perform database operations
} catch (SQLException e) {
System.err.println(e.getMessage());
}
}
}
In this example, we use the DriverManager
class to establish a connection to the PostgreSQL database running on localhost
at port 5432
. We provide the database name, username, and password as part of the connection URL.
Utilizing Java Persistence API (JPA)
For Java applications that utilize the Java Persistence API (JPA) with an ORM framework such as Hibernate, configuring the data source to connect to Docker PostgreSQL involves setting the connection properties in the persistence.xml
or application properties file. An example configuration for a JPA data source using Hibernate is shown below:
<property name="javax.persistence.jdbc.url" value="jdbc:postgresql://localhost:5432/yourdatabase"/>
<property name="javax.persistence.jdbc.user" value="yourusername"/>
<property name="javax.persistence.jdbc.password" value="yourpassword"/>
Here, we specify the JDBC URL, database username, and password as properties for the JPA data source.
By connecting our Java application to the Docker PostgreSQL instance using JDBC or JPA, we can seamlessly integrate the database into our local testing workflow.
Cleaning up
Once testing is complete, it's important to clean up the Docker PostgreSQL container to free up resources and avoid cluttering the local environment. Use the following command to stop and remove the container:
docker stop postgresql-container
docker rm postgresql-container
Closing the Chapter
In conclusion, leveraging Docker for setting up a PostgreSQL instance for local testing of Java applications provides a portable, consistent, and efficient approach. By following the steps outlined in this post, you can master running Docker PostgreSQL like a pro and ensure a reliable testing environment for your Java projects.
Now that you have the knowledge and tools to run Docker PostgreSQL for local testing, go ahead and streamline your testing process with confidence! Happy coding!
Remember, the ability to spin up isolated, reproducible PostgreSQL instances locally using Docker provides an ideal environment for reliable testing of Java applications. Cheers to mastering local testing with Docker PostgreSQL like a pro!