Boost Your Backlog: 7 Keys to Valuing Features Right
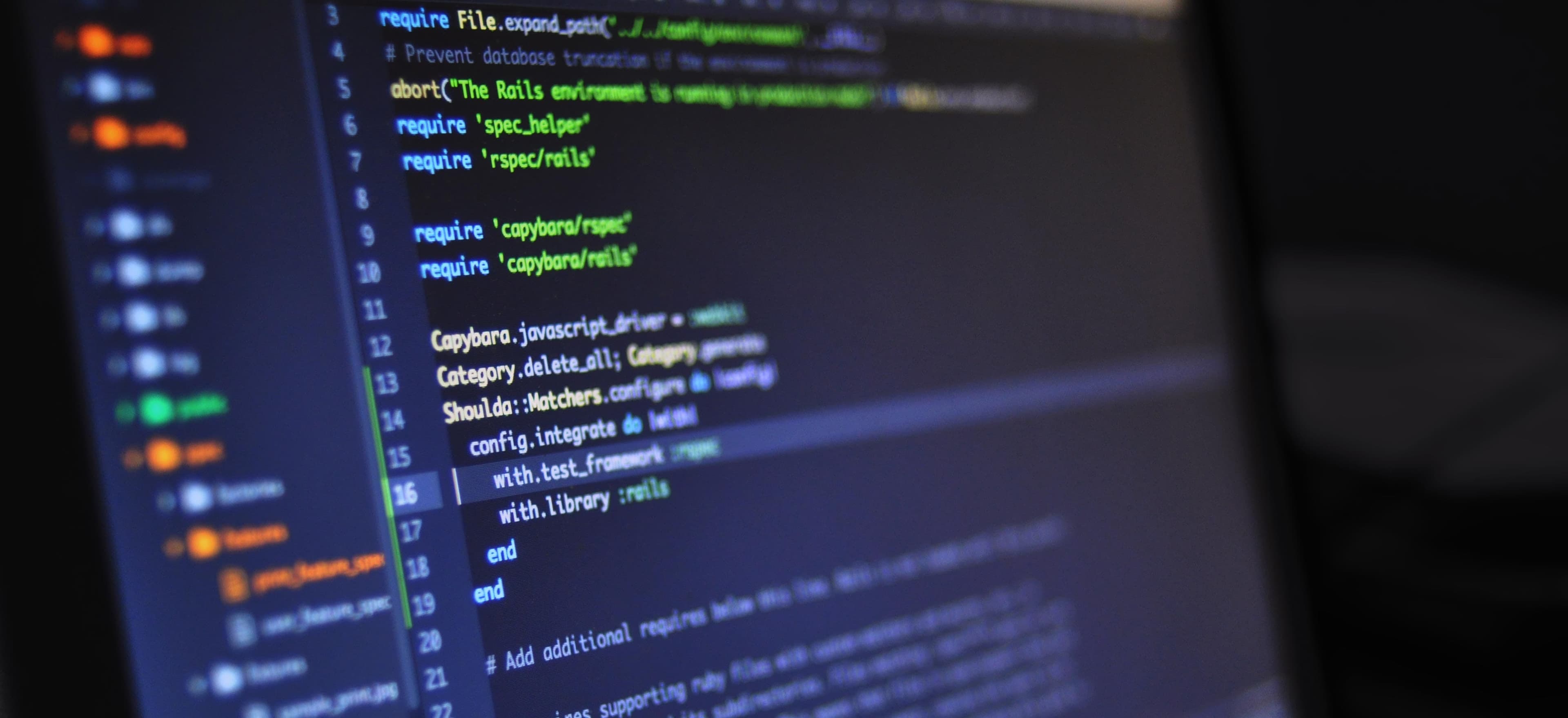
- Published on
Boost Your Backlog: 7 Keys to Valuing Features Right
As a Java developer, you understand the importance of prioritizing features and enhancements in your backlog. Prioritization ensures that you and your team are delivering value with each sprint and iteration. However, the process of valuing features can often be challenging and subjective. In this blog post, we'll explore 7 keys to valuing features right in your Java projects, helping you make informed decisions and deliver impactful results.
1. Understand User Stories and Business Value
User stories are at the heart of Agile development. Understanding the user perspective and the business value of a feature is crucial in determining its priority. By collaborating closely with product owners and stakeholders, you can gain a clear understanding of the impact each feature will have on the end users and the business goals.
When implementing user stories in Java, utilizing frameworks such as Spring MVC can streamline the process, allowing you to focus more on the business logic of the features.
@RequestMapping("/user")
public class UserController {
@Autowired
private UserService userService;
@GetMapping("/{id}")
public User getUserById(@PathVariable Long id) {
return userService.getUserById(id);
}
// Other methods
}
2. Measure Effort and Complexity
Estimating the effort and complexity of implementing a feature is essential for prioritization. Techniques such as story points and planning poker can help your team assess and align on the effort required for each feature. Understanding the technical complexity, dependencies, and potential risks associated with a feature allows for more accurate prioritization.
In Java development, utilizing tools like JIRA for backlog management and sprint planning can facilitate the estimation process and provide transparency across the team.
// Sample JIRA user story with story points
public class UserStory {
private String title;
private int storyPoints;
// Other attributes and methods
}
3. Leverage Data and Analytics
Data-driven decision making is instrumental in valuing features. By analyzing user metrics, feedback, and usage patterns, you can prioritize features that address the most pressing user needs or pain points. Incorporating A/B testing and user behavior analytics into the development process can further validate the impact of features.
In a Java application, integrating Google Analytics or Apache Hadoop for data collection and analysis empowers you to make informed decisions based on user insights.
// Sample integration of Google Analytics in a Java web application
public class AnalyticsService {
public void trackEvent(String eventName, Map<String, String> eventParams) {
// Implementation
}
// Other analytics methods
}
4. Validate Market Demand
Understanding market demand and competitive landscape is crucial in feature prioritization. Conducting market research, competitor analysis, and gathering customer feedback enables you to align feature development with market needs. Identifying unique selling propositions (USPs) and areas of differentiation can guide the prioritization process.
In Java, leveraging Apache Lucene or Elasticsearch for indexing and searching market data can provide valuable insights into demand and trends.
// Sample usage of Apache Lucene for market data indexing
public class MarketIndexer {
public void indexMarketData(MarketData data) {
// Indexing implementation
}
// Other indexing methods
}
5. Consider Technical Debt and Maintenance
Balancing feature development with technical debt and maintenance is essential for long-term product sustainability. Evaluating the impact of introducing new features on the codebase, system performance, and maintainability helps in making informed trade-offs. Prioritize features that not only add value but also minimize technical debt and upkeep efforts.
In Java projects, using SonarQube for continuous code quality inspection can aid in identifying and addressing technical debt proactively.
// Sample code analysis using SonarQube in a Java project
public class CodeAnalyzer {
public void analyzeCodeQuality(String sourceDirectory) {
// Analysis implementation
}
// Other analysis methods
}
6. Engage Cross-Functional Collaboration
Feature valuation is not solely a development team's responsibility. Engaging cross-functional teams, including UX designers, product managers, and QA engineers, in the valuation process provides diverse perspectives and ensures holistic decision making. Collaborative workshops and brainstorming sessions can uncover different dimensions of feature value.
In Java development, utilizing JUnit for collaborative testing and Mockito for simulating cross-functional interactions can enhance the quality and reliability of the features.
// Sample collaborative testing using JUnit and Mockito in Java
public class FeatureTest {
@Test
public void testFeatureFunctionality() {
// Test scenario with simulated interactions
}
// Other test cases
}
7. Adapt to Agile Iterative Feedback
Agile development thrives on iterative feedback and adaptation. Continuously re-evaluating feature priorities based on the outcomes of each iteration fosters a responsive and value-driven approach. Embracing the principles of inspect and adapt allows your Java team to remain adaptable and deliver features that resonate with the evolving needs of the users and the market.
In Java projects, utilizing Continuous Integration/Continuous Deployment (CI/CD) pipelines with tools like Jenkins enables seamless iteration and timely feedback incorporation.
// Sample Jenkinsfile for CI/CD in a Java project
pipeline {
agent any
stages {
stage('Build') {
steps {
// Build steps
}
}
stage('Test') {
steps {
// Test steps
}
}
stage('Deploy') {
steps {
// Deployment steps
}
}
}
}
A Final Look
Valuing features right in your Java projects is a strategic process that encompasses user perspective, technical considerations, and market dynamics. By understanding user stories, measuring effort, leveraging data, considering market demand, addressing technical debt, engaging collaboration, and adapting to iterative feedback, you can ensure that your team prioritizes features effectively and delivers impactful solutions.
Prioritization is not a one-time activity, but a continuous journey that requires constant alignment with the evolving needs of users and the business. Embrace the keys to valuing features right and witness the positive impact on your Java projects. Happy coding!
Remember to continuously embrace the essence of Agile development and seek opportunities for enhancing your team's efficiency and stakeholder satisfaction through well-prioritized features. Happy coding, Java developers!
Remember that delivering value is the core of feature prioritization. Embrace these keys to valuing features right and make a lasting impact with your Java projects.