Unlocking JDBC: Effortless Blob & Clob Insertion Techniques
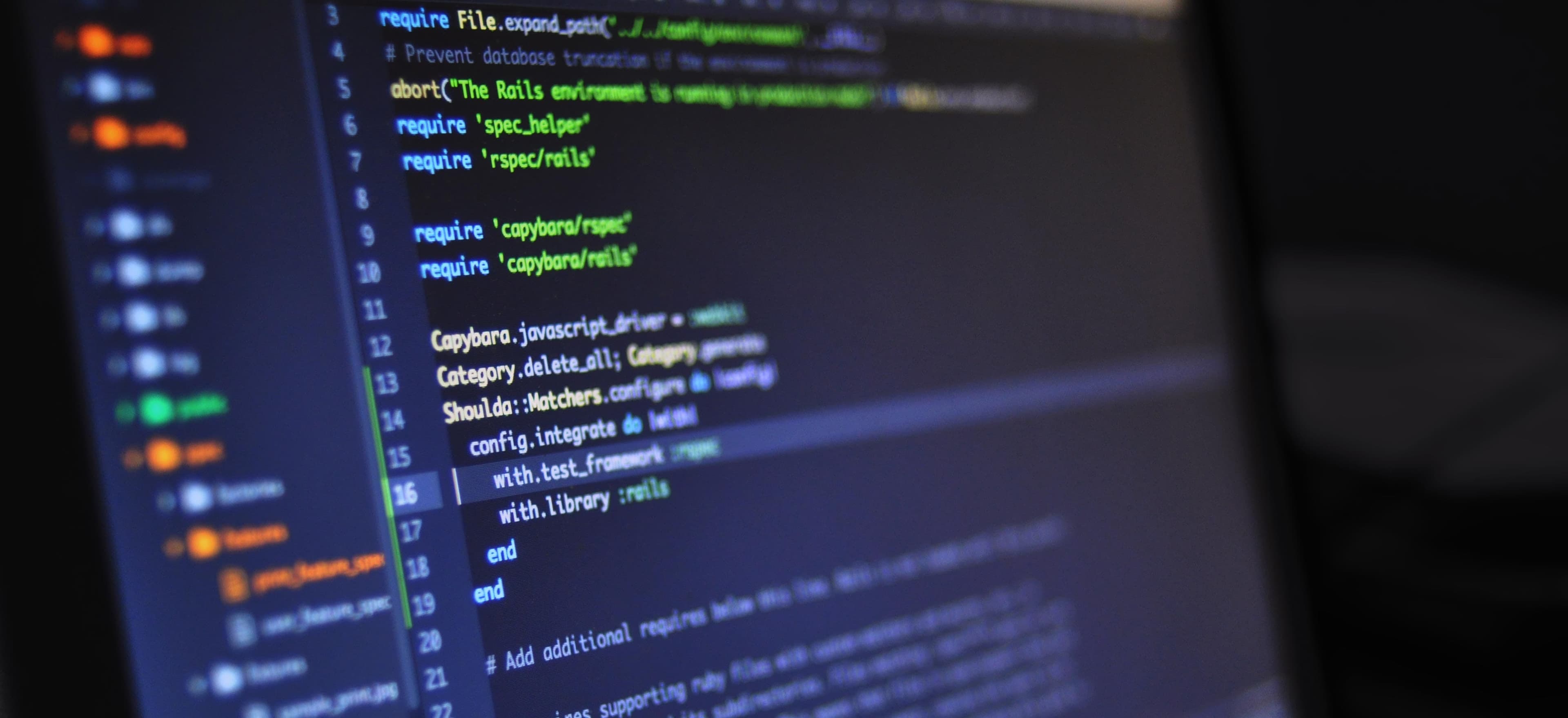
- Published on
Inserting Blobs and Clobs using JDBC in Java
Introduction It's common for Java applications to work with databases, and when dealing with large data types such as Binary Large Objects (BLOBs) and Character Large Objects (CLOBs), proper handling is crucial. In this article, we'll explore how to effortlessly insert BLOBs and CLOBs using Java Database Connectivity (JDBC). We'll delve into examples, explanations, and best practices to ensure a seamless understanding of these techniques.
Understanding BLOBs and CLOBs BLOBs are binary data types used to store large amounts of unstructured binary data, such as images, videos, and documents, in a database. On the other hand, CLOBs are character data types meant for storing large text data, including XML, JSON, and documents.
Preparation Before attempting to insert BLOBs and CLOBs into a database, ensure JDBC and the appropriate database drivers are correctly set up in your Java project.
Inserting BLOBs
Let’s start by examining how to insert BLOBs into a database. Suppose we have a table named employees
with columns id
, name
, and image
.
try (Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/company", "user", "password")) {
File imageFile = new File("path/to/image.jpg");
try (PreparedStatement statement = connection.prepareStatement("INSERT INTO employees (id, name, image) VALUES (?, ?, ?)")) {
statement.setInt(1, 1);
statement.setString(2, "John Doe");
statement.setBlob(3, new FileInputStream(imageFile));
statement.executeUpdate();
}
}
In the above code:
- We establish a connection to the database.
- We create a
File
object pointing to the image file. - We prepare a statement with an SQL query to insert data into the
employees
table. - We set the values for
id
andname
usingsetInt()
andsetString()
, respectively. - We insert the image as a BLOB using
setBlob()
after reading it as aFileInputStream
. - We execute the statement to insert the data.
It's essential to handle exceptions and close resources properly, as demonstrated with the try-with-resources construct.
Inserting CLOBs
Next, let's dive into inserting CLOBs into the database. Consider a table documents
with columns id
and content
.
try (Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/company", "user", "password")) {
String documentContent = "Lorem ipsum dolor sit amet, consectetur adipiscing elit...";
try (PreparedStatement statement = connection.prepareStatement("INSERT INTO documents (id, content) VALUES (?, ?)")) {
statement.setInt(1, 1);
statement.setCharacterStream(2, new StringReader(documentContent));
statement.executeUpdate();
}
}
In the aforementioned snippet:
- We establish a connection to the database.
- We create a
String
containing the document content. - We prepare a statement to insert data into the
documents
table. - We set the
id
usingsetInt()
. - We insert the document content as a CLOB using
setCharacterStream()
after converting it to aStringReader
. - We execute the statement to insert the data.
Why use setBlob() and setCharacterStream()?
The setBlob()
and setCharacterStream()
methods are crucial when inserting BLOBs and CLOBs. They allow us to set the corresponding data types in the prepared statement. setBlob()
accepts a Blob
object or an InputStream
, enabling the insertion of BLOBs, while setCharacterStream()
accepts a Reader
object or a String
, facilitating the insertion of CLOBs.
Best Practices
- Parameterized Queries: Always use parameterized queries to prevent SQL injection attacks and improve code reusability.
- Exception Handling: Properly handle exceptions to maintain the integrity of the application.
- Resource Management: Utilize try-with-resources for managing database connections and statements to ensure timely release of resources.
Conclusion Inserting BLOBs and CLOBs using JDBC in Java is integral for applications handling large data types. By understanding the methods, examples, and best practices outlined in this article, you can seamlessly incorporate BLOBs and CLOBs into your database operations with confidence and precision.
In conclusion, incorporating BLOBs and CLOBs using JDBC can be straightforward and effective if the appropriate methods and precautions are applied. As showcased in the examples, utilizing setBlob()
and setCharacterStream()
enables seamless insertion of BLOBs and CLOBs into a database, while adhering to best practices such as parameterized queries, exception handling, and resource management ensures the reliability and security of the application.
Now, armed with the insights and practical knowledge presented here, you can confidently navigate the intricacies of working with BLOBs and CLOBs using JDBC in your Java applications. Happy coding!
Resources
- Java Database Connectivity (JDBC)
- JDBC Drivers
- MySQL Documentation
Checkout our other articles