Scaling Woes: Navigating Fallacies in Distributed Computing
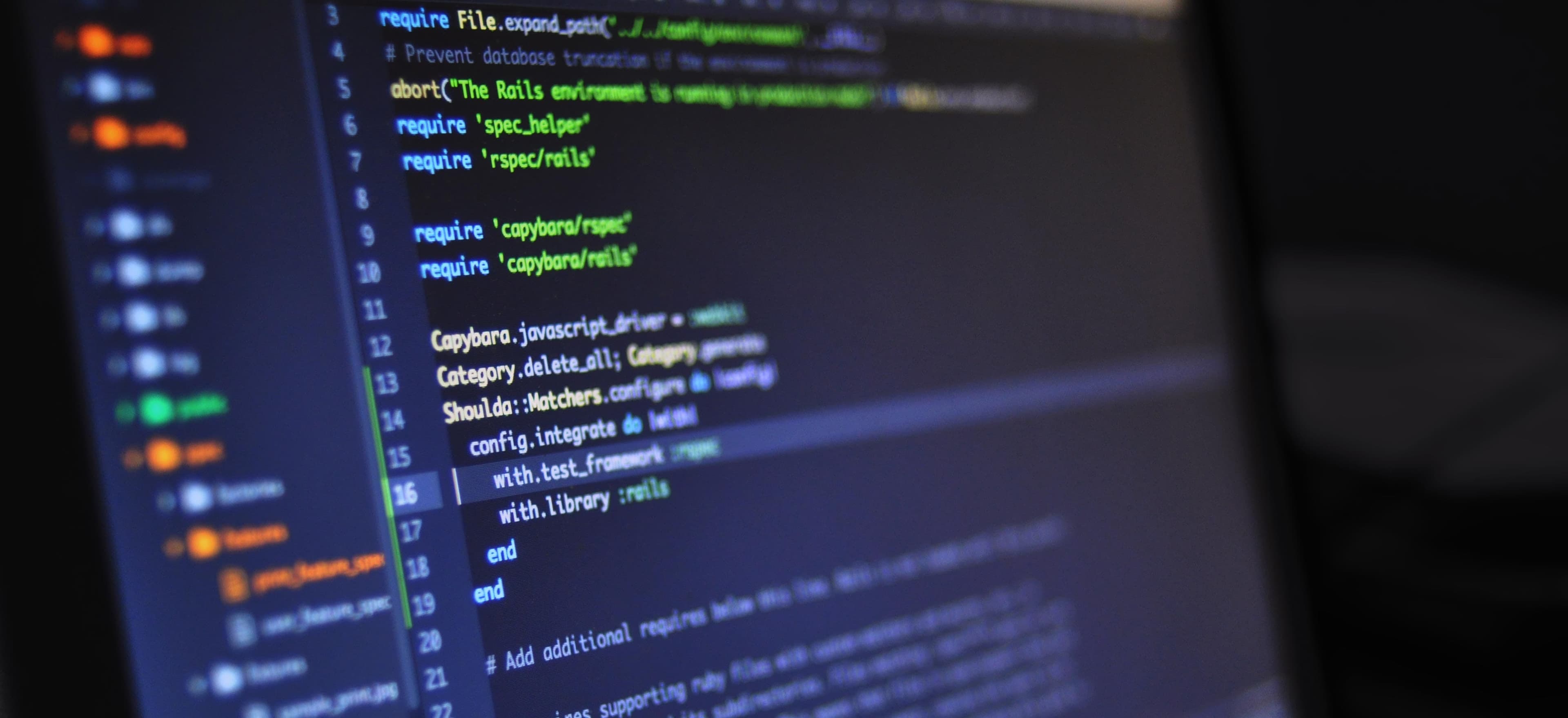
- Published on
Navigating Fallacies in Distributed Computing with Java
Distributed computing has become an integral part of modern software systems, allowing for scalability, fault tolerance, and enhanced performance. However, it also introduces a unique set of challenges that every developer must be aware of. In this blog post, we'll explore the common pitfalls, or fallacies, in distributed computing and discuss how to navigate them using Java.
The 8 Fallacies of Distributed Computing
Peter Deutsch's "The Fallacies of Distributed Computing Explained" outlines eight fallacies that are commonly assumed by developers when building distributed systems. These fallacies are:
- The network is reliable
- Latency is zero
- Bandwidth is infinite
- The network is secure
- Topology doesn't change
- There is one administrator
- Transport cost is zero
- The network is homogeneous
These fallacies are a foundation for understanding the challenges of distributed computing. Let's discuss how we can address these fallacies when developing distributed systems in Java.
Handling Network Reliability in Java
In a distributed system, network failures are inevitable. To address the fallacy that "the network is reliable," Java provides robust libraries for building fault-tolerant systems. For example, using the java.util.concurrent
package, we can implement mechanisms such as thread pools and executor services to manage network-related tasks and handle failures gracefully.
ExecutorService executor = Executors.newFixedThreadPool(10);
executor.submit(() -> {
// perform network-related tasks
});
By utilizing Java's concurrency utilities, we can design our system to withstand network hiccups and ensure reliability even in the face of intermittent failures.
Dealing with Latency and Bandwidth Constraints
Contrary to the fallacy that "latency is zero" and "bandwidth is infinite," real-world networks impose constraints on communication. In Java, we can mitigate the impact of latency and bandwidth limitations by leveraging asynchronous programming techniques. The CompletableFuture
class in Java facilitates non-blocking, asynchronous operations, allowing our system to make efficient use of network resources without being bound by latency.
CompletableFuture.supplyAsync(() -> {
// perform network-bound operation
}, executor)
.thenAccept(result -> {
// handle the result asynchronously
});
By embracing asynchronous paradigms in Java, we can effectively address the fallacies of zero latency and infinite bandwidth, leading to more responsive and resource-efficient distributed systems.
Ensuring Network Security and Adaptability
The fallacy that "the network is secure" emphasizes the need to incorporate robust security measures in distributed systems. With Java's extensive set of security features, including the Java Secure Socket Extension (JSSE) for encrypted communication and the Java Authentication and Authorization Service (JAAS) for access control, we can bolster the security of our networked applications.
Additionally, to tackle the fallacy that "topology doesn't change," we can utilize Java's support for dynamic discovery and configuration of network nodes. Libraries such as Apache ZooKeeper or Consul can be employed to build adaptable, resilient systems that gracefully handle changes in network topology.
Managing System Administration and Transport Costs
The assumption of "one administrator" and "zero transport cost" underscores the importance of efficient system administration and network resource management. Java's extensive ecosystem offers tools like JMX (Java Management eXtensions) for monitoring and managing distributed applications, as well as frameworks like Apache Kafka for cost-effective, high-throughput message transportation.
By harnessing these Java-based solutions, we can proactively address the fallacies related to system administration and transport costs, leading to more streamlined and economical distributed systems.
Addressing Network Heterogeneity
The fallacy that "the network is homogeneous" overlooks the inherent diversity in network environments. Java's platform independence and the "write once, run anywhere" principle empower developers to build distributed systems that can seamlessly operate across heterogeneous network infrastructures. By adhering to Java's portability and abstraction layers, we can mitigate the challenges posed by network heterogeneity and ensure consistent behavior across diverse environments.
Embracing Resilience and Realism in Distributed Systems
In conclusion, while the fallacies of distributed computing serve as cautionary reminders, Java equips developers with a comprehensive toolkit to navigate these challenges effectively. From fault-tolerant networking capabilities to robust security frameworks, Java empowers us to build distributed systems that embrace resilience and realism in the face of distributed computing fallacies.
By understanding these fallacies and leveraging Java's features, we can architect distributed systems that are well-equipped to thrive in the dynamic and interconnected landscape of modern software infrastructure.
Java Concurrency Utilities Apache ZooKeeper
Remember, awareness of fallacies leads to robust, reliable, and resilient systems. Happy coding with distributed systems in Java!
Checkout our other articles