Preventing Data Breach: Thwarting Hash Length Extension
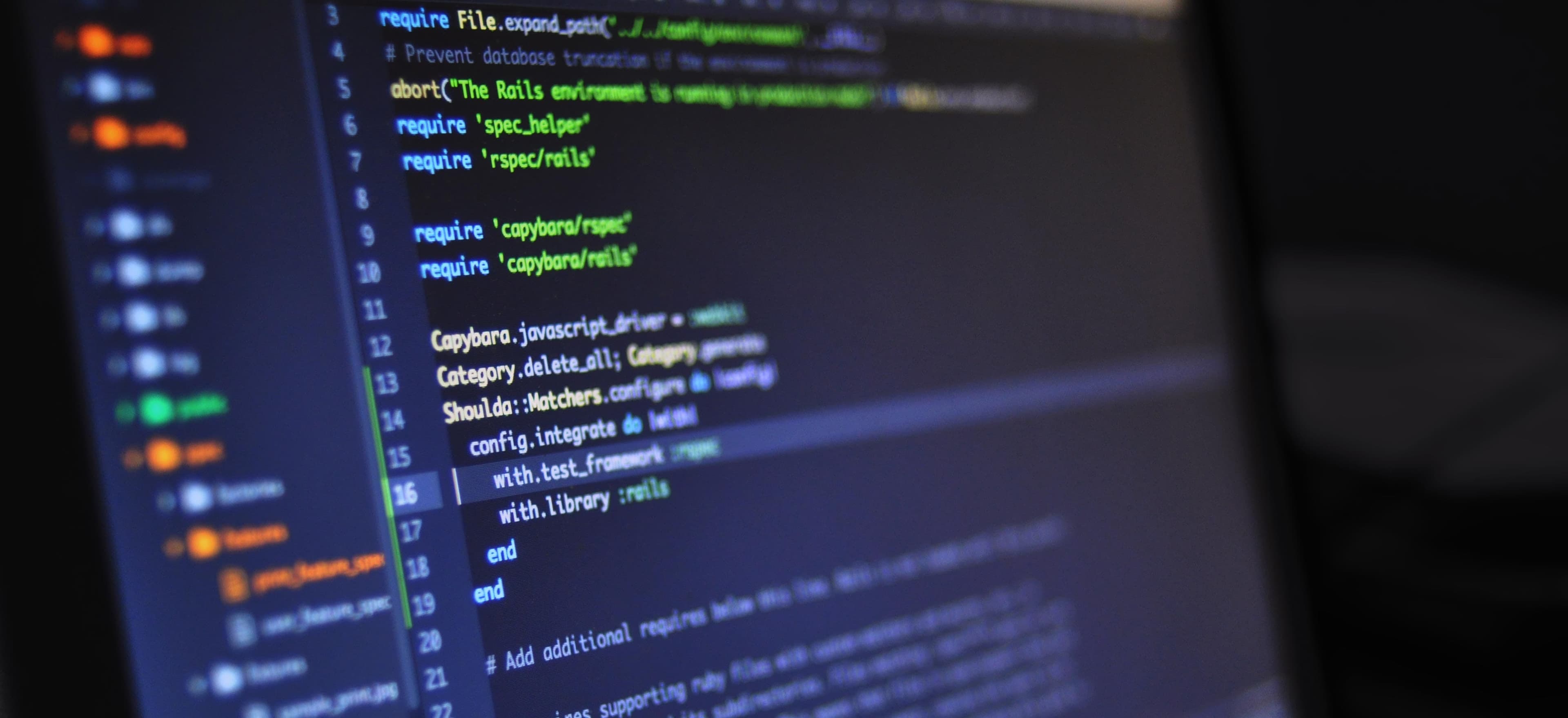
- Published on
Preventing Data Breach: Thwarting Hash Length Extension
In today's digital landscape, securing sensitive information is of paramount importance. Hash functions play a crucial role in ensuring the integrity of data. However, the threat of hash length extension attacks looms large, posing a significant risk to data security. In this blog post, we'll delve into the concept of hash length extension attacks, understand the underlying principles, and explore how to prevent them in Java.
Understanding Hash Length Extension Attacks
Hash length extension attacks exploit the deterministic nature of hash functions, allowing an attacker to append data to the original input without knowing the secret key. In essence, if the hash of a message and the length of the message are known, an attacker can extend the message without possessing the original message or the secret key.
Vulnerable Hash-Based Authentication
One common scenario where hash length extension attacks pose a threat is in hash-based message authentication code (HMAC). Without proper mitigation, an attacker could manipulate the message and its hash, leading to unauthorized access or data corruption.
Mitigating Hash Length Extension Attacks in Java
Java provides robust mechanisms to defend against hash length extension attacks. Let's explore some best practices and techniques to thwart these attacks.
1. Using HMAC with Secure Hash Algorithms
When implementing message authentication in Java, it's crucial to use strong and secure hash algorithms such as SHA-256 or SHA-512 in combination with HMAC. This ensures that the integrity of the message is preserved, making it resistant to length extension attacks.
import javax.crypto.Mac;
import javax.crypto.spec.SecretKeySpec;
import java.security.NoSuchAlgorithmException;
import java.security.InvalidKeyException;
public class HmacUtil {
public static byte[] calculateHmac(byte[] key, byte[] message)
throws NoSuchAlgorithmException, InvalidKeyException {
Mac hmac = Mac.getInstance("HmacSHA256");
SecretKeySpec secretKey = new SecretKeySpec(key, "HmacSHA256");
hmac.init(secretKey);
return hmac.doFinal(message);
}
}
In the above example, the calculateHmac
method uses the HMAC algorithm with SHA-256 for message authentication. By employing strong cryptographic primitives, the vulnerability to hash length extension attacks is significantly reduced.
2. Verifying Message Integrity
In scenarios where message integrity is critical, Java's cryptographic libraries offer methods to verify the integrity of the received message. By comparing the computed HMAC with the received HMAC, any tampering or manipulation can be detected, mitigating the risk of hash length extension attacks.
public class HmacVerifier {
public static boolean verifyHmac(byte[] key, byte[] message, byte[] receivedHmac)
throws NoSuchAlgorithmException, InvalidKeyException {
byte[] computedHmac = HmacUtil.calculateHmac(key, message);
return MessageDigest.isEqual(computedHmac, receivedHmac);
}
}
The verifyHmac
method compares the computed HMAC with the received HMAC, utilizing a constant-time comparison to prevent timing attacks. This mitigates the risk of hash length extension attacks by ensuring the integrity of the message.
3. Utilizing Secure Random Number Generation
Java's SecureRandom
class enables the generation of cryptographically secure random numbers, which are essential for key generation and nonce creation. By utilizing secure random number generation, the predictability of HMAC outputs is mitigated, thereby enhancing resistance against hash length extension attacks.
import java.security.SecureRandom;
public class SecureRandomUtil {
public static byte[] generateRandomBytes(int length) {
SecureRandom secureRandom = new SecureRandom();
byte[] randomBytes = new byte[length];
secureRandom.nextBytes(randomBytes);
return randomBytes;
}
}
The generateRandomBytes
method leverages SecureRandom
to obtain cryptographically secure random bytes, which are indispensable for mitigating predictability and thwarting hash length extension attacks.
The Last Word
Hash length extension attacks pose a significant threat to data integrity and security. By understanding the underlying principles and leveraging appropriate techniques in Java, such as using HMAC with secure hash algorithms, verifying message integrity, and utilizing secure random number generation, developers can effectively thwart these attacks and bolster data protection. It's imperative to stay abreast of emerging security threats and adhere to best practices to fortify the resilience of applications against evolving attack vectors.
In conclusion, mitigating hash length extension attacks in Java requires a multifaceted approach, encompassing robust cryptographic primitives, secure coding practices, and a comprehensive understanding of the threat landscape.
Additional Resources
To further explore cryptographic best practices and security measures in Java, consider referring to the following resources:
- Java Cryptography Architecture
- OWASP Java Cryptography Libraries
By leveraging these resources and honing the skills necessary to implement secure cryptographic solutions in Java, developers can bolster the robustness of their applications and safeguard against emerging security threats.
Checkout our other articles