Overcoming Friction in Software Development: A Guide
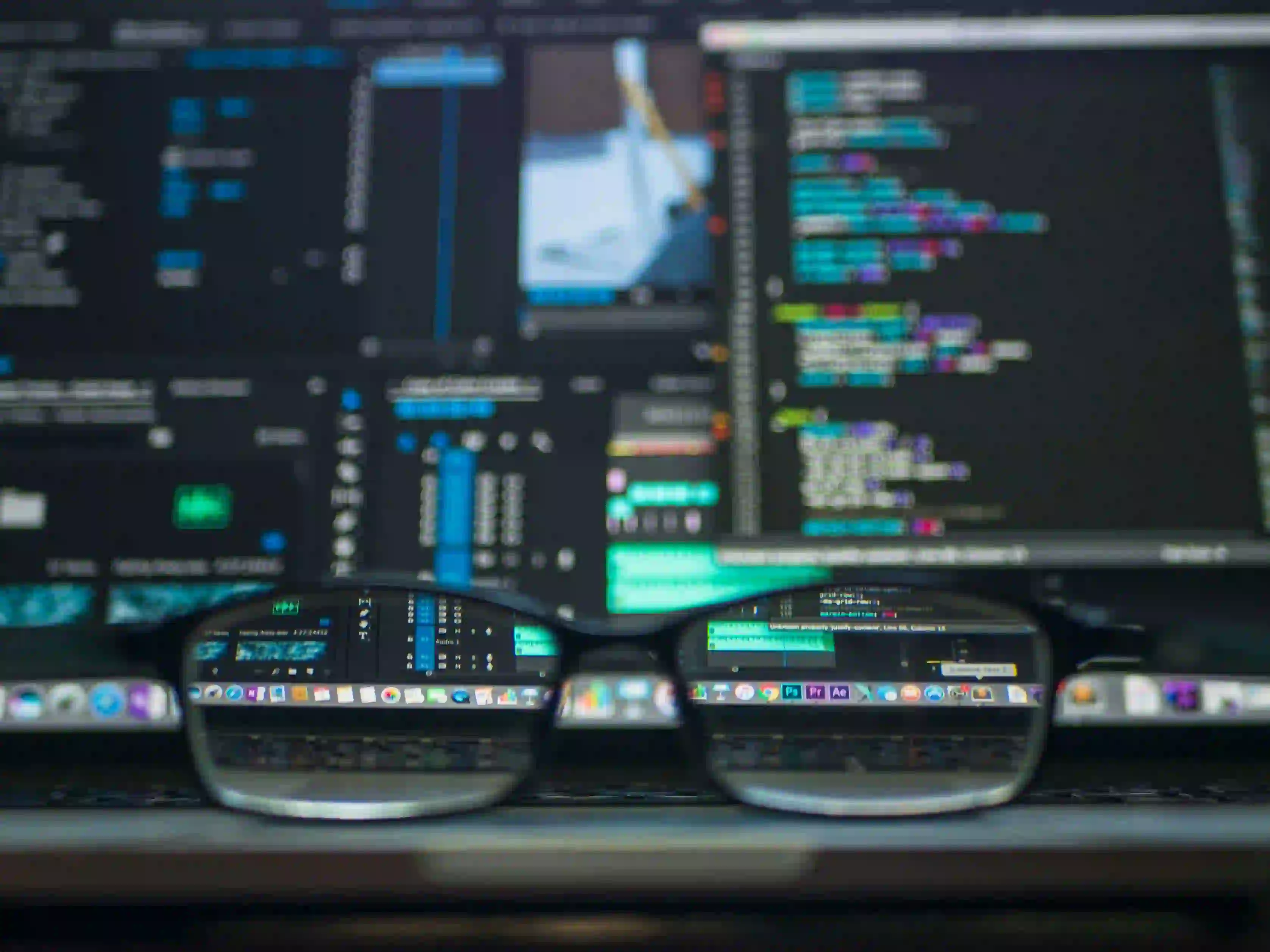
Overcoming Friction in Software Development: A Guide
Software development is a complex and demanding process. With various stages like planning, coding, testing, and deployment, it's no surprise that friction can arise, slowing down progress and hindering productivity. In this blog post, we'll explore the concept of friction in software development, its common sources, and most importantly, how Java developers can overcome it.
Understanding Friction in Software Development
Friction in software development refers to any obstacles that impede the smooth flow of the development process. These obstacles can take many forms, including technical challenges, miscommunication, unclear requirements, and inefficient processes. Left unaddressed, friction can lead to delays, quality issues, and overall project failure.
One of the primary goals of a software development team is to minimize or eliminate friction to ensure efficient, high-quality output. Java developers, in particular, face unique challenges that require specific strategies to overcome.
Common Sources of Friction for Java Developers
1. Outdated Dependencies
Java's extensive ecosystem relies heavily on third-party libraries and frameworks. When these dependencies become outdated, it can lead to compatibility issues, security vulnerabilities, and performance bottlenecks.
2. Ineffective Collaboration
In a team-based environment, ineffective collaboration can introduce friction at various stages of the development process. Misaligned goals, poor communication, and lack of coordination can all contribute to this problem.
3. Poor Code Quality
Maintaining high code quality is essential for Java developers. Friction arises when code becomes complex, hard to understand, or lacks proper documentation, leading to debugging and maintenance challenges.
4. Inefficient Build and Deployment Processes
Slow build times, cumbersome deployment procedures, and unreliable testing environments can all slow down development cycles and create unnecessary friction.
Overcoming Friction in Java Development
1. Dependency Management with Gradle
plugins {
id 'java'
}
repositories {
mavenCentral()
}
dependencies {
implementation 'org.springframework:spring-web:5.3.8'
implementation 'com.google.guava:guava:30.1-jre'
}
Use a modern build tool like Gradle to manage dependencies efficiently. Keep dependencies updated to minimize compatibility issues and security vulnerabilities.
2. Embracing Agile Methodologies
Embrace agile methodologies such as Scrum or Kanban to foster effective collaboration within the development team. Regular stand-up meetings, sprint planning, and retrospectives can help align team members towards common goals and improve communication.
3. Code Quality with Checkstyle
public class Example {
private int value;
public int getValue() {
return value;
}
}
Leverage tools like Checkstyle to enforce code quality standards. Automated checks for coding style, naming conventions, and potential bugs can help maintain clean, readable code.
4. Automation with Jenkins
Set up continuous integration and deployment pipelines using tools like Jenkins. Automation streamlines build processes, tests code changes, and deploys applications, reducing manual errors and accelerating feedback loops.
In Conclusion, Here is What Matters
Friction is an inevitable part of the software development process, but with the right strategies, Java developers can overcome it. By focusing on efficient dependency management, improved collaboration, code quality, and automation, teams can mitigate friction and deliver high-quality software effectively.
Incorporate these techniques into your Java development workflow to streamline your processes and elevate the overall productivity of your team. Remember, overcoming friction is not a one-time effort—it requires continuous attention and adaptation to the evolving landscape of software development.