Demystifying Spring: Navigating Classic Web Architecture
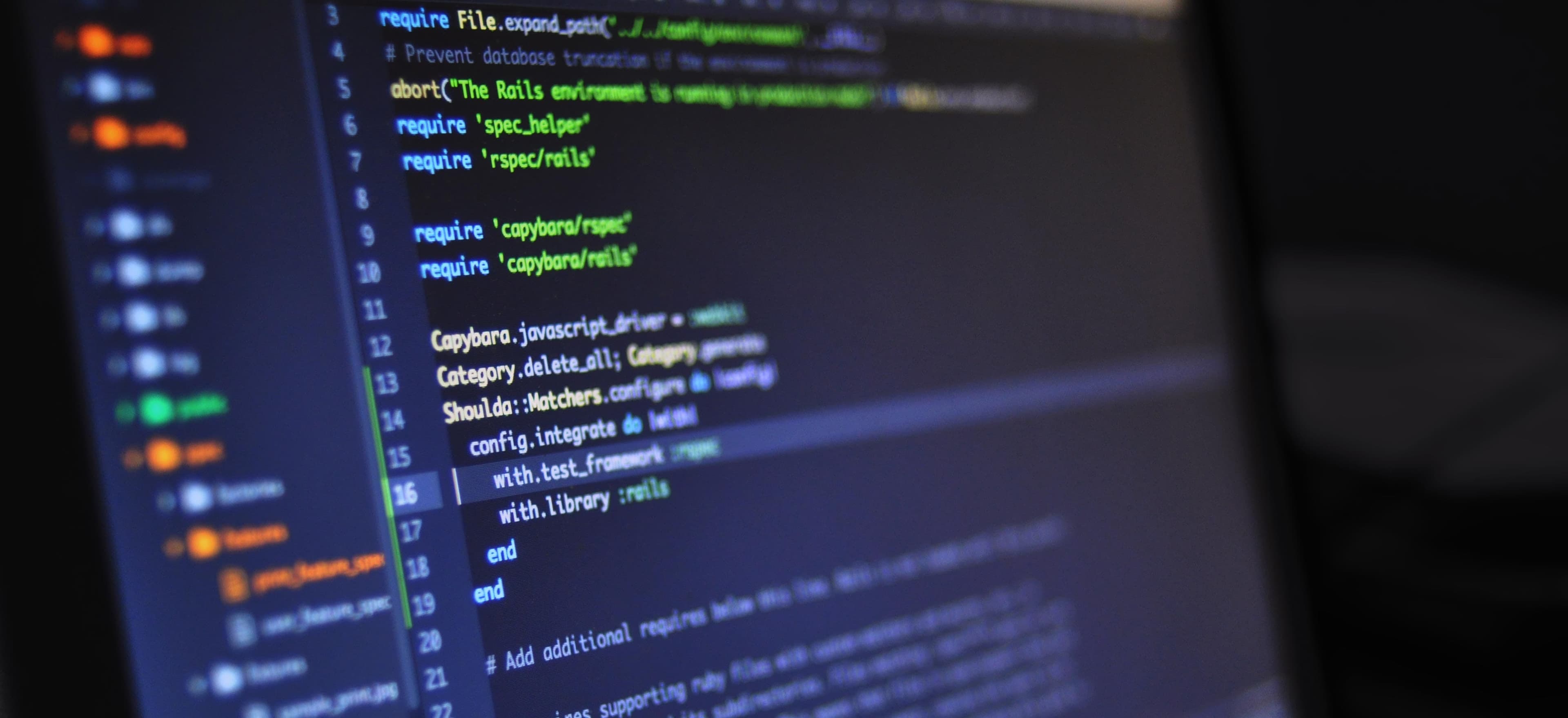
- Published on
Demystifying Spring: Navigating Classic Web Architecture
In the realm of Java development, Spring has established itself as the go-to framework for building robust, scalable, and maintainable web applications. With its vast ecosystem of modules, Spring offers a comprehensive toolkit for addressing various architectural concerns such as inversion of control, aspect-oriented programming, and containerization. In this blog post, we will delve into the classic web architecture principles in the context of Spring, unraveling the intricacies of controllers, services, and data access layers.
The MVC Paradigm and Spring Framework
At the heart of web development lies the Model-View-Controller (MVC) architectural pattern, which facilitates the separation of concerns between the data model, the presentation of data, and the user interactions. Spring, true to its nature, provides robust support for building applications following the MVC paradigm. Let's dissect each component of MVC within the Spring framework.
Model: Defining the Data Model
In the context of Spring, the model constitutes the data structure and business logic. This is often represented by POJOs (Plain Old Java Objects) or entities when working with persistence. The @Entity
annotation in Spring Data JPA, for instance, signifies that a POJO class represents an entity that can be persisted to a data store.
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String firstName;
private String lastName;
// Getters and setters
}
The underlying principle here is the encapsulation of data and behavior within the model, adhering to the domain-driven design philosophy.
View: Handling User Interface
Views in the MVC architecture are responsible for presenting the data to the users. In a Spring web application, these views often take the form of Thymeleaf or JSP templates. Thymeleaf, a modern server-side Java template engine, blends well with Spring MVC, offering a familiar and natural way of creating dynamic web pages.
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>Spring Blog</title>
</head>
<body>
<h1>Welcome, <span th:text="${user.firstName}"></span>!</h1>
</body>
</html>
Thymeleaf's integration with Spring's model attributes simplifies the process of binding and rendering data in the user interface.
Controller: Managing User Interactions
Controllers play a pivotal role in handling user requests and orchestrating the communication between the model and view. Within Spring's MVC framework, annotating a class with @Controller
designates it as a controller, while methods annotated with @RequestMapping
handle specific HTTP requests.
@Controller
@RequestMapping("/users")
public class UserController {
@Autowired
private UserService userService;
@GetMapping("/{id}")
public String getUserDetails(@PathVariable("id") Long userId, Model model) {
User user = userService.getUserById(userId);
model.addAttribute("user", user);
return "userDetails";
}
// More handler methods
}
By using annotations, Spring eliminates the need for cumbersome XML configurations, making the development process more streamlined and readable.
Service Layer: Business Logic Abstraction
In traditional MVC, the model often encapsulates the business logic. However, as applications grow in complexity, it becomes imperative to extract and centralize the business logic. This is where the service layer comes into play. In Spring, the service layer typically consists of classes annotated with @Service
, each encapsulating a distinct set of functionalities. The service layer acts as a bridge between the controllers and the data access layer, abstracting the business logic into reusable components.
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
public User getUserById(Long id) {
// Business logic for fetching user by ID from the repository
return userRepository.findById(id);
}
// More business operations
}
The clear separation of concerns facilitated by the service layer not only enhances code maintainability but also simplifies unit testing by isolating the business logic from the presentation layer.
Data Access Layer: Interaction with the Database
In a classic web architecture, the data access layer is responsible for interacting with the underlying database, encapsulating the CRUD (Create, Read, Update, Delete) operations. Spring provides extensive support for data access through its powerful 'Spring Data' modules. When working with relational databases, Spring Data JPA, an implementation of the Java Persistence API (JPA), offers a repository-centric approach for performing database operations.
public interface UserRepository extends JpaRepository<User, Long> {
// Custom query methods if needed
}
Defining a JPA repository interface in Spring Data JPA might seem deceptively simple at first glance. However, through powerful method signature inference, Spring Data JPA empowers developers to declare query methods directly within the repository interfaces. This capability significantly reduces the amount of boilerplate code when dealing with basic database interactions.
Wrapping Up
Understanding and effectively utilizing the classic web architecture within the Spring framework sets the stage for building robust and maintainable web applications. By adhering to the MVC paradigm, leveraging the service layer for business logic abstraction, and harnessing Spring's support for data access, developers can navigate the complexities of web application development with confidence.
As you embark on your journey through Spring's classic web architecture, remember to embrace the modularity and extensibility that Spring offers, empowering you to tackle even the most intricate of web development challenges.
Embrace the classics, embrace Spring.
For further reading on Spring framework and classic web architecture, dive into the resources below:
Happy coding!
Checkout our other articles