Mastering State Persistence in IntelliJ Plugins
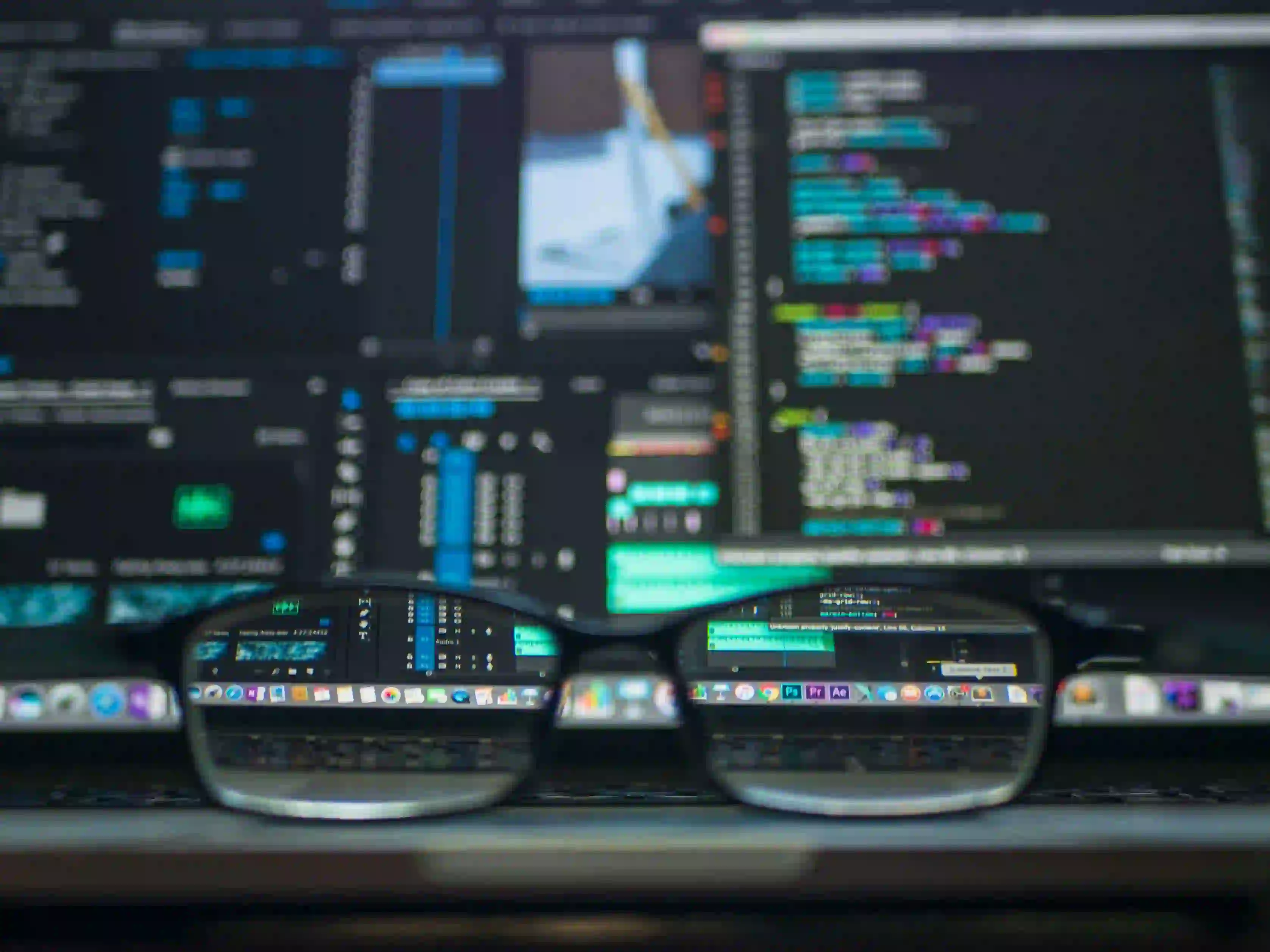
Mastering State Persistence in IntelliJ Plugins
When developing IntelliJ plugins, it's essential to understand how to manage and persist the state of your plugin. State persistence ensures that your plugin remembers user settings, preferences, and other data across sessions. In this post, we'll explore the intricacies of state persistence in IntelliJ plugins and how you can master this aspect of plugin development.
Why State Persistence is Important
State persistence is crucial for providing a seamless user experience. Imagine if your plugin's settings were reset every time a user restarted IntelliJ. This would be frustrating for users and diminish the usability of your plugin.
By mastering state persistence, you can ensure that your plugin retains important user data such as configuration settings, customizations, and any other state-related information.
Understanding the Concept of State Persistence
In the context of IntelliJ plugins, state persistence refers to the ability of a plugin to store and retrieve its state across sessions. This can include user preferences, configuration settings, last-used values, and other relevant data.
In essence, state persistence allows your plugin to remember and restore user-specific information, providing a personalized experience for each user.
Using PropertiesComponent
for Simple Key-Value Data
IntelliJ provides a simple and efficient way to persist key-value data using the PropertiesComponent
class. This class allows plugins to store and retrieve simple string key-value pairs.
import com.intellij.ide.util.PropertiesComponent;
// Storing data
PropertiesComponent.getInstance().setValue("key", "value");
// Retrieving data
String savedValue = PropertiesComponent.getInstance().getValue("key");
The PropertiesComponent
is ideal for persisting straightforward data such as user preferences, last-used values, and other similar settings. It's lightweight and easy to use, making it a great choice for simple state persistence requirements.
Managing Complex State with PersistentStateComponent
For more complex state persistence requirements, IntelliJ provides the PersistentStateComponent
interface. This interface allows plugins to manage more intricate state data by serializing and deserializing complex objects.
import com.intellij.openapi.components.PersistentStateComponent;
import com.intellij.openapi.components.State;
import com.intellij.openapi.components.Storage;
@State(
name = "MyPluginState",
storages = {
@Storage("MyPluginState.xml")
}
)
public class MyPluginState implements PersistentStateComponent<MyPluginState> {
private String customValue;
@Nullable
@Override
public MyPluginState getState() {
return this;
}
@Override
public void loadState(MyPluginState state) {
this.customValue = state.customValue;
}
// Other methods and logic for managing state
}
The PersistentStateComponent
interface allows for the serialization and deserialization of custom state objects. It provides a more structured and organized approach to managing complex plugin state.
Utilizing FileBasedStorage
In some cases, you may need to persist state data in a more structured and file-based manner. IntelliJ's FileBasedStorage
provides a solution for scenarios where storing state in a file is more appropriate.
Using FileBasedStorage
allows for more flexibility in how state data is organized and managed, making it suitable for plugins with extensive state persistence requirements.
Handling State Migration
As your plugin evolves, it's essential to consider how existing state data will be managed during updates. State migration involves handling the conversion of old state data to the new format when users update to a newer version of your plugin.
By incorporating state migration logic into your plugin, you can ensure a smooth transition for existing users when introducing changes to the plugin's state structure.
Ensuring Consistency with @State
Annotations
When utilizing state persistence components such as PersistentStateComponent
, the @State
annotation plays a crucial role in defining the state storage and versioning.
By using the @State
annotation, you can specify the name of the state, its storage format, and other relevant details. This helps maintain consistency in state persistence across different versions of your plugin.
Testing State Persistence
Proper testing of state persistence is vital to ensure the reliability and stability of your plugin. Writing tests to cover state serialization, deserialization, migration, and data retrieval scenarios helps identify and prevent potential issues related to state persistence.
By incorporating unit tests and integration tests that specifically target state persistence functionality, you can confidently validate the robustness of your plugin's state management capabilities.
Key Takeaways
Mastering state persistence in IntelliJ plugins is a fundamental aspect of developing high-quality, user-friendly extensions. By understanding the various methods and components available for state persistence, you can ensure that your plugin provides a smooth and consistent experience for users, maintaining their preferences and settings across sessions.
IntelliJ's robust API for state persistence, encompassing PropertiesComponent
, PersistentStateComponent
, FileBasedStorage
, and state migration mechanisms, empowers plugin developers to build resilient and reliable state management solutions.
As you dive into IntelliJ plugin development, remember to prioritize state persistence as a key factor in delivering a polished and professional user experience.
By implementing effective state persistence strategies, you can set your IntelliJ plugin apart as a seamless and user-centric extension within the IDE ecosystem.
Now that you've grasped the fundamentals of state persistence, consider exploring IntelliJ IDEA Plugin Development for further insights into developing robust plugins that leverage state persistence to deliver exceptional user experiences.