Why Ignoring Common Sense Hurts Code Quality
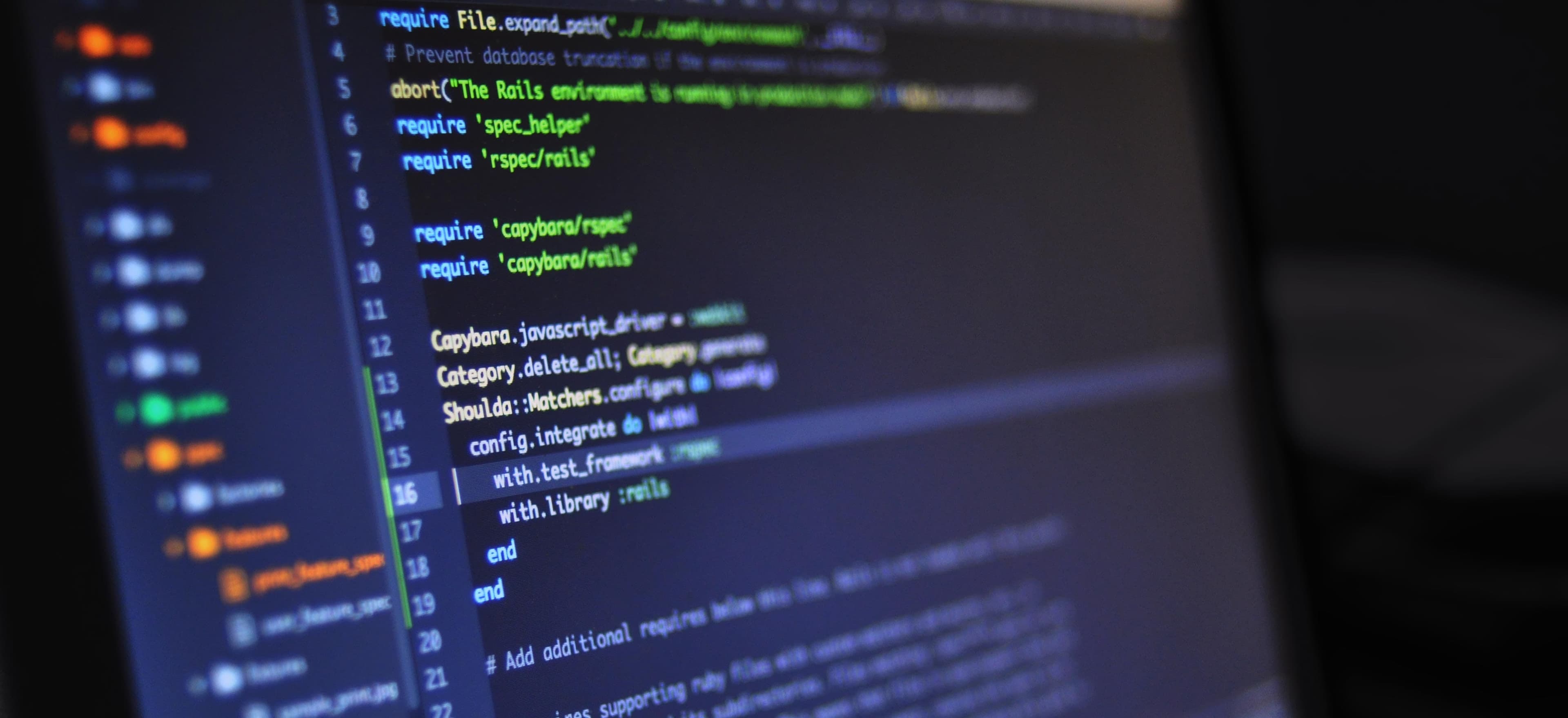
- Published on
The Importance of Common Sense in Java Programming
As a Java developer, you may have encountered situations where applying common sense becomes indispensable in writing high-quality code. While the Java language provides a robust framework for building powerful applications, it’s the application of common sense that often separates good code from great code.
In this article, we’ll explore the significance of incorporating common sense practices into Java programming. From writing clean and efficient code to debugging complex issues, common sense plays a pivotal role in every aspect of software development.
Writing Readable and Maintainable Code
Incorporating common sense into your Java code leads to writing clear, readable, and maintainable code. When naming variables, methods, and classes, choosing intuitive and descriptive names can make a significant difference in understanding the codebase. For instance, using names like getUserData()
instead of obscure names like fetch()
not only provides clarity but also enhances the code's readability.
Let's take a look at an example to illustrate the use of common sense in naming conventions:
// Not using common sense
int x = userService.retrieveDataFromDatabase();
// Using common sense
int userData = userService.fetchUserDataFromDatabase();
In the above example, the second approach using common sense clearly conveys the purpose of the method, making the code more comprehensible for other developers.
Embracing Simplicity
Java developers often encounter situations where the simplest solution is the most effective. Applying common sense dictates that we opt for simplicity over unnecessary complexity. This can manifest in various forms, such as favoring built-in language features over reinventing the wheel and avoiding convoluted logic when simpler alternatives are available.
Consider the following example, where common sense plays a crucial role in choosing a simpler approach:
// Complicated approach
if (status.equals("active") || (status.equals("pending") && userType.equals("admin"))) {
// Perform action
}
// Simpler approach using common sense
if (status.equals("active") || (status.equals("pending") && isAdminUser(userType))) {
// Perform action
}
In the second approach, the common sense dictates the use of a dedicated isAdminUser
method rather than cluttering the conditional statement with complex logic.
Efficient Resource Management
Common sense dictates that efficient resource management is vital in Java programming. Whether it’s handling file operations, database connections, or memory allocation, applying common sense leads to writing code that not only performs well but also minimizes potential resource leaks.
For instance, consider the following common sense practice for resource management when working with file I/O:
// Not using common sense - potential resource leak
FileInputStream fileInputStream = new FileInputStream("file.txt");
// Process file
fileInputStream.close(); // Might not execute in case of an exception
// Using common sense for resource management
try (FileInputStream fileInputStream = new FileInputStream("file.txt")) {
// Process file
} catch (IOException e) {
// Exception handling
}
The common sense approach ensures that the file input stream is automatically closed after its use, mitigating the risk of resource leaks.
Debugging and Troubleshooting
In the realm of debugging and troubleshooting, common sense can be an invaluable asset. When encountering issues or unexpected behavior in your Java code, applying common sense allows you to approach problems systematically and logically.
Let’s consider an example where common sense aids in identifying a null reference exception:
// Null check without common sense
if (object != null) {
// Perform operation
} else {
// Handle null case
}
// Applying common sense for null check
Objects.requireNonNull(object, "Object cannot be null");
// Perform operation
In the second approach, using common sense, we employ Objects.requireNonNull
to explicitly indicate that the object should not be null, providing a more robust and deliberate check.
Final Considerations
In the world of Java programming, the application of common sense can truly elevate the quality of your code. Whether it’s writing clean and maintainable code, embracing simplicity, managing resources efficiently, or tackling debugging challenges, common sense serves as a guiding principle for making informed decisions and crafting exceptional software solutions. By integrating common sense practices into your Java programming endeavors, you can significantly enhance the overall quality and reliability of your codebase.
Incorporating common sense into Java programming not only fosters code quality but also fosters a collaborative and consistent coding culture, benefiting the entire development team and the longevity of the software product. As you continue to refine your Java coding skills, remember that embracing common sense is not just a choice, but an essential element in crafting elegant, robust, and high-quality Java applications.
Checkout our other articles