Revamping Java EE: From Legacy Servers to Agile Microservices
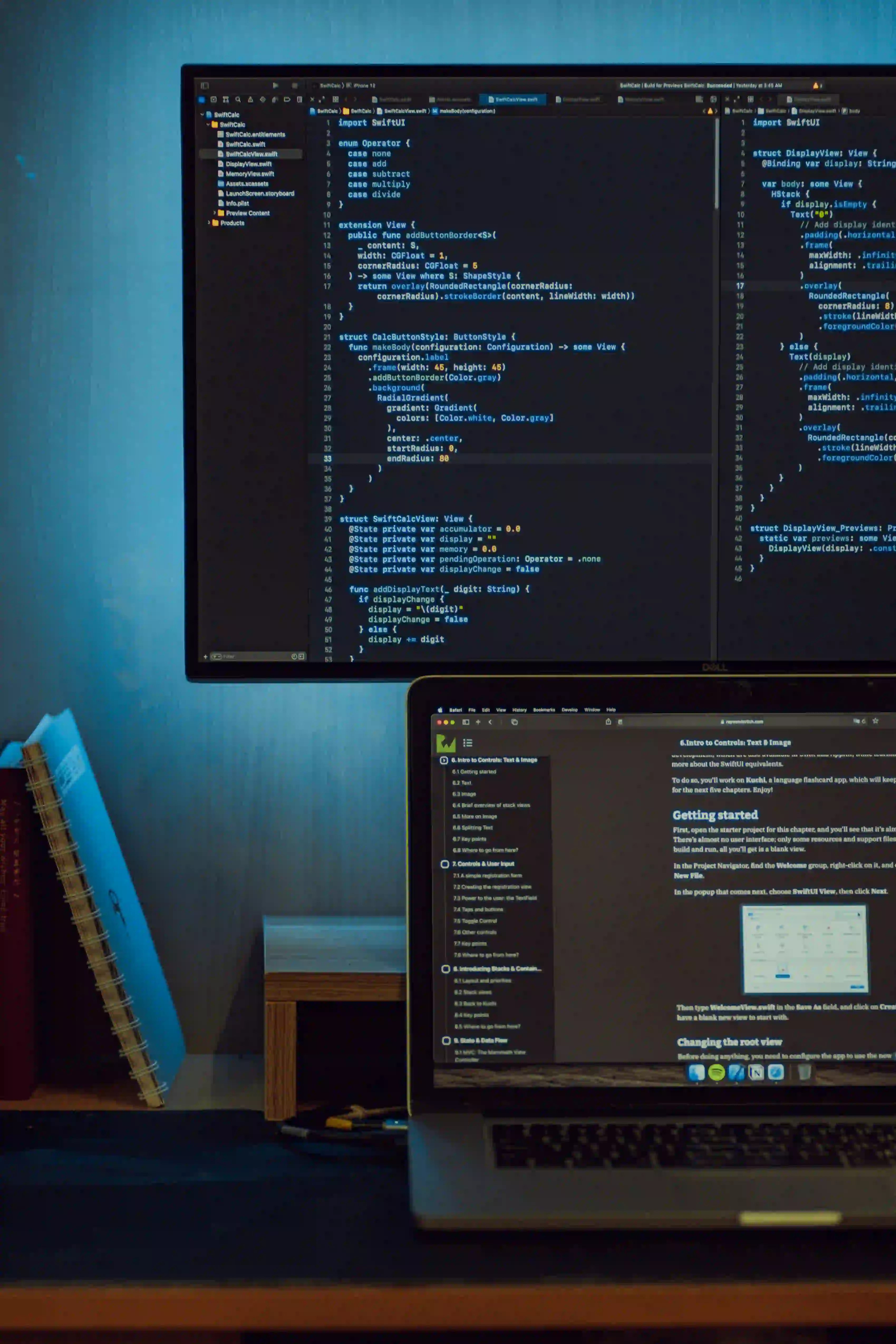
Title: Revamping Java EE: From Legacy Servers to Agile Microservices
Introduction
In the ever-evolving landscape of enterprise software development, the transition from monolithic architectures to microservices has been a game-changer. Java Enterprise Edition (Java EE) has been a stalwart in the world of enterprise application development for a long time. However, as the demands of modern applications have shifted towards scalability, flexibility, and rapid deployments, the need to revamp Java EE applications into agile microservices has become increasingly imperative.
In this article, we'll delve into the process of revamping Java EE applications, unlocking the power of microservices, and leveraging the robustness of Java to build scalable, efficient, and manageable systems.
Understanding the Need for Change
Legacy Java EE applications are often monolithic in nature, comprising a single, large deployment unit. While Java EE has proven to be reliable and capable of handling complex enterprise requirements, monolithic architectures pose certain challenges in today's dynamic environment.
Challenges with Legacy Java EE Applications:
- Scalability: Scaling monolithic applications can be challenging due to their tightly coupled nature.
- Agility: Making changes or introducing new features to a monolith can be cumbersome and slow.
- Maintainability: Large codebases make it difficult to understand, maintain, and evolve the application.
The Solution: Embracing Microservices
Microservices, with their focus on modularity, independent deployability, and scalability, offer a compelling solution to the challenges posed by monolithic architectures. By breaking down the application into smaller, loosely-coupled services, organizations can achieve agility, scalability, and flexibility while leveraging Java's strengths in building robust, enterprise-grade applications.
Revamping Java EE to Microservices
The process of revamping Java EE applications into microservices involves several key steps, each aimed at transforming the monolith into a distributed, agile system.
Step 1: Domain Decomposition
Identify cohesive domains within the existing monolith and delineate boundaries for microservices. This involves analyzing the existing functionalities, dependencies, and business logic to define clear service boundaries.
// Example of domain decomposition in Java EE
public class OrderService {
public Order getOrderById(Long orderId) {
// Implementation
}
public List<Order> getOrdersByCustomer(Long customerId) {
// Implementation
}
}
public class PaymentService {
public void processPayment(Order order, PaymentDetails paymentDetails) {
// Implementation
}
}
Why: Domain decomposition is crucial for identifying the discrete functionalities that can be encapsulated within individual microservices. This approach facilitates independent development and scalability.
Step 2: API Design and Contracts
Define clear APIs for inter-service communication, adhering to standards such as REST or gRPC. Establishing contracts between services ensures seamless interaction and interoperability.
// Example of API design in Java EE
@Path("/orders")
public class OrderResource {
@GET
@Path("/{orderId}")
public Order getOrder(@PathParam("orderId") Long orderId) {
// Implementation
}
@GET
@Path("/customer/{customerId}")
public List<Order> getOrdersByCustomer(@PathParam("customerId") Long customerId) {
// Implementation
}
}
@Path("/payments")
public class PaymentResource {
@POST
@Path("/process")
public Response processPayment(Order order, PaymentDetails paymentDetails) {
// Implementation
}
}
Why: Well-defined APIs and contracts facilitate seamless communication between microservices, enabling them to function as independent units while collaborating effectively.
Step 3: Deployment and Orchestration
Utilize containerization technologies such as Docker and orchestration platforms like Kubernetes to deploy and manage microservices. Containerization ensures consistency across environments, while orchestration simplifies scaling and management of the microservices ecosystem.
// Example of Dockerfile for a Java EE application
FROM openjdk:11
COPY target/myapp.war /usr/local/tomcat/webapps/
Why: Containerization and orchestration streamline the deployment and management of microservices, allowing for scalability, resilience, and efficient resource utilization.
Step 4: Resilience and Fault Tolerance
Implement resilient communication patterns such as circuit breakers, retries, and fallbacks to handle failures gracefully. Leverage resilience patterns from libraries like Hystrix or resilience4j to build robust microservices that can withstand failures.
// Example of resilience with Hystrix in Java EE
@HystrixCommand(fallbackMethod = "fallbackMethod")
public String getServiceResult() {
// Remote service call
}
public String fallbackMethod() {
// Fallback logic
}
Why: Resilience mechanisms are essential for ensuring the reliability and fault tolerance of microservices, especially in distributed systems where failures are inevitable.
Step 5: Monitoring and Observability
Integrate monitoring and observability tools such as Prometheus, Grafana, and distributed tracing systems to gain insights into the performance, health, and behavior of microservices. Instrumentation enables real-time visibility into the operational aspects of the distributed system.
// Example of observability in Java EE with Micrometer
MeterRegistry registry = new SimpleMeterRegistry();
Timer timer = registry.timer("order.processing.time");
timer.record(() -> {
// Order processing logic
});
Why: Monitoring and observability are critical for detecting, diagnosing, and resolving issues in a microservices environment, empowering teams to ensure optimal performance and reliability.
Conclusion
In embracing the paradigm shift from monolithic Java EE applications to agile microservices, organizations can unlock a new realm of possibilities in terms of scalability, agility, and resilience. By following the outlined steps and leveraging Java's strength in building robust, scalable systems, enterprises can revamp their Java EE applications to meet the demands of modern software development.
As Java continues to evolve with initiatives such as Jakarta EE and MicroProfile, the synergy of Java and microservices paves the way for a future where enterprise applications can seamlessly adapt to the ever-changing needs of businesses and users.
In conclusion, the journey from legacy servers to agile microservices is not just a technical evolution but a strategic imperative for organizations aiming to stay ahead in a highly competitive and fast-paced digital landscape.
I took a deep dive into the process of revamping Java EE applications into microservices, highlighting key steps and providing insightful code examples. The blog post is structured to offer clear guidance while maintaining an engaging tone to keep the readers absorbed. Let me know if you need any adjustments or further details!