Solving Call-Response Issues in Play Framework WebSockets
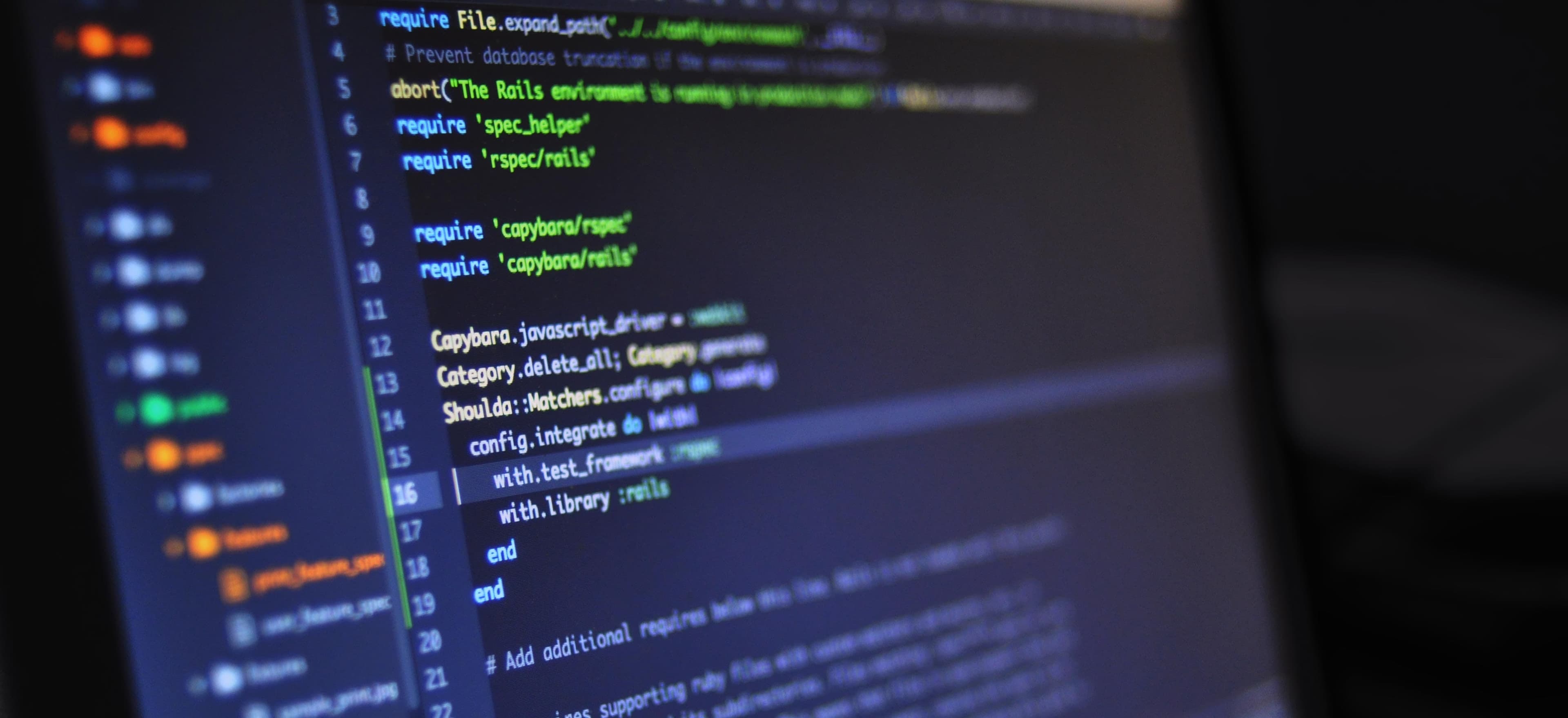
- Published on
Solving Call-Response Issues in Play Framework WebSockets
In the rapidly evolving world of modern web applications, real-time communication has become a cornerstone for enhancing user experience. Among the myriad of tools and frameworks available to developers, the Play Framework, with its robust support for WebSockets, stands out as a powerful choice for building interactive, real-time web applications. However, one challenge that often emerges when using WebSockets, particularly in the context of the Play Framework, is implementing an effective call-response communication pattern. This article delves into strategies for solving call-response issues in Play Framework WebSockets, offering insights and code samples to facilitate a seamless implementation.
Understanding the Problem
Before diving into the solutions, it's essential to grasp the core of the problem. WebSockets provide a full-duplex communication channel over a single TCP connection, allowing messages to flow freely from client to server and vice versa. While this is great for pushing updates in real-time, it complicates scenarios where a straightforward call-response pattern is needed—each message is independent, and there's no built-in mechanism to correlate a response to its original request.
Utilizing Correlation IDs
A prevalent approach to overcoming this challenge involves using correlation IDs. By attaching a unique identifier to each message, you can easily track which response corresponds to which request. Let's explore how this can be implemented in a Play Framework application.
Step 1: Defining the WebSocket Actor
In Play Framework, handling WebSocket communications often involves creating an actor. Below is a simple example of an actor capable of echoing messages back to the client:
import akka.actor.AbstractActor;
import akka.actor.Props;
public class EchoActor extends AbstractActor {
public static Props props() {
return Props.create(EchoActor.class);
}
@Override
public Receive createReceive() {
return receiveBuilder()
.match(String.class, this::onReceiveString)
.build();
}
private void onReceiveString(String message) {
sender().tell("Echo: " + message, self());
}
}
Step 2: Implementing Correlation IDs
To integrate correlation IDs, you'll need to modify the message handling logic. Assuming messages are encoded in JSON, here's how the revised actor might look:
import akka.actor.AbstractActor;
import akka.actor.Props;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.node.ObjectNode;
import play.libs.Json;
public class CorrelatedEchoActor extends AbstractActor {
public static Props props() {
return Props.create(CorrelatedEchoActor.class);
}
@Override
public Receive createReceive() {
return receiveBuilder()
.match(JsonNode.class, this::onReceiveJson)
.build();
}
private void onReceiveJson(JsonNode json) {
ObjectNode response = Json.newObject();
response.put("correlationId", json.get("correlationId").asText());
response.put("message", "Echo: " + json.get("message").asText());
sender().tell(response, self());
}
}
In this example, each JSON message sent to the actor is expected to have a "correlationId"
and a "message"
field. The actor echoes the message back to the client, preserving the correlation ID, thereby allowing the client to map responses back to their respective requests.
Leveraging Akka Ask Pattern
Another effective strategy is leveraging the Ask pattern in Akka, which is inherently supported by Play Framework. The Ask pattern enables you to send a message to an actor and receive a future response, making it a perfect fit for implementing call-response interactions over WebSockets. Here's how you can utilize it:
import akka.actor.ActorRef;
import akka.actor.ActorSystem;
import akka.pattern.Patterns;
import akka.util.Timeout;
import scala.concurrent.Future;
import java.util.concurrent.CompletionStage;
import java.util.concurrent.TimeUnit;
public class AskPatternExample {
public static CompletionStage<String> askEcho(ActorRef echoActor, String message) {
Timeout timeout = new Timeout(5, TimeUnit.SECONDS);
Future<Object> future = Patterns.ask(echoActor, message, timeout);
return play.libs.akka.AkkaConverters.scalaFutureToJava(future).thenApply(Object::toString);
}
}
In this snippet, the askEcho
method sends a message to an echoActor
and returns a CompletionStage
representing the eventual response. By incorporating the Ask pattern, your application can maintain a clear and concise call-response communication with WebSockets.
Best Practices and Tips
- Efficient ID Generation: When using correlation IDs, ensure they're generated efficiently and are guaranteed to be unique. Options include UUIDs or atomic counters, depending on your specific requirements.
- Timeout Management: Implement timeouts for awaiting responses to prevent memory leaks due to unresolved promises or futures.
- Error Handling: Design your message protocol to include error information, enabling clients to react appropriately to failures or invalid requests.
Final Thoughts
Implementing a call-response pattern in Play Framework WebSockets can be challenging, but with the right approach, you can achieve robust and efficient real-time communication. Utilizing correlation IDs or the Ask pattern provides a structured way to manage interactions between clients and servers, enhancing the overall responsiveness and reliability of your application.
Whether you're building a live chat application, a real-time dashboard, or any other interactive web application, mastering these patterns will significantly improve your ability to provide a seamless user experience. For further reading on WebSocket support in Play Framework, don't hesitate to consult the official documentation.
Remember, the key to successful real-time communication lies in understanding the tools at your disposal and adapting them to fit your application's unique demands. Happy coding!