Unraveling Spring: The Mechanics Behind @Transactional
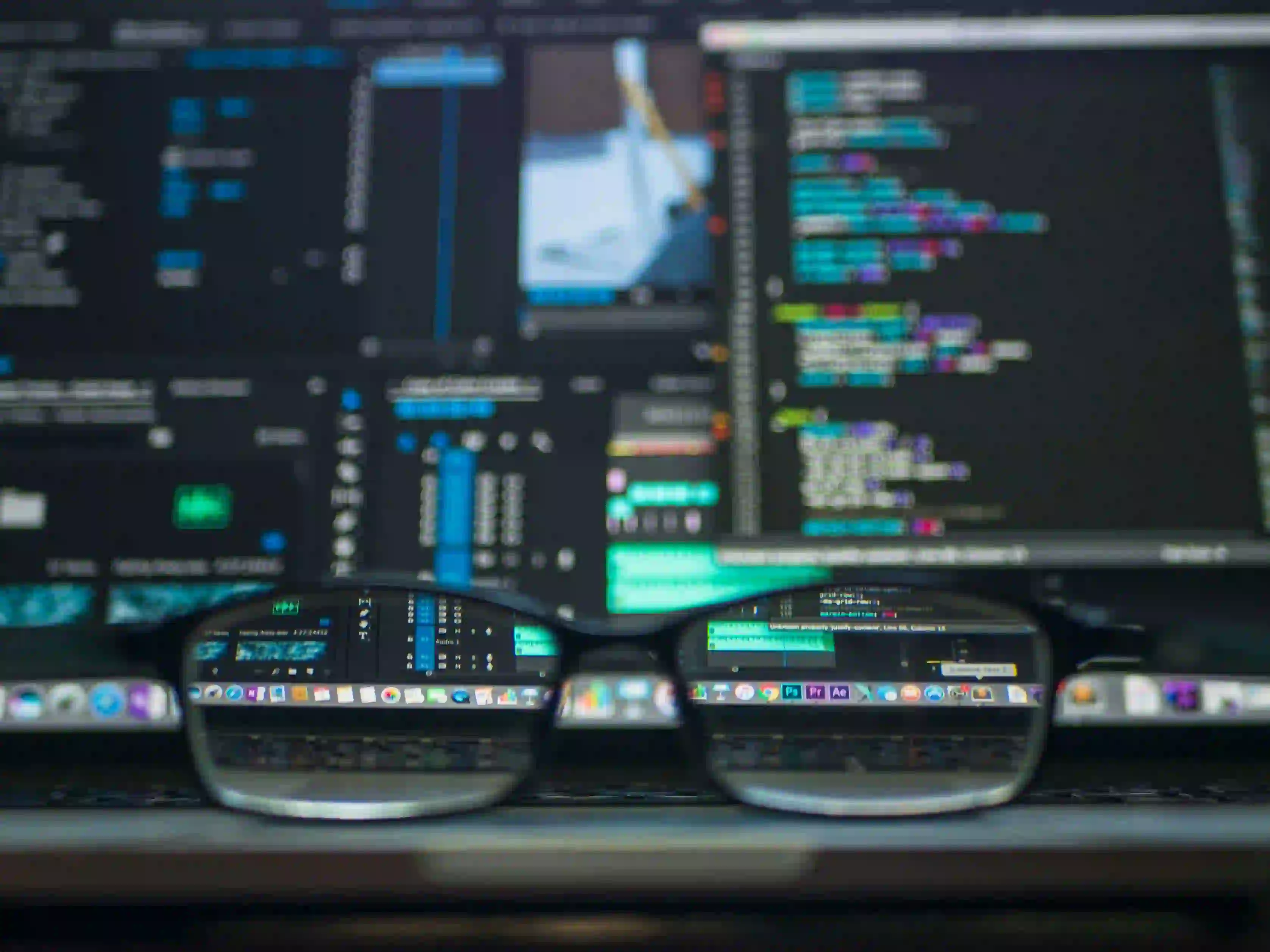
Unraveling Spring: The Mechanics Behind @Transactional
When working with a Java application, particularly in the realm of enterprise-level projects, managing transactions becomes a crucial aspect. The @Transactional
annotation in Spring is a powerful tool that simplifies the management of transactions in our applications. In this blog post, we will delve into the mechanics behind the @Transactional
annotation, understand its working, and explore best practices for its usage.
Understanding Transactions
Before we can fully appreciate the @Transactional
annotation, it's essential to have a solid understanding of transactions.
In the context of a database, a transaction represents a single unit of work. This unit of work is typically a series of database operations that are treated as a single, indivisible operation. The ACID (Atomicity, Consistency, Isolation, Durability) properties govern the behavior of a transaction, ensuring that it is executed reliably and consistently.
In Java applications, Spring Framework provides extensive support for managing transactions, allowing developers to seamlessly integrate transactional behavior into their code.
Introducing @Transactional
The @Transactional
annotation, part of the org.springframework.transaction.annotation
package, marks a method as transactional. When this annotation is applied to a method, Spring ensures that the method is executed within a transactional context.
Let's take a look at a simple example to illustrate the usage of @Transactional
:
import org.springframework.transaction.annotation.Transactional;
@Service
public class OrderService {
@Autowired
private OrderRepository orderRepository;
@Transactional
public void placeOrder(Order order) {
orderRepository.save(order);
// Additional business logic related to order placement
}
}
In this example, the placeOrder
method is annotated with @Transactional
. When this method is invoked, Spring will automatically manage the transaction, committing the changes made to the database if the method executes successfully and rolling back the transaction if an exception occurs.
Behind the Scenes: How @Transactional Works
Under the hood, the @Transactional
annotation utilizes Spring's AOP (Aspect-Oriented Programming) capabilities to intercept the method calls and apply transactional behavior.
When a method annotated with @Transactional
is invoked, Spring dynamically creates a proxy that wraps around the original bean. This proxy intercepts the method calls, evaluates the transactional semantics, and applies the necessary transaction management logic.
Spring's transactional proxies use a combination of JDK dynamic proxies and CGLIB proxies to achieve this behavior. For interfaces, JDK dynamic proxies are employed, while for classes, CGLIB proxies come into play. This allows Spring to cater to a wide range of scenarios, whether the transactional method resides in an interface or a concrete class.
Additionally, the @Transactional
annotation provides various attributes and configurations that allow developers to fine-tune the transactional behavior. Isolation levels, propagation rules, timeout settings, and rollback conditions are just some of the aspects that can be customized to suit the specific requirements of the application.
Best Practices for Using @Transactional
While the @Transactional
annotation simplifies transaction management, it's important to use it judiciously to avoid unintended consequences.
Use @Transactional at the Appropriate Layer
Applying the @Transactional
annotation at the appropriate layer of the application is crucial. Typically, transaction management is handled at the service layer, where business logic resides. Placing @Transactional
directly on the service methods helps to encapsulate the transactional behavior, ensuring that the services operate within a consistent transactional context.
@Service
public class OrderService {
@Autowired
private OrderRepository orderRepository;
@Transactional
public void placeOrder(Order order) {
orderRepository.save(order);
// Additional business logic related to order placement
}
}
Understand Transaction Propagation
The propagation
attribute of the @Transactional
annotation controls how transactions are propagated when a transactional method calls another transactional method. Understanding the different propagation behaviors and choosing the appropriate propagation mode is essential to maintain the integrity of the transactional operations.
Avoid Long-Running Transactions
Long-running transactions can lead to various issues, including resource contention, lock escalation, and decreased throughput. It's important to keep the scope of transactions as narrow as possible and to delegate long-running tasks to asynchronous processing mechanisms if needed.
Handle Transactions Carefully in Concurrent Environments
In a multi-threaded and concurrent application, careful consideration must be given to transaction management to prevent issues such as race conditions, deadlocks, and inconsistent data states. Proper synchronization, isolation levels, and transaction boundaries should be evaluated to ensure the robustness of the transactional operations.
The Closing Argument
The @Transactional
annotation in Spring is a powerful ally in simplifying transaction management within Java applications. By leveraging the mechanics of AOP and proxy-based interception, Spring provides a seamless and flexible approach to handling transactions.
Understanding the intricacies of transaction management, including the appropriate usage of @Transactional
and the best practices for its application, equips developers to design robust and reliable transactional workflows in their applications.
Incorporating the @Transactional
annotation effectively, while adhering to best practices, empowers developers to build scalable, resilient, and maintainable enterprise applications with comprehensive transactional support.
So, the next time you annotate a method with @Transactional
, remember that you're not just marking it for transaction management – you're embracing a robust mechanism that facilitates the cohesive and dependable execution of your business operations.