Avoiding Java Class Stereotypes: A Developer's How-To
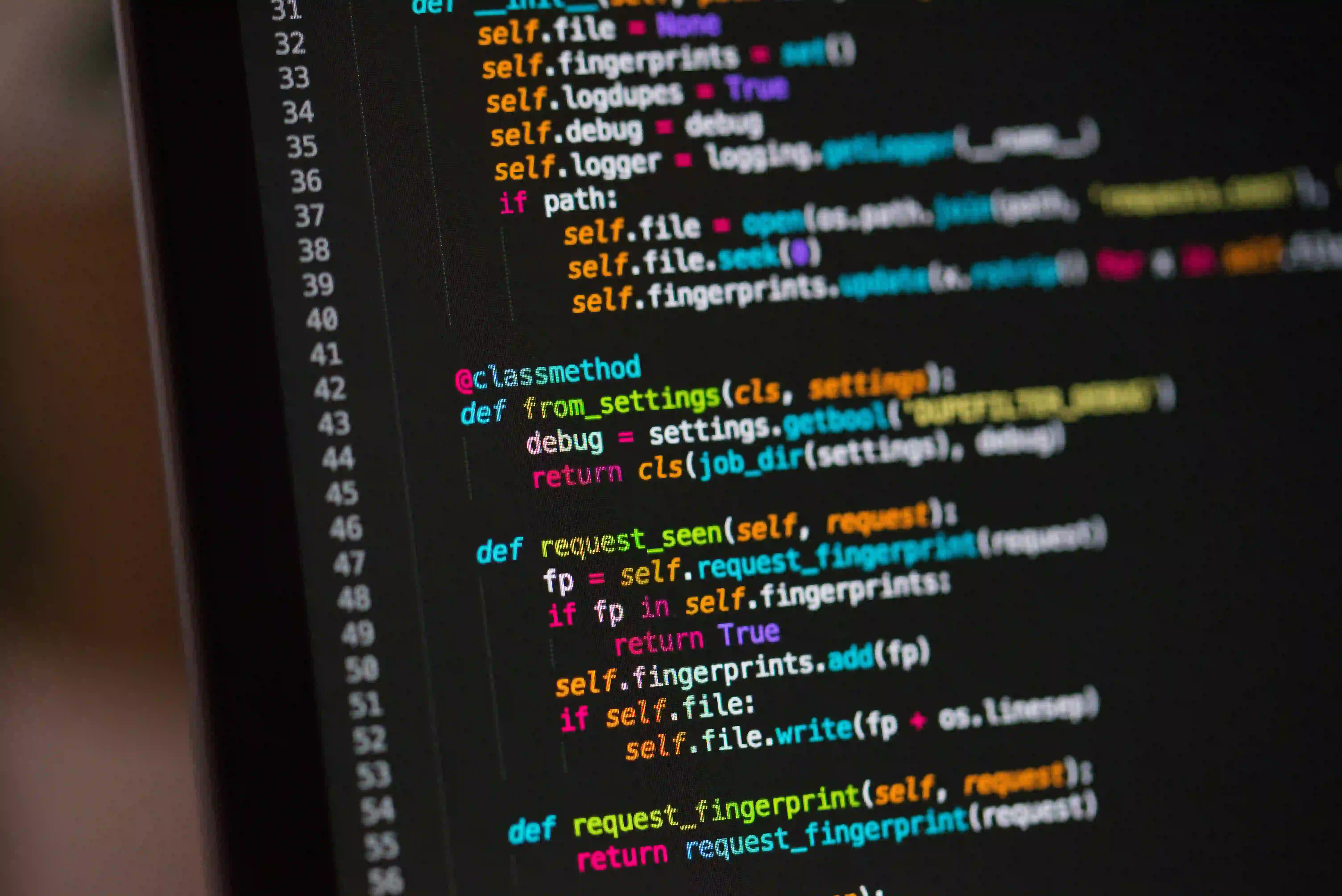
Avoiding Java Class Stereotypes: A Developer's How-To
As a developer, working with Java means working with classes. However, it's easy to fall into certain stereotypes when creating and using classes. In this post, we'll discuss the common stereotypes associated with Java classes and how to avoid them. By breaking these stereotypes, you can write more modular, maintainable, and efficient Java code.
1. Large Monolithic Classes
One of the most common stereotypes in Java development is the creation of large monolithic classes that try to do too much. These classes often end up being difficult to maintain and test. To avoid this stereotype, it's important to embrace the concept of separation of concerns. This principle advocates for breaking down large classes into smaller, specialized classes that each have a single responsibility.
// Example of a large monolithic class
public class MonolithicClass {
private int data;
public void processData() {
// ...code to process data
}
public void generateReport() {
// ...code to generate a report
}
// ...other methods dealing with unrelated tasks
}
In the above example, the MonolithicClass
is responsible for processing data and generating a report, violating the principle of separation of concerns. Let’s refactor this class by separating these responsibilities into distinct classes.
// Example of refactored classes
public class DataProcessor {
public void processData() {
// ...code to process data
}
}
public class ReportGenerator {
public void generateReport() {
// ...code to generate a report
}
}
By splitting the responsibilities into separate classes, we adhere to the principle of separation of concerns, resulting in a more maintainable and testable codebase.
2. Anemic Domain Models
Another stereotype is the creation of anemic domain models, where classes lack behavior and are simply used as data containers. To avoid this, embrace the principles of domain-driven design (DDD) and object-oriented modeling.
// Example of anemic domain model
public class AnemicEmployee {
private String name;
private double salary;
// ...getters and setters
}
In the above example, AnemicEmployee
is merely a container for data without any behavior.
// Example of a domain-driven design approach
public class Employee {
private String name;
private double salary;
public void raiseSalary(double amount) {
this.salary += amount;
}
}
In the refactored Employee
class, behavior related to an employee, such as giving a raise, is encapsulated within the class itself, adhering to the principles of DDD.
3. Overusing Getters and Setters
Java classes often fall into the trap of exposing internal state through excessive getters and setters, leading to bloated interfaces and breaking encapsulation. To avoid this, consider the Tell, Don't Ask principle.
// Example of overusing getters and setters
public class User {
private String username;
private String password;
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
}
In the above example, the User
class exposes its internal state through getters and setters, violating encapsulation.
// Example of Tell, Don't Ask principle
public class User {
private String username;
private String password;
public void authenticate(String username, String password) {
// ...authentication logic
}
}
By using the Tell, Don't Ask principle, the authenticate
method hides the internal state of the User
class and performs the necessary actions, promoting better encapsulation.
4. Utility Classes Galore
Another common stereotype is the proliferation of utility classes that end up being dumping grounds for unrelated methods. To avoid this stereotype, consider using static factory methods and the builder pattern.
// Example of a utility class with unrelated methods
public class StringUtils {
public static boolean isNullOrEmpty(String str) {
return str == null || str.isEmpty();
}
public static String sanitizeString(String str) {
// ...logic to sanitize the string
}
// ...other unrelated methods
}
In the above example, the StringUtils
class contains unrelated methods, violating the principle of cohesion.
// Example of static factory methods and the builder pattern
public class TextUtils {
private String text;
private TextUtils(String text) {
this.text = text;
}
public static TextUtils of(String text) {
// ...logic for creating an instance
return new TextUtils(text);
}
public String sanitize() {
// ...logic to sanitize the text
return text;
}
}
By using static factory methods and the builder pattern, we encapsulate related functionality within the TextUtils
class, promoting better cohesion and maintainability.
The Last Word
In conclusion, by recognizing and avoiding these common stereotypes associated with Java classes, you can write more maintainable, modular, and efficient code. Embracing principles such as separation of concerns, domain-driven design, Tell, Don't Ask, and cohesive design patterns can elevate your Java development skills and lead to cleaner, more maintainable codebases.
So, the next time you find yourself reaching for a monolithic class or anemic domain model, take a step back and consider how to break free from these stereotypes for a more elegant and effective Java code.
Remember, the key to writing exceptional Java code is not just about knowing the language, but also about understanding and applying best practices and principles that transcend stereotypes and lead to better software development. Happy coding!
Feel free to check out Oracle's Java Documentation for a deeper understanding of Java best practices and design principles.