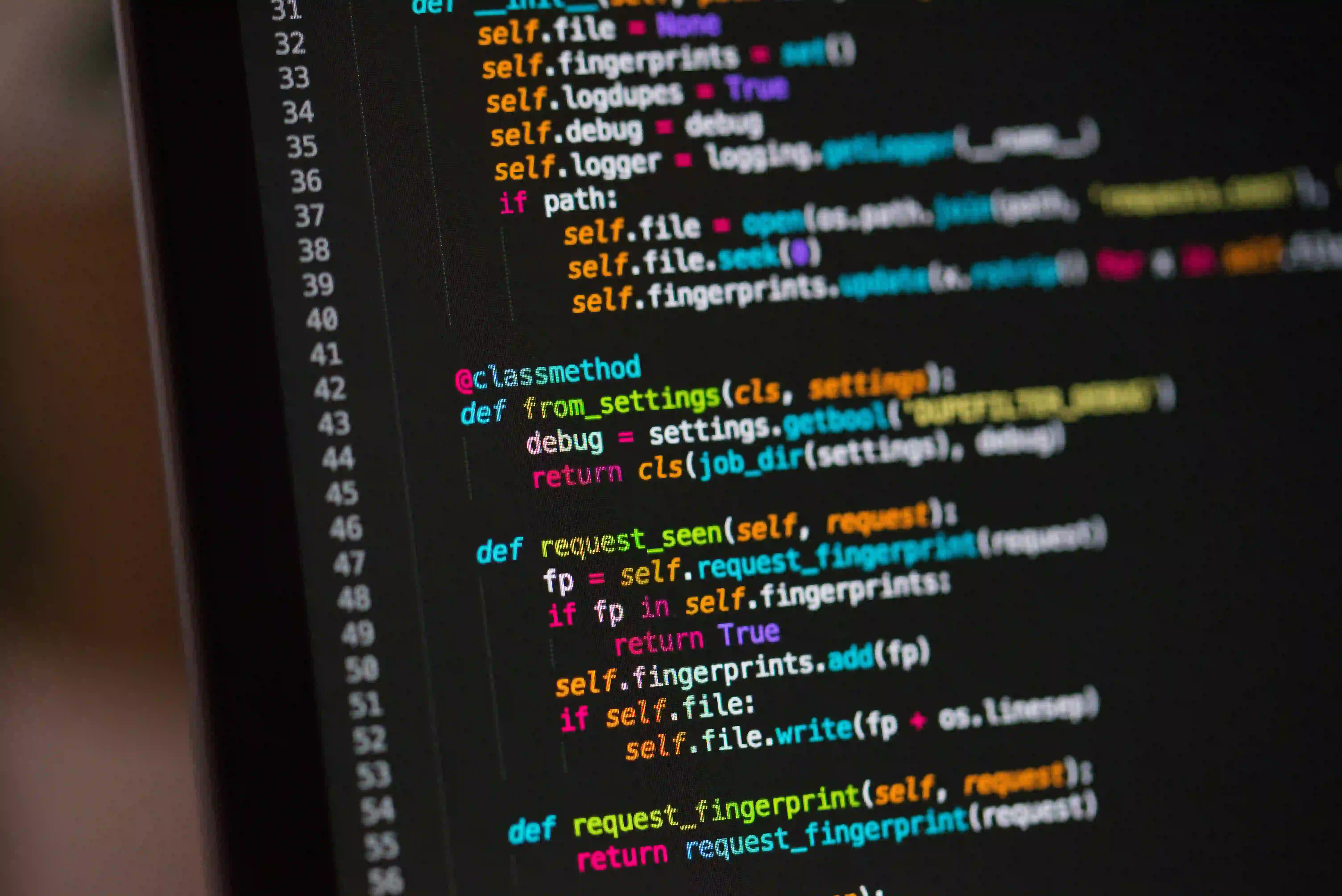
Simplify Logins: Integrating Social Sign-In with Spring MVC
In today's digital age, user authentication plays a pivotal role in the success of web applications. As developers, it is our responsibility to ensure a seamless and secure authentication process for users. One way to simplify the authentication process is by integrating social sign-in functionality into your Java web application. This not only provides a convenient login experience for users but also allows you to leverage the authentication systems of popular social platforms.
In this article, we will explore how to integrate social sign-in with Spring MVC, a widely used Java web framework. We will focus on implementing social sign-in using the popular platforms like Google and Facebook. By the end of this tutorial, you will have a clear understanding of how to integrate social sign-in into your Spring MVC application and simplify the login process for your users.
Setting Up the Project
Before we delve into the integration of social sign-in, let's set up a basic Spring MVC project using Spring Initializr. We will include the necessary dependencies for Spring Web, Thymeleaf, and Spring Social in our project.
Once the project is set up, we can start integrating social sign-in functionality.
Integrating Google Sign-In
Step 1: Configure Google Developer Console
To enable Google Sign-In, you need to create a project in the Google Developer Console and obtain the client ID and client secret. Create OAuth 2.0 credentials for a Web Application and provide the authorized redirect URI, typically http://localhost:8080/google/signin
.
Step 2: Add Spring Social Google
Include the Spring Social Google dependency in your project's pom.xml
:
<dependency>
<groupId>org.springframework.social</groupId>
<artifactId>spring-social-google</artifactId>
<version>1.0.0.RELEASE</version>
</dependency>
Step 3: Configure Spring Social
Configure the Spring Social connection factory for Google in your Spring configuration:
@Configuration
@EnableSocial
public class SocialConfig implements SocialConfigurer {
@Override
public void addConnectionFactories(ConnectionFactoryConfigurer connectionFactoryConfigurer, Environment environment) {
GoogleConnectionFactory googleConnectionFactory = new GoogleConnectionFactory(clientId, clientSecret);
connectionFactoryConfigurer.addConnectionFactory(googleConnectionFactory);
}
// Other configurations
}
Step 4: Implement Google Sign-In in Controller
Now, implement the Google sign-in functionality in your controller:
@Controller
@RequestMapping("/google")
public class GoogleController {
@Autowired
private ConnectionFactoryLocator connectionFactoryLocator;
@Autowired
private UsersConnectionRepository usersConnectionRepository;
@RequestMapping(value = "/signin", method = RequestMethod.GET)
public String signInWithGoogle() {
Connection<?> connection = connectionFactoryLocator.getConnectionFactory(Google.class)
.createConnection(new ServletWebRequest(request));
usersConnectionRepository.createConnectionRepository(connection.getKey()).findPrimaryConnection(Google.class);
return "redirect:/home";
}
// Other methods
}
Integrating Facebook Sign-In
Step 1: Configure Facebook Developer Dashboard
Similar to Google Sign-In, you need to create a project on the Facebook Developer Dashboard to obtain the app ID and app secret. In the app settings, provide the valid OAuth redirect URIs, usually http://localhost:8080/facebook/signin
.
Step 2: Add Spring Social Facebook
Include the Spring Social Facebook dependency in your project's pom.xml
:
<dependency>
<groupId>org.springframework.social</groupId>
<artifactId>spring-social-facebook</artifactId>
<version>3.0.0.RELEASE</version>
</dependency>
Step 3: Configure Spring Social
Configure the Spring Social connection factory for Facebook in your Spring configuration:
@Configuration
@EnableSocial
public class SocialConfig implements SocialConfigurer {
@Override
public void addConnectionFactories(ConnectionFactoryConfigurer connectionFactoryConfigurer, Environment environment) {
FacebookConnectionFactory facebookConnectionFactory = new FacebookConnectionFactory(appId, appSecret);
connectionFactoryConfigurer.addConnectionFactory(facebookConnectionFactory);
}
// Other configurations
}
Step 4: Implement Facebook Sign-In in Controller
Now, implement the Facebook sign-in functionality in your controller:
@Controller
@RequestMapping("/facebook")
public class FacebookController {
@Autowired
private ConnectionFactoryLocator connectionFactoryLocator;
@Autowired
private UsersConnectionRepository usersConnectionRepository;
@RequestMapping(value = "/signin", method = RequestMethod.GET)
public String signInWithFacebook() {
Connection<?> connection = connectionFactoryLocator.getConnectionFactory(Facebook.class)
.createConnection(new ServletWebRequest(request));
usersConnectionRepository.createConnectionRepository(connection.getKey()).findPrimaryConnection(Facebook.class);
return "redirect:/home";
}
// Other methods
}
The Bottom Line
In this tutorial, we learned how to simplify user logins by integrating social sign-in with Spring MVC. We explored the integration of Google and Facebook sign-in functionality, leveraging Spring Social to streamline the process. By implementing social sign-in, you not only provide a convenient authentication method for users but also tap into the vast user base of popular social platforms. Feel free to expand this approach to include other social sign-in options and enrich your application's user experience.
By following these steps, you can simplify logins and elevate the user experience of your Spring MVC web application.