Overcoming Lambda Expression Hurdles in Nashorn Engine
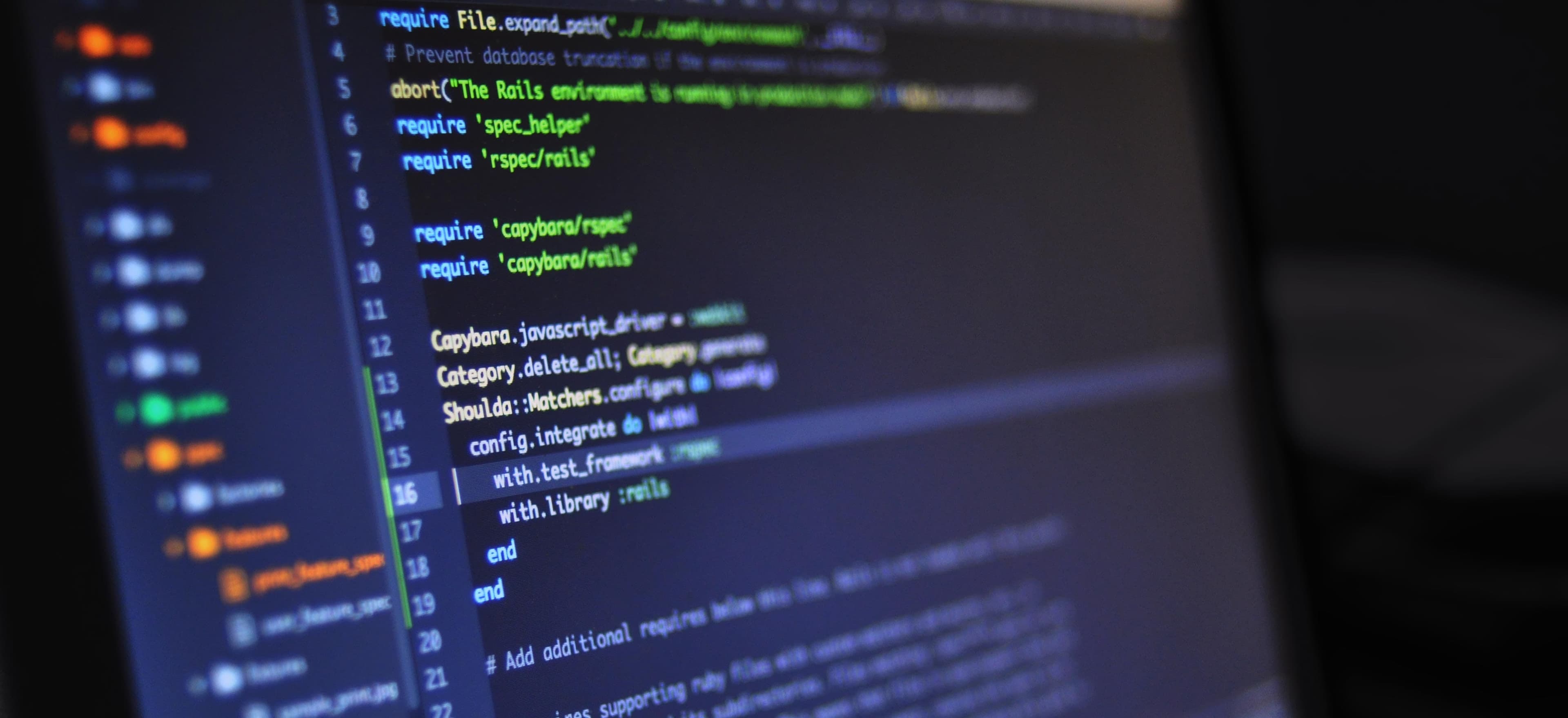
- Published on
Overcoming Lambda Expression Hurdles in Nashorn Engine
In the world of Java, the Nashorn engine has been a game-changer since its introduction in JDK 8. It brought JavaScript compatibility to the table, allowing developers to leverage the power of JavaScript within their Java applications. However, Nashorn's initial release had its limitations, especially when it came to handling lambda expressions. In this article, we will delve into the challenges posed by lambda expressions in Nashorn and explore effective ways to overcome them.
Understanding Lambda Expressions in Java
Lambda expressions are a significant feature introduced in Java 8, enabling developers to write concise and expressive code. They provide a way to represent a piece of code in a more concise manner, often used for implementing functional interfaces. The syntax of a lambda expression consists of parameters, an arrow (->), and a body. For example:
// Lambda expression to double the input value
Function<Integer, Integer> doubleValue = x -> x * 2;
Nashorn Engine and Lambda Expression Limitations
When Nashorn was initially released, it lacked full support for certain features of ECMAScript 6, including proper support for Java 8 lambda expressions. This limitation hindered developers from seamlessly integrating modern JavaScript code that leveraged lambda expressions within their Java applications.
The inability to directly use and manipulate Java functional interfaces with JavaScript code running on Nashorn was a significant obstacle. This made it challenging to reap the full benefits of Java 8 lambdas in a Nashorn environment.
Overcoming the Hurdles
Leveraging the javax.script API
One of the approaches to overcome the limitations of using lambda expressions in Nashorn is to leverage the javax.script
API, which provides a standard interface for embedding scripting engines within Java applications. By using this API, developers can bridge the gap between Java and JavaScript, allowing seamless interaction between the two.
Creating Wrapper Functions
To work around the limitations of Nashorn when it comes to handling lambda expressions, developers can create wrapper functions in JavaScript to encapsulate the behavior of Java functional interfaces. These wrapper functions can then be invoked from the Java code, providing a way to indirectly utilize lambda expressions in a Nashorn environment.
Using Polyglot Programming
With the introduction of GraalVM, a high-performance polyglot virtual machine, developers gained the ability to run multiple languages, including JavaScript and Java, on a single platform with seamless interoperability. By leveraging GraalVM, developers can transcend the limitations of Nashorn and empower their applications to seamlessly work with both Java 8 lambdas and modern JavaScript code.
// Example of invoking JavaScript code from Java using GraalVM
Context context = Context.newBuilder("js").allowAllAccess(true).build();
Value jsFunction = context.eval("js", "function doubleValue(x) { return x * 2; }");
Real-World Application
Imagine a scenario where a Java application requires the execution of a JavaScript callback function that utilizes a lambda expression. By applying the aforementioned approaches, developers can effectively bridge the gap and enable the seamless invocation of JavaScript functions containing lambda expressions from within a Java environment using the Nashorn engine.
The Closing Argument
The integration of modern JavaScript code utilizing lambda expressions within a Java application running on the Nashorn engine was initially a challenging feat. However, with the advent of GraalVM and the creative use of the javax.script
API, developers now have the means to transcend these hurdles and enable seamless interoperability between Java 8 lambdas and JavaScript code.
By understanding the limitations posed by Nashorn and exploring effective strategies to overcome them, developers can harness the full potential of both Java and JavaScript, creating robust and interoperable applications that leverage the power of lambda expressions across both languages.
In conclusion, the Nashorn engine, coupled with the right techniques, opens up a world of possibilities for developers seeking to seamlessly integrate Java and JavaScript, leveraging the strengths of both languages while overcoming any limitations posed by the underlying scripting engine.
For further details on Java lambda expressions, you can refer to the official Oracle documentation. Additionally, the GraalVM documentation offers comprehensive insights into leveraging GraalVM for polyglot programming, further enriching the interplay between Java and JavaScript.