Mastering Moxy's Object Graphs for Dynamic JAXB Magic
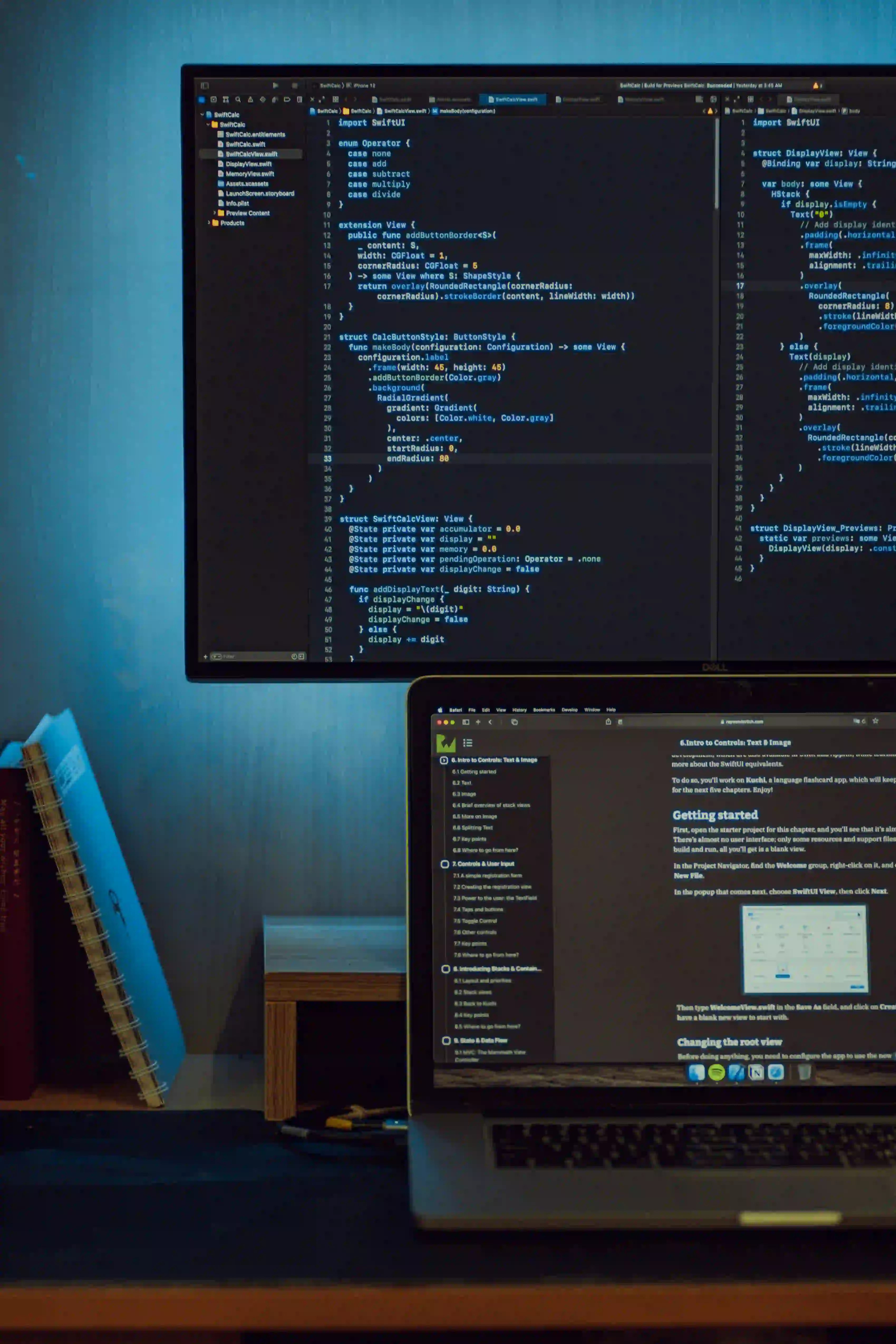
Mastering Moxy's Object Graphs for Dynamic JAXB Magic
When it comes to managing object graphs in Java, Moxy's Object Graphs feature for dynamic JAXB processing offers a powerful and flexible solution. With Moxy's Object Graphs, developers can easily define and manipulate different views of their object models, providing greater control over XML and JSON binding processes. In this article, we'll explore the basics of Moxy's Object Graphs and demonstrate how they can be leveraged to create dynamic and customizable JAXB mappings.
Understanding Moxy's Object Graphs
Moxy's Object Graphs allow developers to define subsets of their object model and specify how those subsets are to be processed during marshalling and unmarshalling. This provides a way to create different views of the same object model, allowing for flexible and dynamic processing of XML and JSON representations.
Defining Object Graphs
Defining an object graph involves identifying a subset of classes and fields within an object model that should be considered as part of the graph. Moxy provides annotations such as @XmlPath
and @XmlNamedObjectGraph
to specify the fields and classes that are included in a particular object graph.
@XmlNamedObjectGraph(
name = "summary",
attributeNodes = {
@XmlNamedAttributeNode("id"),
@XmlNamedAttributeNode("name")
}
)
public class Employee {
private int id;
private String name;
// Getters and setters
}
In the example above, we define an object graph named "summary" for the Employee
class, including only the id
and name
fields. This allows us to create a tailored view of the Employee
object for specific use cases.
Leveraging Object Graphs for Dynamic Mapping
One of the key advantages of Moxy's Object Graphs is their ability to dynamically apply different views of an object model based on runtime conditions. This can be achieved using the Unmarshaller
and Marshaller
interfaces provided by Moxy.
public String marshalEmployeeSummary(Employee employee) {
JAXBContext context = JAXBContext.newInstance(Employee.class);
Marshaller marshaller = context.createMarshaller();
marshaller.setProperty(MarshallerProperties.OBJECT_GRAPH, "summary");
StringWriter writer = new StringWriter();
marshaller.marshal(employee, writer);
return writer.toString();
}
In this example, we create a Marshaller
and specify the "summary" object graph to be used during marshalling. This allows us to dynamically generate a summary view of the Employee
object based on the specific requirements of the application.
Advanced Techniques with Moxy's Object Graphs
Moxy's Object Graphs offer a range of advanced techniques for managing object models in JAXB. Let's explore some of the more advanced features and capabilities provided by Moxy's Object Graphs.
Inheritance and Polymorphism
Moxy's Object Graphs support inheritance and polymorphism, allowing developers to define object graphs that include fields and classes from hierarchies of related types. This provides a powerful way to manage complex object models with varying structures and relationships.
@XmlNamedObjectGraph(
name = "detailed",
attributeNodes = {
@XmlNamedAttributeNode(value = "id", subgraph = "summary"),
@XmlNamedAttributeNode(value = "name", subgraph = "summary"),
@XmlNamedAttributeNode(value = "department", subgraph = "detailed")
}
)
public interface EmployeeDetails extends Employee {
Department getDepartment();
}
In this example, we define an object graph named "detailed" for the EmployeeDetails
interface, including the fields from the "summary" graph as well as the Department
field. This demonstrates how Moxy's Object Graphs can handle inheritance and polymorphic relationships within object models.
Dynamic Graph Resolution
Moxy's Object Graphs also support dynamic resolution, allowing object graphs to be resolved at runtime based on specific conditions or criteria. This provides a way to dynamically change the view of an object model in response to changing requirements or environmental factors.
public String marshalEmployeeGraph(Employee employee, String graphName) {
JAXBContext context = JAXBContext.newInstance(Employee.class);
Marshaller marshaller = context.createMarshaller();
marshaller.setProperty(MarshallerProperties.OBJECT_GRAPH, graphName);
StringWriter writer = new StringWriter();
marshaller.marshal(employee, writer);
return writer.toString();
}
In this example, we create a marshalEmployeeGraph
method that allows specifying the object graph to be used at runtime. This demonstrates how Moxy's Object Graphs can be dynamically resolved based on specific criteria, providing greater flexibility and adaptability in managing object models.
Lessons Learned
Moxy's Object Graphs offer a powerful and flexible solution for managing object models in JAXB, providing the ability to define and manipulate different views of object graphs dynamically. By leveraging Moxy's Object Graphs, developers can create customized mappings for XML and JSON representations, handle complex inheritance and polymorphism, and dynamically resolve object graphs based on specific runtime conditions. This makes Moxy's Object Graphs a valuable tool for achieving dynamic JAXB magic in Java applications.
Incorporating Moxy's Object Graphs into your JAXB project can unlock a new level of flexibility and control in managing complex object models. By mastering Moxy's Object Graphs, you can elevate your JAXB processing to dynamically adapt to diverse and evolving requirements with ease and elegance.
As you delve deeper into the world of dynamic JAXB magic with Moxy's Object Graphs, consider exploring the official EclipseLink Moxy documentation and GitHub repository. These resources provide in-depth insights and additional examples to further expand your expertise and unleash the full potential of Moxy's Object Graphs in Java. Happy coding!