Mastering Java Functions: Common Parameter Pitfalls
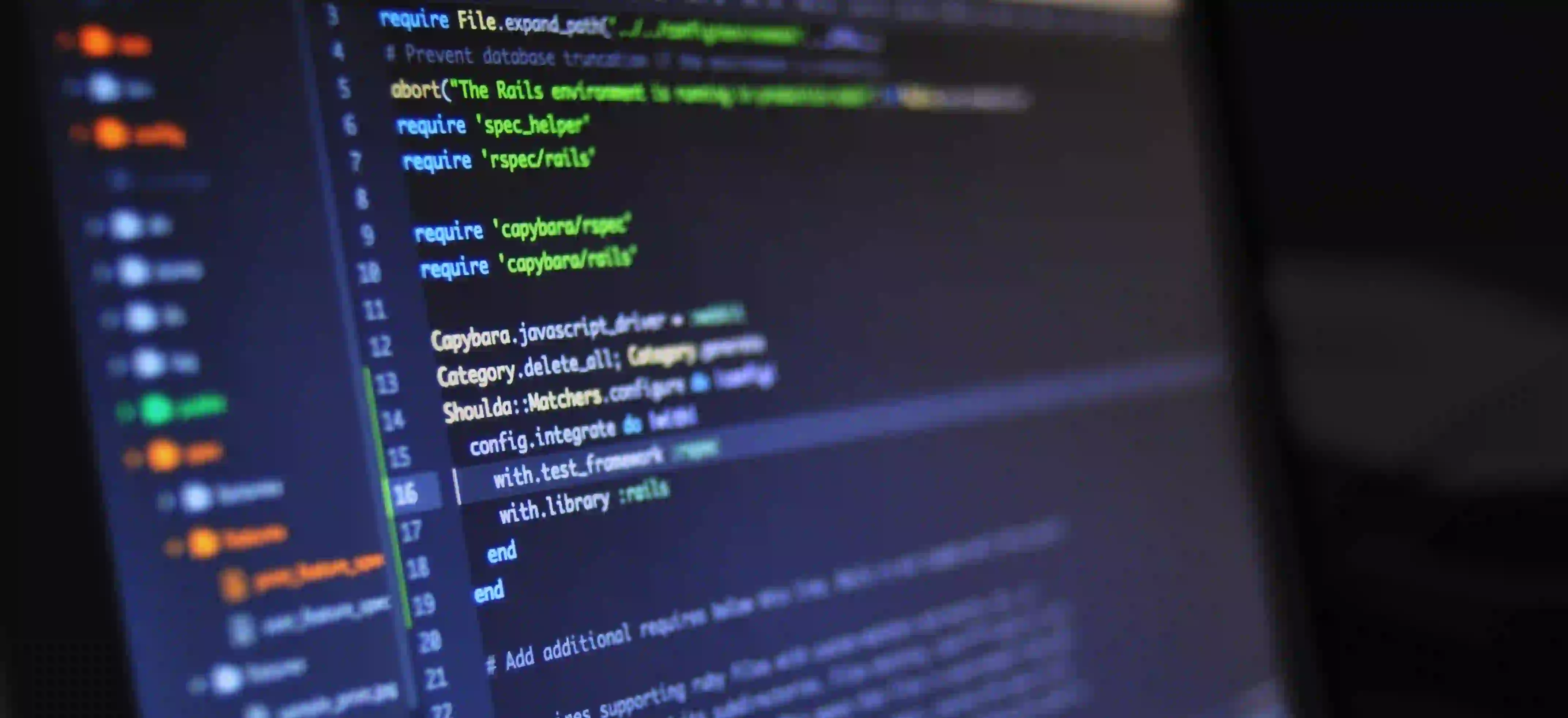
Mastering Java Functions: Common Parameter Pitfalls
Java is a powerful, versatile programming language that can be used for a wide range of applications, from web development to mobile applications. One critical aspect of Java programming that every developer should master is the use of functions (or methods). Functions enable code reusability and modular programming, but they can also lead to complications, particularly when it comes to parameters. In this guide, we will explore common parameter pitfalls in Java, provide code examples, and explain the rationale behind best practices.
Table of Contents
- Understanding Java Functions
- The Importance of Method Parameters
- Common Parameter Pitfalls
- 3.1. Misunderstanding Pass-by-Value
- 3.2. Mutable vs Immutable Parameters
- 3.3. Overusing Varargs
- 3.4. Excessive Parameter Length
- 3.5. Overloading Issues
- Best Practices for Parameter Management
- Conclusion
Understanding Java Functions
Before diving into pitfalls, let’s clarify what functions are in Java. A function (or method) is a block of reusable code that performs a specific task. Functions can accept parameters (inputs) and return a value.
public int add(int a, int b) {
return a + b; // This method takes two integers and returns their sum.
}
In this example, add
is a simple function that takes two parameters, a
and b
, and returns their sum.
The Importance of Method Parameters
Parameters play a crucial role in how functions operate. They define the inputs a function requires to process data effectively. Both the clarity and usability of your APIs rely heavily on how well you design your method parameters.
Common Parameter Pitfalls
3.1. Misunderstanding Pass-by-Value
One of the most common misconceptions among Java developers is how parameters are passed to functions. Java uses pass-by-value, which means that a copy of the variable is passed to the function. This can lead to unexpected results when dealing with object references.
class Person {
String name;
public Person(String name) {
this.name = name;
}
}
public void changeName(Person person) {
person.name = "John"; // Modifying the object through the reference
}
public void reassignName(Person person) {
person = new Person("Doe"); // Reassigning the parameter reference
}
public static void main(String[] args) {
Person person = new Person("Alice");
changeName(person);
System.out.println(person.name); // Output: John
reassignName(person);
System.out.println(person.name); // Output still: John
}
Why: Although the changeName
method alters the internal state of the person
object, the reassignName
method does not change the original reference because it only reassigns the parameter's copy.
3.2. Mutable vs Immutable Parameters
When you pass mutable objects (like arrays or collections) as parameters, modifications in the method affect the original object. It can lead to side effects:
public void modifyList(List<String> list) {
list.add("New Item");
}
public static void main(String[] args) {
List<String> list = new ArrayList<>();
modifyList(list);
System.out.println(list); // Output: [New Item]
}
Why: Here, modifying the list
inside modifyList
impacts the original list declared in main
. To avoid unexpected changes, consider passing immutable objects or create a copy of the mutable object.
3.3. Overusing Varargs
Variadic methods (using varargs
) can simplify method calls but should be used judiciously. Over-using varargs can lead to confusion in method signatures and potential performance degradation:
public void printNumbers(int... numbers) {
for (int number : numbers) {
System.out.println(number);
}
}
public static void main(String[] args) {
printNumbers(1, 2, 3, 4);
}
Why: While this example is simple, excessive reliance on varargs can lead to ambiguous method calls when combined with other parameters. Use them sparingly for clarity, and prefer explicit parameter definitions when possible.
3.4. Excessive Parameter Length
Passing too many parameters to a method can lead to confusion and maintenance difficulties. If a method takes more than three or four parameters, consider if you can encapsulate them into an object:
class User {
String name;
String email;
String address;
// Constructor, getters, and setters omitted for brevity
}
public void createUser(String name, String email, String address) {
// Logic to create user
}
Why: In this case, you are passing three distinct parameters. It might be better to encapsulate them into a User
object to enhance readability and maintainability.
3.5. Overloading Issues
Method overloading allows you to have multiple methods with the same name but different parameter types or counts. However, it can introduce ambiguity:
public void display(String text) {
System.out.println(text);
}
public void display(String text, int count) {
for (int i = 0; i < count; i++) {
System.out.println(text);
}
}
public void display(Object obj) {
System.out.println("Object: " + obj.toString());
}
When you call display(null)
, which method gets executed is ambiguous, leading to potential runtime errors.
Why: This is a classic example of the pitfalls of overloading. Always strive for unambiguous method signatures to ensure that your code behaves as expected.
Best Practices for Parameter Management
-
Limit the Number of Parameters: If your method has too many parameters, consider creating a parameter object.
-
Explicit Parameter Types: Use explicit types and avoid varargs if less ambiguous signatures are available.
-
Use Immutable Types Where Possible: Pass immutable types to avoid accidental modifications.
-
Documentation: Always document your methods clearly, explaining parameter purposes and structures.
-
Validation: Validate parameters within your methods to ensure proper data types and ranges, enhancing robustness.
-
Encapsulation: Group related parameters into objects. This can simplify method signatures and enhance clarity.
Lessons Learned
Parameters are a fundamental aspect of Java functions. While they enhance code reusability and modularity, they also come with challenges. By understanding and avoiding common pitfalls associated with function parameters—such as misunderstanding pass-by-value, dealing with mutable and immutable types, overusing varargs, excessive parameter lengths, and overloading issues—you can write cleaner, more maintainable Java code.
For further reading on Java functions and best practices, check out Java Tutorials or explore effective Java by Joshua Bloch for more in-depth insights.
Mastering Java functions and parameter management can significantly improve your coding efficiency and code quality. Start applying these principles in your projects today!