Boosting Business: Turbocharge Your Client-Server Efficiency
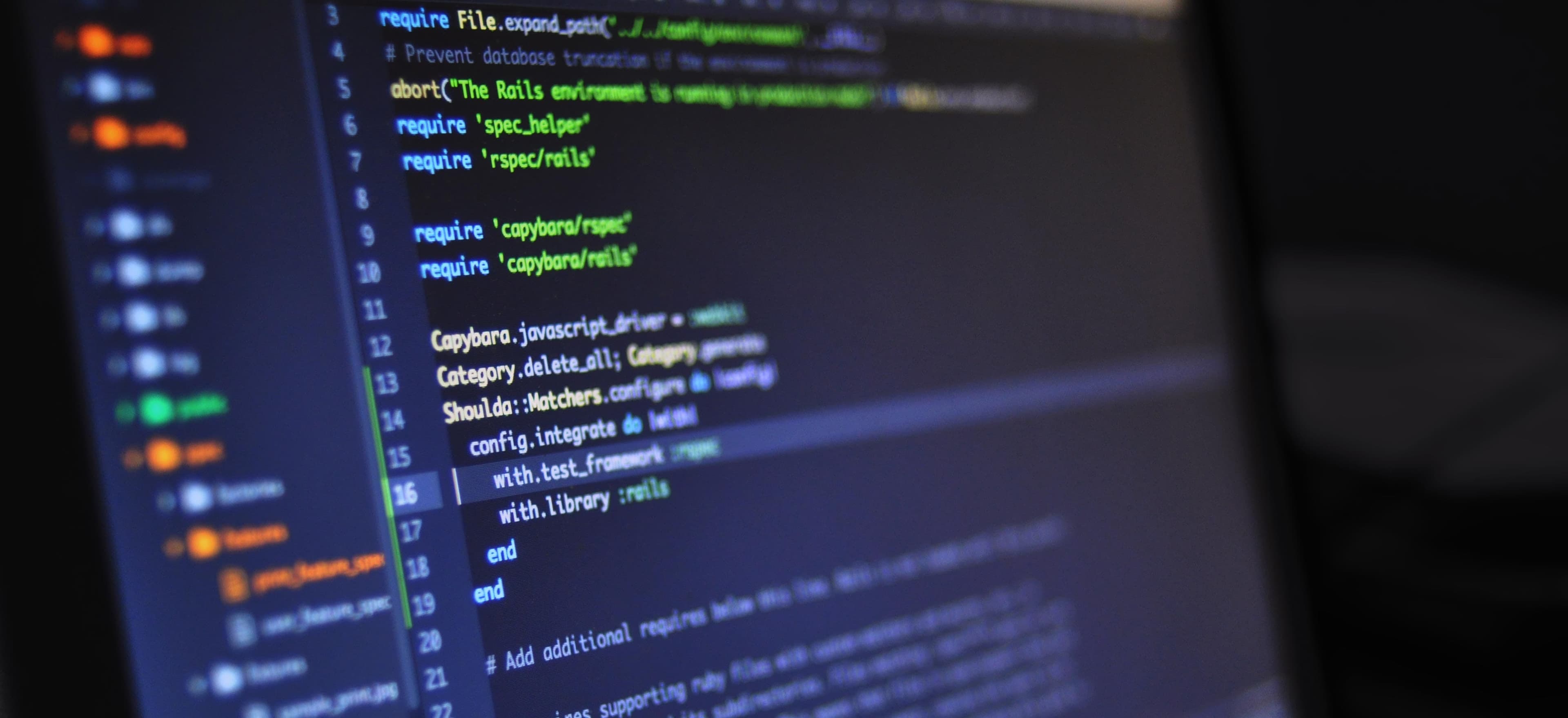
- Published on
Boosting Business: Turbocharge Your Client-Server Efficiency
In the realm of enterprise software development, the client-server architecture stands as a fundamental concept. This architecture defines the relationship between the client, typically a user interface, and the server, which holds the application logic, data, and functions. Java, as a versatile and robust programming language, offers a myriad of tools and libraries to maximize the efficiency of client-server communication. In this blog, we will delve into various strategies and techniques to optimize your Java-based client-server architecture, ultimately boosting your business efficiency.
Understanding the Client-Server Model
Before diving into the optimization techniques, it's crucial to understand the client-server model. In this architecture, the server provides resources and services to multiple clients, which in turn request and consume these resources. The communication between a client and server is typically facilitated through protocols such as HTTP, TCP/IP, or WebSockets.
Leveraging Asynchronous Communication
The Why:
Traditionally, in a synchronous communication model, the client sends a request to the server and waits for a response. This can result in potential inefficiencies, especially in scenarios where the server might take a significant amount of time to process the request. Asynchronous communication allows the client to send a request and continue with other tasks, receiving a response later.
The How:
Java provides robust support for asynchronous communication through libraries like java.nio
and frameworks like Netty. By leveraging asynchronous communication, you can enhance the responsiveness and scalability of your client-server system.
// Example of asynchronous communication using CompletableFuture in Java
CompletableFuture.supplyAsync(() -> performTimeConsumingTask())
.thenAccept(result -> sendResponseToClient(result));
Implementing RESTful Web Services
The Why:
Representational State Transfer (REST) is a popular architectural style for developing web services. It promotes scalability, modifiability, and simplicity. By implementing RESTful services, you can streamline communication between the client and server, leading to improved efficiency and flexibility.
The How:
In Java, you can develop RESTful services using frameworks like Spring Boot, Dropwizard, or JAX-RS. These frameworks offer annotations and conventions that simplify the creation of RESTful endpoints.
// Example of a simple RESTful endpoint using Spring Boot
@RestController
public class UserController {
@GetMapping("/users/{id}")
public User getUserById(@PathVariable Long id) {
// Fetch user from database and return
}
}
Employing Efficient Data Serialization
The Why:
Efficient data serialization is crucial for transmitting data between client and server. Inefficient serialization techniques can lead to increased network overhead and reduced performance. By using efficient serialization methods, you can optimize data transfer and improve overall system efficiency.
The How:
Java provides various serialization options, including JSON (via libraries like Jackson or Gson), XML, and Protocol Buffers. Choose the serialization format based on factors such as data complexity, payload size, and performance requirements.
// Example of JSON serialization using Jackson library
ObjectMapper objectMapper = new ObjectMapper();
String json = objectMapper.writeValueAsString(myObject);
Utilizing Connection Pooling
The Why:
Establishing connections between the client and server can be a resource-intensive process. Connection pooling helps mitigate this overhead by reusing established connections, thereby improving performance and resource utilization.
The How:
Java frameworks such as HikariCP and Apache Commons DBCP offer robust connection pooling capabilities. By configuring and utilizing connection pools, you can significantly reduce the overhead associated with establishing new connections for each client request.
// Example of configuring a connection pool using HikariCP
HikariConfig config = new HikariConfig();
config.setJdbcUrl("jdbc:mysql://localhost:3306/mydb");
config.setUsername("username");
config.setPassword("password");
config.setMaximumPoolSize(10);
DataSource dataSource = new HikariDataSource(config);
Prioritizing Security Measures
The Why:
Security is paramount in client-server communication, especially when dealing with sensitive data. Implementing strong security measures ensures the integrity and confidentiality of the data being transmitted between the client and server, thereby bolstering your business's credibility and trustworthiness.
The How:
Utilize industry-standard security protocols such as HTTPS, TLS, and OAuth for securing communication channels. Additionally, employ robust authentication and authorization mechanisms to control access to server resources.
// Example of configuring HTTPS in Spring Boot application
server.port=8443
server.ssl.key-store=classpath:keystore.jks
server.ssl.key-store-password=yourpassword
server.ssl.key-password=yourpassword
Scaling with Load Balancing
The Why:
As your business grows, handling an increasing number of client requests becomes challenging. Load balancing distributes incoming client requests across multiple server instances, ensuring optimal resource utilization and improved system scalability.
The How:
Adopt load balancing techniques using tools like NGINX, HAProxy, or cloud providers' load balancing services. Incorporate these tools to evenly distribute client requests, prevent server overloads, and enhance the overall efficiency of your client-server architecture.
// Example of configuring NGINX for load balancing
upstream backend {
server backend1.example.com;
server backend2.example.com;
server backend3.example.com;
}
server {
location / {
proxy_pass http://backend;
}
}
Final Considerations
In today's dynamic business landscape, optimizing client-server efficiency is pivotal for delivering seamless user experiences and maintaining a competitive edge. By leveraging the powerful capabilities of Java and adhering to best practices in client-server architecture, you can elevate your business's performance, scalability, and reliability. Implementing asynchronous communication, efficient data serialization, RESTful services, and robust security measures are pivotal steps towards turbocharging your client-server efficiency. Moreover, incorporating load balancing and connection pooling further cements a strong foundation for handling increasing client loads. As you embark on this journey of optimization, remember that continuous refinement and adaptation to evolving technologies will be key to sustaining and enhancing your business's efficiency in the long run.
Optimize your client-server architecture with Java, and watch your business reach new heights of efficiency and scalability.
Incorporate these optimizations into your Java-based client-server architecture and unleash the true potential of your business. Visit Oracle's Java documentation for in-depth insights into Java's networking and concurrency features.
Boost your business by turbocharging your client-server efficiency through Java!
Disclaimer: The code examples provided in this blog are for illustrative purposes and may require additional error handling and validation in production environments.
Checkout our other articles