Overcoming Quarkus Remote Dev Challenges in Docker
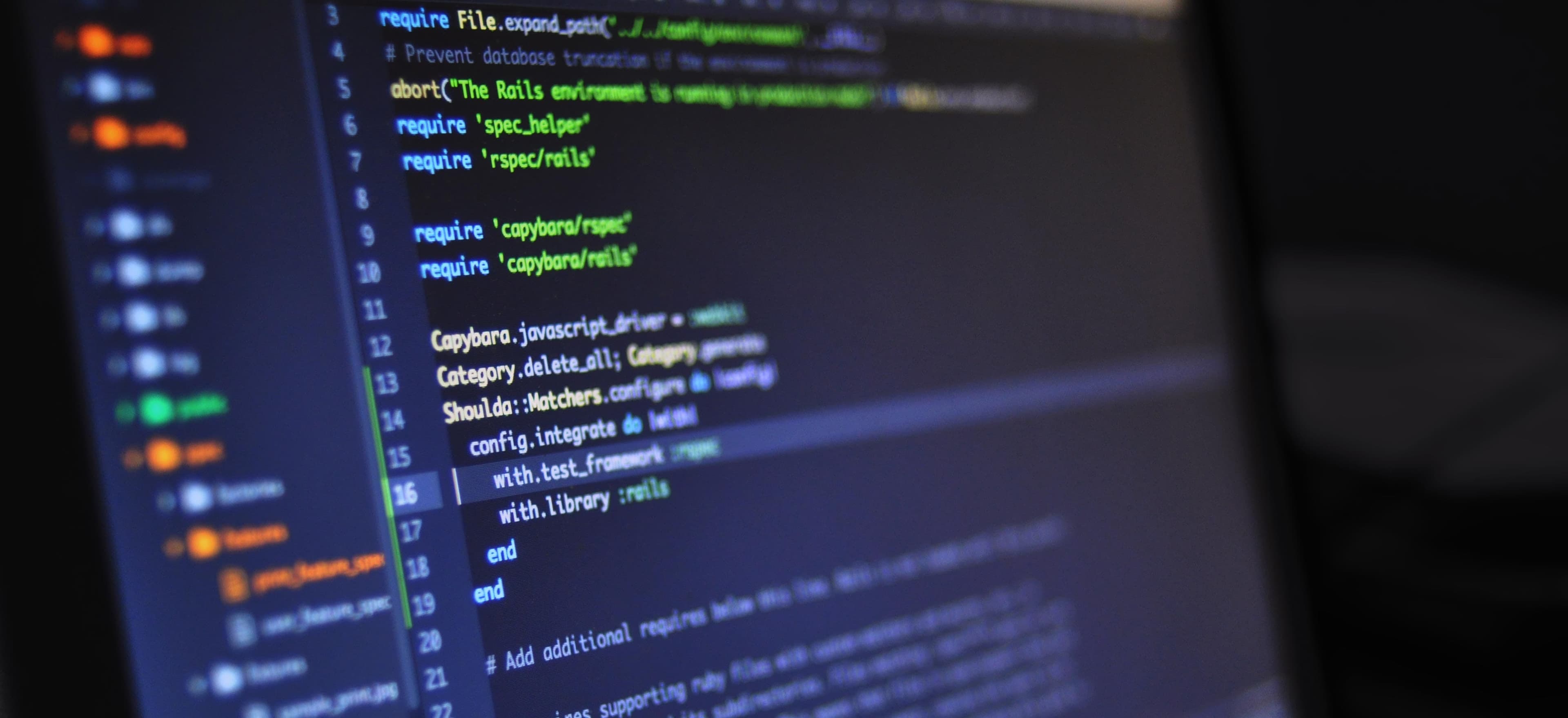
- Published on
Overcoming Quarkus Remote Dev Challenges in Docker
Quarkus is a supremely effective Java framework designed especially for Kubernetes and cloud environments. Its ability to compile Java applications into native executables often results in significantly reduced memory footprints and faster startup times. However, when employing Quarkus in a remote development setup using Docker, developers often encounter unique challenges. In this blog post, we'll explore those challenges and provide solutions to effectively use Quarkus with Docker for a seamless development experience.
Understanding Quarkus and Docker
Before diving into the challenges, it's essential to understand the relationship between Quarkus and Docker. Quarkus streamlines development in containerized environments by:
- Lightweight Architecture: Quarkus is designed from the ground up to work well within containers.
- Hot Reloading: This feature allows developers to see changes in real-time without restarting the application, making development more efficient.
- Native Compilation: The
quarkus build --native
command produces images that are optimized for containerized deployment.
Docker serves as an excellent companion to Quarkus, providing isolation and ease of deployment across various environments. However, remote development can introduce complexities.
Common Challenges
- Network Latency: When using Docker for remote development, network latency can reduce performance, especially when reloading changes.
- Files Not Syncing: Code changes may not automatically reflect in the Docker container, leading to the need for manual reloads.
- Dependency Caching: Caching dependencies in a Docker build can slow down the iterative development process.
- Configuration Complexity: Setting up both Docker and Quarkus might require intricate configuration changes, which can be daunting for beginners.
Solutions to Overcome Challenges
1. Optimizing Network Latency
Network latency issues can be alleviated by using Docker's host networking mode. This allows the container to share the same network interface as the host, reducing round trips.
docker run --network="host" -v ${PWD}:/work -w /work your-quarkus-image
Using --network="host"
will significantly reduce latency, making hot reloads faster. However, this approach works only on Linux hosts, as Docker on Windows and macOS uses a virtual machine.
2. Ensuring Files Sync
To make sure that file changes in your local development environment reflect in the Docker container, you can leverage volume mounting effectively.
docker run -v ${PWD}:/work -w /work your-quarkus-image ./mvnw quarkus:dev
Here, -v ${PWD}:/work
ensures that your working directory is shared with the container. As you save changes, they are instantly available to Quarkus, thanks to live reload features.
3. Managing Dependency Caching
To minimize the time consumed during dependency resolution, you can cache the dependencies outside the context of the application. Here’s how you can structure your Dockerfile to use a multi-stage build.
# First stage: Build the dependencies
FROM maven:3.8.1-openjdk-11 as builder
WORKDIR /app
COPY pom.xml .
COPY src ./src
RUN mvn dependency:go-offline
# Second stage: Build the application
FROM maven:3.8.1-openjdk-11 as final
WORKDIR /app
COPY --from=builder /root/.m2 /root/.m2
COPY . .
RUN mvn clean package -DskipTests
# Final image running stage
FROM openjdk:11-jre
COPY --from=final /app/target/*-runner /application
ENTRYPOINT ["/application"]
In this multi-stage build:
- The dependencies are resolved during the first stage.
- This approach speeds up subsequent builds, especially when you only change application code but not the dependencies.
4. Simplifying Configuration
Creating a docker-compose.yml
can provide a straightforward way to manage containers and configurations. Here is an example:
version: '3.8'
services:
quarkus:
image: your-quarkus-image
build:
context: .
dockerfile: Dockerfile
volumes:
- .:/work
ports:
- "8080:8080"
environment:
- QUARKUS_HTTP_PORT=8080
Using docker-compose up
will bring up your application alongside any supporting services like databases, making it easier to manage your development environment.
Best Practices for Remote Development with Quarkus in Docker
-
Use
.dockerignore
: Create a.dockerignore
file to reduce context size and speed up builds by excluding unnecessary files from Docker context.target/ .git/ .mvn/ README.md
-
Leverage Hot Reload: Always ensure you're running the development mode with live reload enabled (default behavior when using
quarkus:dev
). -
Monitor Resource Usage: Keep an eye on resource consumption. Docker containers can consume considerable CPU and memory, especially when many containers run simultaneously.
-
Regularly Update Your Images: Ensure you use the latest versions of Quarkus and your base Docker images to benefit from performance improvements and new features.
Wrapping Up
Remote development with Quarkus in Docker can significantly streamline workflow efficiencies, provided you overcome the inherent challenges. By optimizing network configurations, setting up proper dependency management, and using volume mounts effectively, a smooth development experience is attainable.
Consider the strategies and code snippets discussed in this blog post as foundational tools in your toolkit. Feel empowered to experiment and adapt them to your circumstances. As Quarkus evolves, do not hesitate to explore the official documentation for the latest best practices.
For more insights and discussions on Java development, stay tuned to our blog. Happy coding!
Checkout our other articles