Upgrade Your Java Skills: Tackle 2023 with The Latest Tips!
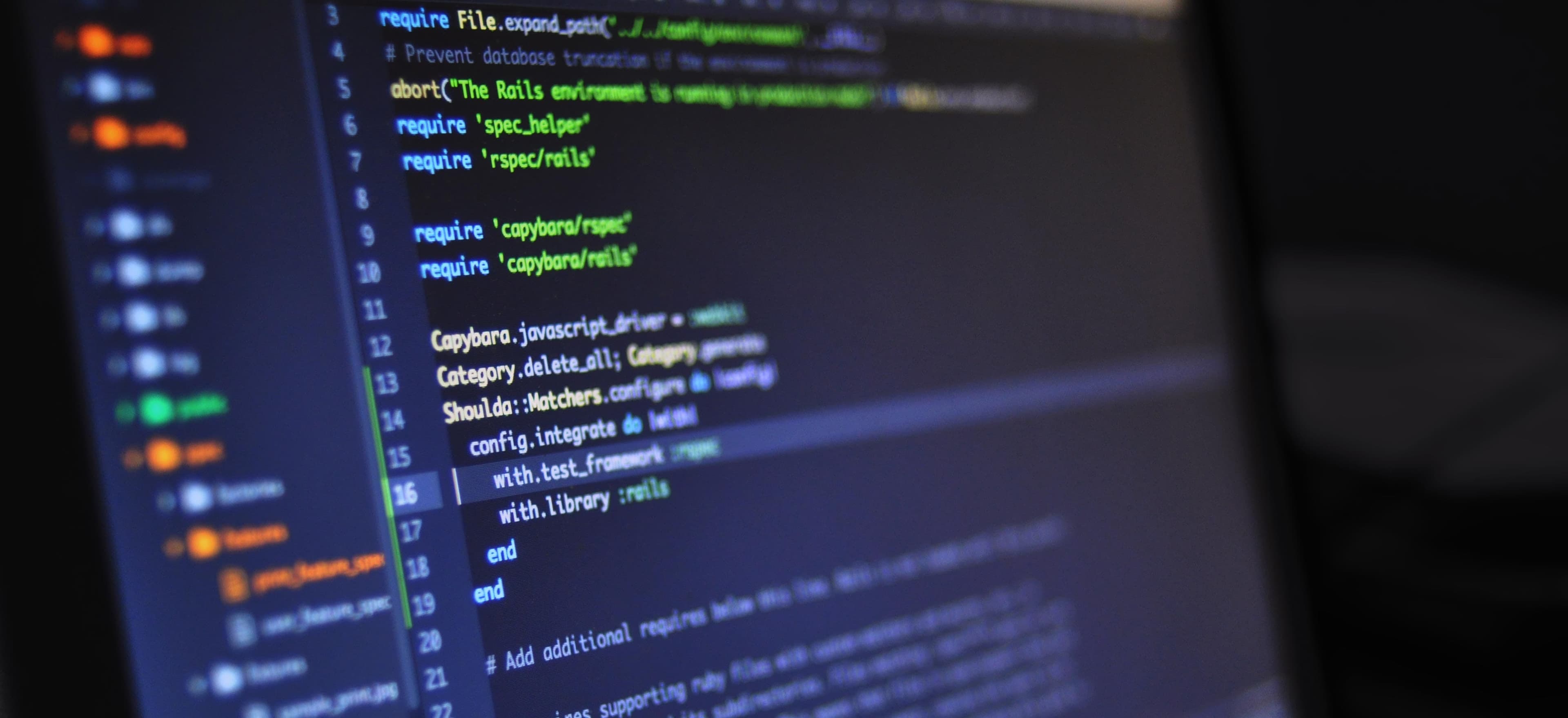
- Published on
Mastering Java in 2023: The Ultimate Guide for Developers
As we step into 2023, it’s crucial for Java developers to stay at the top of their game. With its strong object-oriented foundation, portability, and robust libraries, Java continues to be a dominant force in the programming world. However, to truly excel in this language, developers must keep up with the latest trends and best practices. In this comprehensive guide, we will explore some of the advanced tips and techniques that will help you elevate your Java skills in 2023.
Embracing Modern Java Features
Java has come a long way from its early versions, and the modern iterations offer a plethora of features that can significantly enhance your coding experience. From the enhanced switch statement in Java 12 to the introduction of sealed classes and interfaces in Java 15, staying abreast of these updates will not only improve your code but also make it more concise and readable.
Utilize Records for Concise Data Classes
One of the most exciting additions to Java is the introduction of records in Java 16. Records provide a compact method for declaring classes that are solely meant for storing data.
public record Point(int x, int y) { }
With just a single line of code, you can define a data class with immutable properties, automatically generating the constructor, accessors, and equals()
, hashCode()
, and toString()
methods. This not only reduces boilerplate code but also enhances code readability.
Sealed Classes and Interfaces for Improved Security
Java 17 introduced sealed classes and interfaces, allowing developers to restrict which other classes or interfaces may extend or implement them. By explicitly stating the permitted subclasses or implementing classes, you can enforce stronger design constraints, ensuring that your codebase remains cohesive and secure.
Enhancing Performance with Project Loom
In the ever-evolving landscape of software development, handling concurrency is a critical aspect. Traditionally, working with threads in Java has been complex and resource-intensive. However, Project Loom, expected to be fully integrated in Java 18, aims to revolutionize concurrent programming in Java with the introduction of lightweight, user-mode threads known as fibers.
Simplifying Concurrency with Fibers
Fibers, also referred to as virtual threads, provide a more efficient and scalable approach to concurrency by reducing the overhead associated with traditional threads. They offer a simpler and more straightforward concurrency model, allowing developers to handle a large number of concurrent tasks with minimal resources.
Fiber<Void> fiber = Fiber.schedule(() -> {
// Asynchronous task
});
By leveraging Project Loom, developers can expect drastic improvements in the performance and scalability of their applications, making it a pivotal advancement to watch out for in 2023.
Leveraging Functional Programming Paradigms
The rise of functional programming has greatly influenced Java, leading to the integration of several features that promote a more functional style of coding. Learning how to harness these paradigms can significantly enhance your ability to write concise, maintainable, and robust code.
Embrace Immutability with Local-Variable Type Inference
Local-Variable Type Inference, introduced in Java 10, enables developers to declare local variables without explicitly stating their type. By doing so, you can write more concise and readable code while maintaining strong type safety.
var list = new ArrayList<String>();
This feature encourages the use of immutable collections, fostering a more functional approach to working with data.
Streamlining Code with Optional and CompletableFuture
The Optional class, added in Java 8, promotes the avoidance of null values and encourages the use of explicit handling for scenarios where a value may be absent. Similarly, CompletableFuture, introduced in the same version, facilitates asynchronous programming, enabling developers to compose and combine asynchronous operations effortlessly.
Optional<String> name = Optional.ofNullable(getName());
name.ifPresent(System.out::println);
CompletableFuture<Integer> futureResult = CompletableFuture.supplyAsync(() -> performTimeConsumingTask());
By integrating these functional programming constructs into your code, you can write more robust and predictable logic that aligns with modern best practices.
Embracing Containerization with Java
In the era of microservices and cloud-native applications, containerization has become a ubiquitous trend. Java developers can leverage containerization technologies to build, deploy, and manage their applications more efficiently.
Simplify Deployment with Docker
Docker has emerged as a standard for containerization, providing a consistent environment for applications to run across different environments. By containerizing your Java applications, you can streamline the deployment process and ensure that your code operates consistently, regardless of the underlying infrastructure.
Harness Kubernetes for Orchestration
Kubernetes, a powerful container orchestration system, offers sophisticated capabilities for deploying, managing, and scaling containerized applications. Java developers can utilize Kubernetes to automate the deployment and scaling of their applications, ensuring seamless operation in dynamic and resource-constrained environments.
Closing Remarks
As we navigate through 2023, honing your Java skills by embracing modern features, enhancing performance with Project Loom, leveraging functional paradigms, and embracing containerization will set you on the path to success. By staying abreast of the latest advancements and best practices in the Java ecosystem, you can elevate your coding prowess and deliver more efficient, resilient, and scalable solutions.
In conclusion, Java remains a powerhouse in the realm of software development, and with the right skills and knowledge, you can continue to wield its capabilities to build groundbreaking applications that meet the demands of the future. So, gear up, stay informed, and dive into the immersive world of Java development in 2023!
Checkout our other articles